如何在 JavaScript 中獲取輸入值
Kirill Ibrahim
2023年10月12日
JavaScript
JavaScript Input
-
使用
document.getElementById(id_name)
來獲取 JavaScript 中的輸入值 -
在 JavaScript 中使用
document.getElementsByClassName('class_name')
來獲取輸入值 -
使用
document.querySelector('selector')
來獲取 JavaScript 中的輸入值
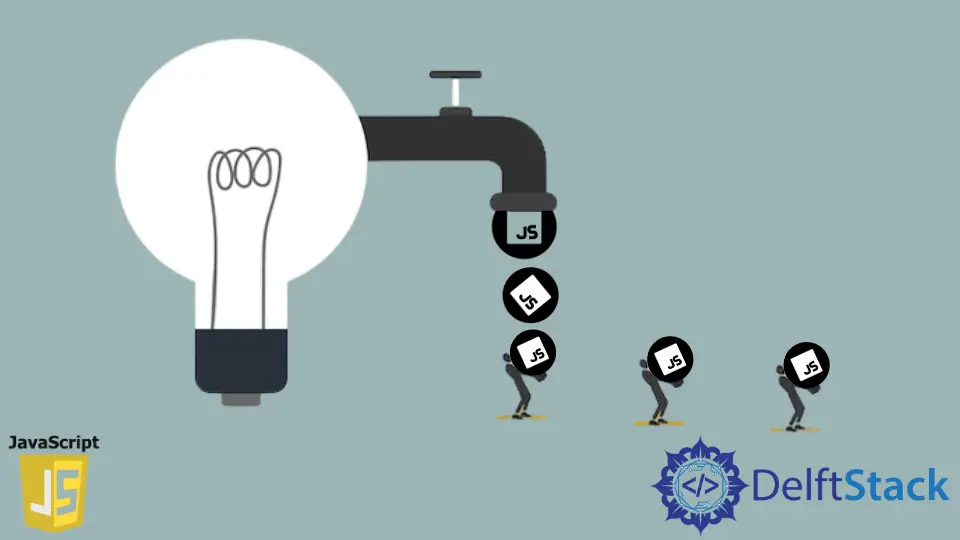
在 JavaScript 中,我們可以通過選擇 DOM 輸入元素並使用 value
屬性來獲取輸入值,而不需要將其包裹在表單元素中。
JavaScript 有不同的方法來選擇 DOM 輸入元素。下面的每個方法都有一個程式碼示例,你可以在你的機器上執行。
使用 document.getElementById(id_name)
來獲取 JavaScript 中的輸入值
我們給 DOM 輸入元素賦予 id
屬性,然後用 document.getElementById(id_name)
來選擇 DOM 輸入元素,你可以直接使用 value
屬性。
例子
<!DOCTYPE html>
<html>
<body>
<h4>
Change the text of the text field
,and then click the button.
</h4>
<label for="domTextElement">Name: </label>
<input type="text" id="domTextElement" >
<button type="button" onclick="getValueInput()">
click me!!
</button>
<p id="valueInput"></p>
<script>
const getValueInput = () =>{
let inputValue = document.getElementById("domTextElement").value;
document.getElementById("valueInput").innerHTML = inputValue;
}
</script>
</body>
</html>
在 JavaScript 中使用 document.getElementsByClassName('class_name')
來獲取輸入值
我們給 DOM 輸入元素賦予 class 屬性,然後用 document.getElementsByClassName('class_name')
來選擇 DOM 輸入元素,但是如果我們有不同的 DOM 輸入元素有相同的 class 名稱,那麼它將返回一個 DOM 輸入陣列,所以我們應該通過給出索引號來指定選擇哪一個:document.getElementsByClassName('class_name')[index_number].value
。
例子
<!DOCTYPE html>
<html>
<body>
<h4>
Change the text of the text field
,and then click the button.
</h4>
<input type="text" class="domTextElement" >
<label for="domTextElement">Name: </label>
<input type="text" class="domTextElement" >
<button type="button" onclick="getValueInput()">
click me!!
</button>
<p id="valueInput"></p>
<script>
const getValueInput = () =>{
let inputValue = document.getElementsByClassName("domTextElement")[1].value;
document.getElementById("valueInput").innerHTML = inputValue;
}
</script>
</body>
</html>
使用 document.querySelector('selector')
來獲取 JavaScript 中的輸入值
document.querySelector('selector')
使用 CSS
選擇器,也就是說,它可以通過 DOM 元素的 id、class、tag 名和 name 屬性來選擇元素。
例子: document.querySelector('selector')
document.querySelector('class_name')
document.querySelector('id_name')
document.querySelector('input') // tag name
document.querySelector('[name="domTextElement"]') // name property
例子:
<!DOCTYPE html>
<html>
<body>
<h4>
Change the text of the text field
,and then click the button.
</h4>
<input type="text" id="domTextElement1" >
<label for="domTextElement">Name: </label>
<input type="text" class="domTextElement2" >
<button type="button" onclick="getValueInput()">
click me!!
</button>
<p id="valueInput"></p>
<script>
const getValueInput = () =>{
let inputValue1 = document.querySelector("#domTextElement1").value;
let inputValue2 = document.querySelector(".domTextElement2").value;
document.querySelector("#valueInput").innerHTML = `First input value: ${inputValue1} Second Input Value: ${inputValue2}`;
}
</script>
</body>
</html>
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe