Difference Between Event Bubbling and Capturing in JavaScript
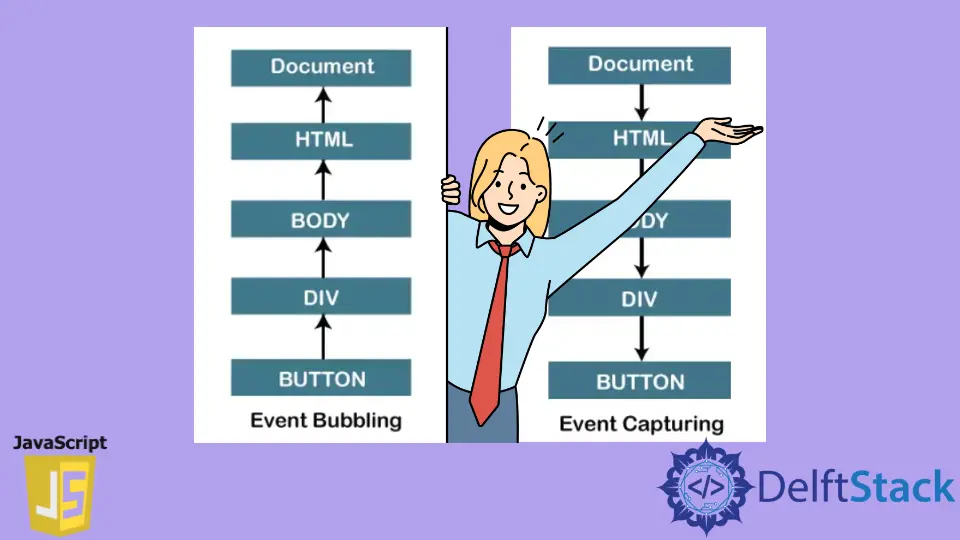
This article will talk about event bubbling and event capturing used in JavaScript. After reading this article, you will clearly understand event bubbling and capturing in JavaScript.
We will also discuss the differences between these events. We will see when to use the event bubbling and capturing in JavaScript.
In JavaScript, event bubbling and capturing describe phases in how the browser handles events targeted at nested elements.
Let’s understand these two events one by one.
Event Bubbling in JavaScript
The event bubbling is a method of event propagation used in JavaScript. Event bubbling is a process that starts with the element that triggers the event and bubbles up the containing elements in order.
Using the event bubbling in JavaScript, the event is first captured and handled by the innermost element and then propagated to the outermost elements.
The principle of the event bubbling is very simple. When an event happens on another element, it first runs on the handlers and then on its parent element.
It relates to the order in which events are propagated in nested elements in JavaScript.
Let’s understand the event bubbling with the help of the below chart.
The above image shows that when a user clicks on the button, the event flows in this order from bottom to top.
Now let’s understand the event bubbling with the help of example code.
As we can see, we have an HTML code in which we created two buttons, parent
and child
. We stored the parent
button in <div>
and the child
button in <p>
.
In the code below, we used JavaScript, and in the script, we used a .addEventListener
object to perform the bubbling event.
We will have two events shown when we run this code: parent
and child
. The child
event is the innermost element, and the parent
event is the outermost element.
Example code:
<!DOCTYPE html>
<html>
<head>
<title>
The Bubbling_Event in Javascript
</title>
</head>
<body>
<h2>The Bubbling_Event in Javascript</h2>
<div id="parent">
<button>
<h2>Parent</h2>
</button>
<button id="child">
<p>Child</p>
</button>
</div><br>
<script>
document.getElementById(
"child").addEventListener("click", function () {
alert("YOU CLICKED THE CHILD ELEMENT.");
}, false);
document.getElementById(
"parent").addEventListener("click", function () {
alert("YOU CLICKED THE PARENT ELEMENT.");
}, false);
</script>
</body>
</html>
The output after running the code:
When we click on the parent
button, we get the following output:
When we click on the child
button, we get the following output:
From the above example code, we see that in the event bubbling, the innermost element’s event is triggered first and then follows the outermost event’s element. The <p>
element is handled first, then the <div>
.
So, we use the bubbling event when we need to handle the innermost element in JavaScript.
Access the demo of the above example code here.
Event Capturing in JavaScript
The event capturing is rarely used in real code but sometimes can be very helpful. The event capturing is the opposite of the event bubbling; the outermost element is targeted first in the event capturing.
In JavaScript, we use event capturing when we need to handle the outermost element’s event first. The event capturing is performed before the event bubbling, but it is rarely used because the event bubbling is sufficient to handle the flow of the events in JavaScript.
The flow chart to understand the event capturing is given below. The image shows that when a user clicks the button, the event flows in this order, from top to bottom.
Now, let’s understand the event capturing with the help of an example code.
As we can see in the following code, we have created a button in the <div>
element. We also used JavaScript in the following HTML code in which we used the .addEventListener
object, which performs the event capturing.
When we run this code and click on the Click Me
button, the console will generate the following output.
Example code:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content=
"width=device-width, initial-scale=1.0">
<title>What is Event Capturing in JavaScript</title>
</head>
<body>
<div class="button-list">
<div class="button-container">
<button class="button"
onClick="console.log('Button!')">
Click Me
</button>
</div>
</div>
<script>
document.addEventListener("click", () =>
console.log('Event Capturing!'), true);
document.addEventListener("click", () =>
console.log('Event Bubbling!'), false);
</script>
</body>
</html>
The output of the code:
As we can see, using the event capturing in JavaScript, the events are handled in the expected order.
Access the demo of the above example code here.
The main difference between these two events is that the event bubbling first handles the events from the innermost to the outermost, and the event capturing first handles the outermost element’s event.
After reading this article, we hope to have shown you how event bubbling and capturing work in JavaScript.
My name is Abid Ullah, and I am a software engineer. I love writing articles on programming, and my favorite topics are Python, PHP, JavaScript, and Linux. I tend to provide solutions to people in programming problems through my articles. I believe that I can bring a lot to you with my skills, experience, and qualification in technical writing.
LinkedIn