如何在 JavaScript 中获取输入值
Kirill Ibrahim
2023年10月12日
JavaScript
JavaScript Input
-
使用
document.getElementById(id_name)
来获取 JavaScript 中的输入值 -
在 JavaScript 中使用
document.getElementsByClassName('class_name')
来获取输入值 -
使用
document.querySelector('selector')
来获取 JavaScript 中的输入值
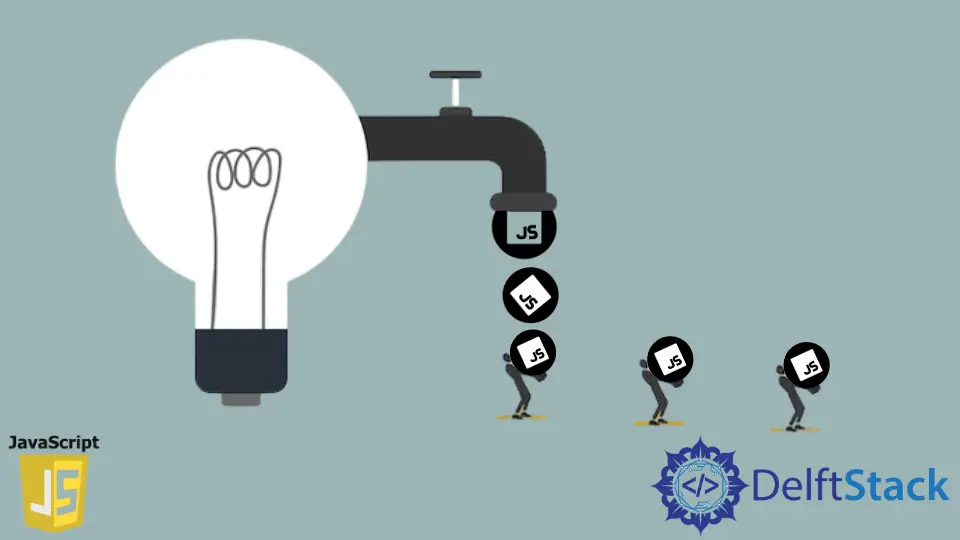
在 JavaScript 中,我们可以通过选择 DOM 输入元素并使用 value
属性来获取输入值,而不需要将其包裹在表单元素中。
JavaScript 有不同的方法来选择 DOM 输入元素。下面的每个方法都有一个代码示例,你可以在你的机器上运行。
使用 document.getElementById(id_name)
来获取 JavaScript 中的输入值
我们给 DOM 输入元素赋予 id
属性,然后用 document.getElementById(id_name)
来选择 DOM 输入元素,你可以直接使用 value
属性。
例子
<!DOCTYPE html>
<html>
<body>
<h4>
Change the text of the text field
,and then click the button.
</h4>
<label for="domTextElement">Name: </label>
<input type="text" id="domTextElement" >
<button type="button" onclick="getValueInput()">
click me!!
</button>
<p id="valueInput"></p>
<script>
const getValueInput = () =>{
let inputValue = document.getElementById("domTextElement").value;
document.getElementById("valueInput").innerHTML = inputValue;
}
</script>
</body>
</html>
在 JavaScript 中使用 document.getElementsByClassName('class_name')
来获取输入值
我们给 DOM 输入元素赋予 class 属性,然后用 document.getElementsByClassName('class_name')
来选择 DOM 输入元素,但是如果我们有不同的 DOM 输入元素有相同的 class 名称,那么它将返回一个 DOM 输入数组,所以我们应该通过给出索引号来指定选择哪一个:document.getElementsByClassName('class_name')[index_number].value
。
例子
<!DOCTYPE html>
<html>
<body>
<h4>
Change the text of the text field
,and then click the button.
</h4>
<input type="text" class="domTextElement" >
<label for="domTextElement">Name: </label>
<input type="text" class="domTextElement" >
<button type="button" onclick="getValueInput()">
click me!!
</button>
<p id="valueInput"></p>
<script>
const getValueInput = () =>{
let inputValue = document.getElementsByClassName("domTextElement")[1].value;
document.getElementById("valueInput").innerHTML = inputValue;
}
</script>
</body>
</html>
使用 document.querySelector('selector')
来获取 JavaScript 中的输入值
document.querySelector('selector')
使用 CSS
选择器,也就是说,它可以通过 DOM 元素的 id、class、tag 名和 name 属性来选择元素。
例子: document.querySelector('selector')
document.querySelector('class_name')
document.querySelector('id_name')
document.querySelector('input') // tag name
document.querySelector('[name="domTextElement"]') // name property
例子:
<!DOCTYPE html>
<html>
<body>
<h4>
Change the text of the text field
,and then click the button.
</h4>
<input type="text" id="domTextElement1" >
<label for="domTextElement">Name: </label>
<input type="text" class="domTextElement2" >
<button type="button" onclick="getValueInput()">
click me!!
</button>
<p id="valueInput"></p>
<script>
const getValueInput = () =>{
let inputValue1 = document.querySelector("#domTextElement1").value;
let inputValue2 = document.querySelector(".domTextElement2").value;
document.querySelector("#valueInput").innerHTML = `First input value: ${inputValue1} Second Input Value: ${inputValue2}`;
}
</script>
</body>
</html>
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe