JavaScript에서 입력 값 가져 오기
Kirill Ibrahim
2023년10월12일
JavaScript
JavaScript Input
-
document.getElementById(id_name)
를 사용하여 JavaScript에서 입력 값 가져 오기 -
document.getElementsByClassName('class_name')
을 사용하여 JavaScript에서 입력 값 가져 오기 -
document.querySelector('selector')
를 사용하여 JavaScript에서 입력 값 가져 오기
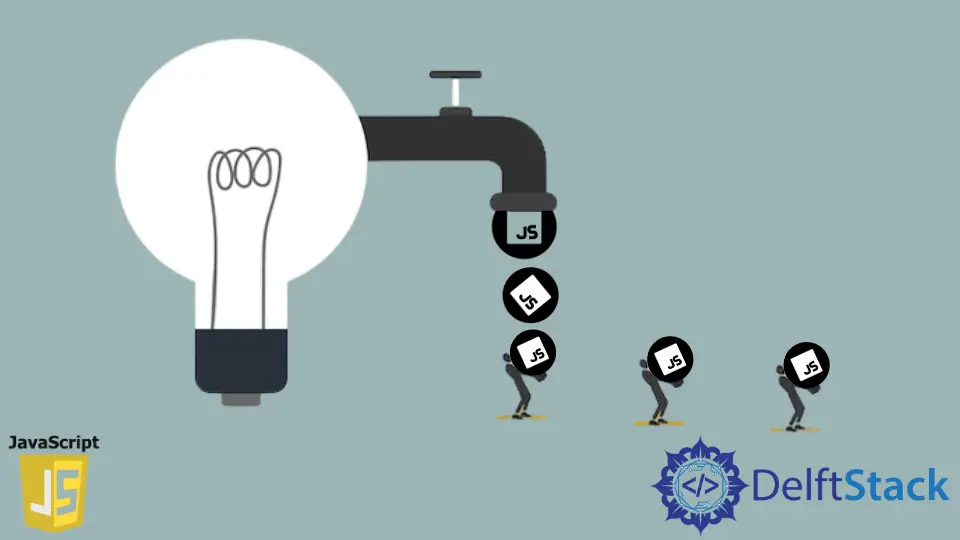
DOM 입력 요소를 선택하고value
속성을 사용하여 JavaScript의 양식 요소 안에 넣지 않고 입력 값을 가져올 수 있습니다.
JavaScript에는 DOM 입력 요소를 선택하는 다른 방법이 있습니다. 아래의 모든 메서드에는 컴퓨터에서 실행할 수있는 코드 예제가 있습니다.
document.getElementById(id_name)
를 사용하여 JavaScript에서 입력 값 가져 오기
Dom 입력 요소에id
속성을 부여한 다음document.getElementById(id_name)
를 사용하여 DOM 입력 요소를 선택합니다. 간단히value
속성을 사용하면됩니다.
예:
<!DOCTYPE html>
<html>
<body>
<h4>
Change the text of the text field
,and then click the button.
</h4>
<label for="domTextElement">Name: </label>
<input type="text" id="domTextElement" >
<button type="button" onclick="getValueInput()">
click me!!
</button>
<p id="valueInput"></p>
<script>
const getValueInput = () =>{
let inputValue = document.getElementById("domTextElement").value;
document.getElementById("valueInput").innerHTML = inputValue;
}
</script>
</body>
</html>
document.getElementsByClassName('class_name')
을 사용하여 JavaScript에서 입력 값 가져 오기
Dom 입력 요소에 클래스 속성을 부여한 다음document.getElementsByClassName('class_name')
을 사용하여 DOM 입력 요소를 선택하지만 동일한 클래스 이름을 가진 다른 Dom 입력 요소가있는 경우 배열을 반환합니다. Dom 입력의 개수이므로 색인 번호document.getElementsByClassName('class_name')[index_number].value
를 제공하여 선택할 항목을 지정해야합니다.
예:
<!DOCTYPE html>
<html>
<body>
<h4>
Change the text of the text field
,and then click the button.
</h4>
<input type="text" class="domTextElement" >
<label for="domTextElement">Name: </label>
<input type="text" class="domTextElement" >
<button type="button" onclick="getValueInput()">
click me!!
</button>
<p id="valueInput"></p>
<script>
const getValueInput = () =>{
let inputValue = document.getElementsByClassName("domTextElement")[1].value;
document.getElementById("valueInput").innerHTML = inputValue;
}
</script>
</body>
</html>
document.querySelector('selector')
를 사용하여 JavaScript에서 입력 값 가져 오기
document.querySelector('selector')
는CSS
선택자를 사용합니다. 즉, DOM 요소의 ID, 클래스, 태그 이름, 이름 속성별로 요소를 선택할 수 있습니다.
예:
document.querySelector('class_name')
document.querySelector('id_name')
document.querySelector('input') // tag name
document.querySelector('[name="domTextElement"]') // name property
예:
<!DOCTYPE html>
<html>
<body>
<h4>
Change the text of the text field
,and then click the button.
</h4>
<input type="text" id="domTextElement1" >
<label for="domTextElement">Name: </label>
<input type="text" class="domTextElement2" >
<button type="button" onclick="getValueInput()">
click me!!
</button>
<p id="valueInput"></p>
<script>
const getValueInput = () =>{
let inputValue1 = document.querySelector("#domTextElement1").value;
let inputValue2 = document.querySelector(".domTextElement2").value;
document.querySelector("#valueInput").innerHTML = `First input value: ${inputValue1} Second Input Value: ${inputValue2}`;
}
</script>
</body>
</html>
튜토리얼이 마음에 드시나요? DelftStack을 구독하세요 YouTube에서 저희가 더 많은 고품질 비디오 가이드를 제작할 수 있도록 지원해주세요. 구독하다