Ottieni valore di input in JavaScript
-
Usa
document.getElementById(id_name)
per ottenere il valore di input in JavaScript -
Usa
document.getElementsByClassName('class_name')
per ottenere il valore di input in JavaScript -
Usa
document.querySelector('selector')
per ottenere il valore di input in JavaScript
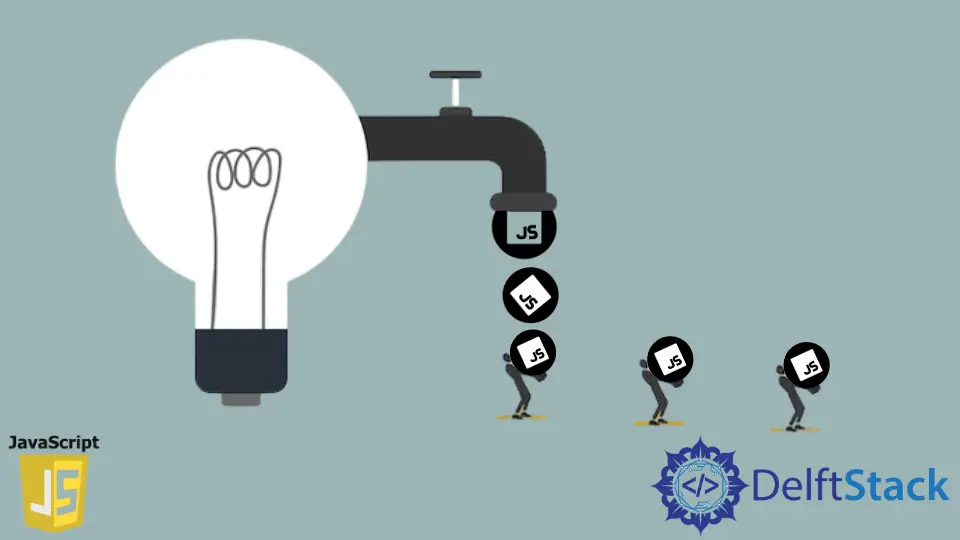
Possiamo ottenere il valore di input senza avvolgerlo all’interno di un elemento del form in JavaScript selezionando l’elemento di input DOM e utilizzando la proprietà value
.
JavaScript ha diversi metodi per selezionare l’elemento di input DOM. Ogni metodo seguente avrà un esempio di codice, che puoi eseguire sulla tua macchina.
Usa document.getElementById(id_name)
per ottenere il valore di input in JavaScript
Diamo la proprietà id
al nostro elemento di input Dom, e quindi usiamo document.getElementById(id_name)
per selezionare l’elemento di input DOM, puoi semplicemente usare la proprietà value
.
Esempio:
<!DOCTYPE html>
<html>
<body>
<h4>
Change the text of the text field
,and then click the button.
</h4>
<label for="domTextElement">Name: </label>
<input type="text" id="domTextElement" >
<button type="button" onclick="getValueInput()">
click me!!
</button>
<p id="valueInput"></p>
<script>
const getValueInput = () =>{
let inputValue = document.getElementById("domTextElement").value;
document.getElementById("valueInput").innerHTML = inputValue;
}
</script>
</body>
</html>
Usa document.getElementsByClassName('class_name')
per ottenere il valore di input in JavaScript
Diamo la proprietà class al nostro elemento di input Dom, quindi usiamo document.getElementsByClassName('class_name')
per selezionare l’elemento di input DOM, ma se abbiamo diversi elementi di input Dom con lo stesso nome di classe, allora restituirà un array di input Dom, quindi dovremmo specificare quale selezioneremo fornendo il numero di indice: document.getElementsByClassName('class_name')[index_number].value
.
Esempio:
<!DOCTYPE html>
<html>
<body>
<h4>
Change the text of the text field
,and then click the button.
</h4>
<input type="text" class="domTextElement" >
<label for="domTextElement">Name: </label>
<input type="text" class="domTextElement" >
<button type="button" onclick="getValueInput()">
click me!!
</button>
<p id="valueInput"></p>
<script>
const getValueInput = () =>{
let inputValue = document.getElementsByClassName("domTextElement")[1].value;
document.getElementById("valueInput").innerHTML = inputValue;
}
</script>
</body>
</html>
Usa document.querySelector('selector')
per ottenere il valore di input in JavaScript
Il document.querySelector('selector')
usa i selettori CSS
, il che significa che può selezionare gli elementi per id, classe, nome del tag e proprietà name dell’elemento DOM.
Esempio:
document.querySelector('class_name')
document.querySelector('id_name')
document.querySelector('input') // tag name
document.querySelector('[name="domTextElement"]') // name property
Esempio:
<!DOCTYPE html>
<html>
<body>
<h4>
Change the text of the text field
,and then click the button.
</h4>
<input type="text" id="domTextElement1" >
<label for="domTextElement">Name: </label>
<input type="text" class="domTextElement2" >
<button type="button" onclick="getValueInput()">
click me!!
</button>
<p id="valueInput"></p>
<script>
const getValueInput = () =>{
let inputValue1 = document.querySelector("#domTextElement1").value;
let inputValue2 = document.querySelector(".domTextElement2").value;
document.querySelector("#valueInput").innerHTML = `First input value: ${inputValue1} Second Input Value: ${inputValue2}`;
}
</script>
</body>
</html>