How to Use Event Target in JavaScript
- Event Target in JavaScript
-
Use
this
Keyword to Grab the Target Object in JavaScript -
Use
event.target
to Grab the Target Object in JavaScript -
Use
event.currentTarget
to Fetch the Root Element in JavaScript
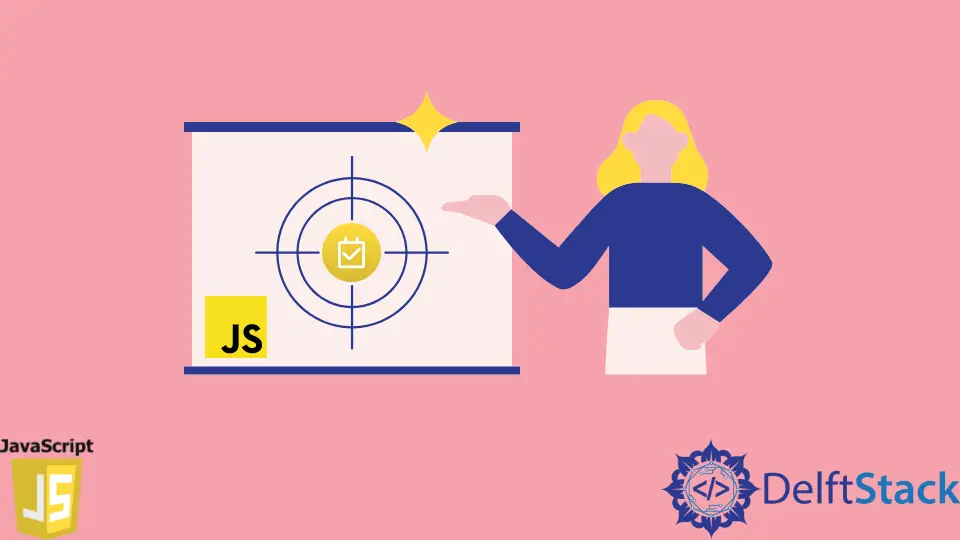
In this article, we will learn the event.target
property in JavaScript source code and the benefit of that property in the JavaScript webpage.
Event Target in JavaScript
The event.target
property helps find the name of the HTML element used to trigger the event. For example, if we call a function from an HTML element click event, we can determine the specified element name to trigger and call a function.
To get that property, we call event.target
and store it in a variable like let variable = event.target
. Now, we can get multiple event.target
properties with that variable.
It returns a reference to the object on which the event occurred. With the help of the target
property of the event, we can do the following tasks.
- We can get the
element
that triggers the event. - We can get access to the
element
properties. - We can easily modify some properties of the
element
.
Modern web browsers fully support this target
event property.
Basic Syntax:
var property = event.target;
var elementName = property.tagName
In the following example, we will use the event.target
object and tagName
property together to determine the element name used to trigger an event on click and call the declared function.
Example:
<!DOCTYPE html>
<html>
<body onclick="myFunction(event)">
<h1 style="color:blueviolet">DelftStack Learning</h1>
<h3>JavaScript Target event property</h3>
<p>Click on button on this web page in this document to find out the element name which is used to triggered the event.</p>
<button>Click here</button>
<p id="myPara"></p>
<script>
function myFunction(event) {
var myVariable = event.target; // get tagert event property
document.getElementById("myPara").innerHTML = "Event triggered by a " + myVariable.tagName + " element";
}
</script>
</body>
</html>
In the above HTML web page source, we have used the HTML element button to trigger an event and call myFunction
. In that function, we have to get an event as an argument, use the variable myVariable
, and store property using the event.
Then, we simply used the default document.getElementById('myPara').innerHTML
to assign the string text to the paragraph element. We have already created the paragraph element in the body and assigned the id myPara
.
We have concatenated the tag name with myVariable.tagName
to display the element name in the paragraph. You can save the above source in the HTML extension, open it in the browser, and see the result.
Use this
Keyword to Grab the Target Object in JavaScript
We will set a div
having a p
, strong
, and a span
tag. And we will apply the click
event on the document.body
.
We will see that the event only returns the object or HTML element referred to via the this
keyword. Let’s check the output.
Code Snippet:
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>event.target demo</title>
<style>
span, strong, p {
padding: 10px;
display: block;
border: 1px solid green;
}
</style>
<script src="https://code.jquery.com/jquery-3.5.0.js"></script>
</head>
<body>
<div id="log"></div>
<div>
<p>
<strong><span>HIT</span></strong>
</p>
</div>
<script>
document.body.addEventListener('click', function(event){
document.getElementById('log').innerHTML = 'clicked: ' + this.nodeName;
});
</script>
</body>
</html>
Output:
So, the click
event exclusively returns the object body
as the event was dispatched initially by the body
element. The p
tag and all other descendants are also under the body
element, yet the this
keyword only focuses on the root object of initiation.
In the next section, we will see how the same code results differ for event.target
.
Use event.target
to Grab the Target Object in JavaScript
We will examine the same instance from before. In this case, we will change the innerHTML
part and switch this
with the event.target
.
Let’s visualize first before explaining.
Code Snippet:
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>event.target demo</title>
<style>
span, strong, p {
padding: 10px;
display: block;
border: 1px solid blue;
}
</style>
<script src="https://code.jquery.com/jquery-3.5.0.js"></script>
</head>
<body>
<div id="log"></div>
<div>
<p>
<strong><span>HIT</span></strong>
</p>
</div>
<script>
document.body.addEventListener('click', function(event){
document.getElementById('log').innerHTML = 'clicked: ' + event.target.nodeName;
});
</script>
</body>
</html>
Output:
Here, the event.target
fetched the whole data structure under the body
element. And after the initiation of the click
event, the reference is pointed to the latest element, which is the span
.
Later on, clicking on the other sections, we could retrieve the strong
, p
, and body
accordingly. This is the basic difference between the this
keyword and event.target
.
Use event.currentTarget
to Fetch the Root Element in JavaScript
The event.currentTarget
is another way of performing the task of the this
keyword. But in operation with this
, we only get the parent elements node name.
Here, in the case of the event.currentTarget
, we will also get the body
as a return value, but the initial pointing would be at the span
, and in the return value, it will only present the last stoppage (bottom to top).
It follows the event bubble phase that enables to return root element of an HTML data structure. Let’s hop into the code fence.
Code Snippet:
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>event.target demo</title>
<style>
span, strong, p {
padding: 10px;
display: block;
border: 1px solid red;
}
</style>
<script src="https://code.jquery.com/jquery-3.5.0.js"></script>
</head>
<body>
<div id="log"></div>
<div>
<p>
<strong><span>HIT</span></strong>
</p>
</div>
<script>
document.body.addEventListener('click', function(event){
document.getElementById('log').innerHTML = 'clicked: ' + event.currentTarget.nodeName;
});
</script>
</body>
</html>
Output: