How to Clear Input in JavaScript
-
Use the
onfocus
Attribute to Vanish Input Field in JavaScript - Use Conditional Statement to Clear Form Input Value in JavaScript
-
Use the
reset()
Method to Erase Form Input Fields in JavaScript
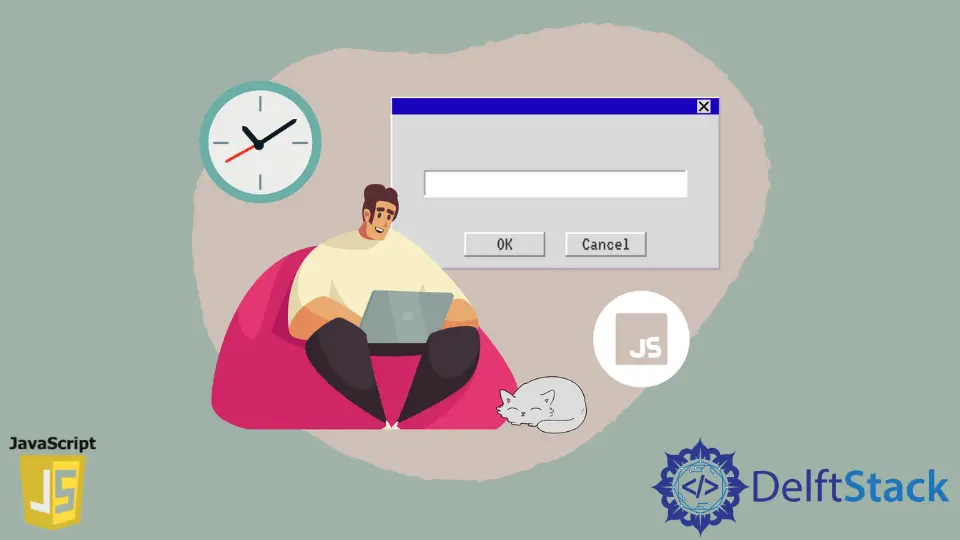
In JavaScript, we usually consider a specific property to deal with the input value. The value
property along with the getElementById()
method grabs an input field’s detail.
Later, we can perform some conditions based on the entry. Again, we can also depend on some HTML attributes to connect the input elements and JavaScript functions.
Here, we will present some examples that can be implemented in multiple ways.
Use the onfocus
Attribute to Vanish Input Field in JavaScript
We will initially declare an input field with a defined value for this example. Also, the input element will jump to perform the function clearF(this)
as soon the cursor will point or focus in the input box.
If the function’s condition is served, it will remove the value, and thus the input gets cleared.
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>test</title>
</head>
<body>
<input type="text" value= "abc@gmail.com" onfocus="clearF(this)">
<script>
function clearF(target) {
if (target.value == 'abc@gmail.com') {
target.value = "";
}
}
</script>
</body>
</html>
Output:
Its content vanishes as the cursor is placed and clicked on the input field. The this
refers to its value for the specific element.
Use Conditional Statement to Clear Form Input Value in JavaScript
According to a form submission, it is necessary to have a corresponding button element to store data. We will take a form
element, and the button
associated with it will carry an onclick
attribute to fire a function.
The function will check if the input field is empty or not. If it finds any content, it will simply reinitialize it empty.
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>test</title>
</head>
<body>
<form>
<input type="text" id="name">
<input type="button" value="clear" onclick="clearF()">
</form>
<script>
function clearF() {
var grab = document.getElementById("name");
if (grab.value !="") {
grab.value = "";
}
}
</script>
</body>
</html>
Output:
Here, we have an instance of the grab
form that will take the id
. When the button is clicked, the function will check if the input is empty, and it will perform just as the conditional statement describes.
Use the reset()
Method to Erase Form Input Fields in JavaScript
We will also examine a form to clear its input by the reset()
method. All it will need is to create an instance of the form, and on the button click, it will reset the whole form vanishing the inserted contents.
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>test</title>
</head>
<body>
<form id="Form">
Name: <input type="text"><br><br>
<input type="button" onclick="myFunction()" value="Reset form">
</form>
<script>
function myFunction() {
document.getElementById("Form").reset();
}
</script>
</body>
</html>
Output: