How to Trigger Event in JavaScript
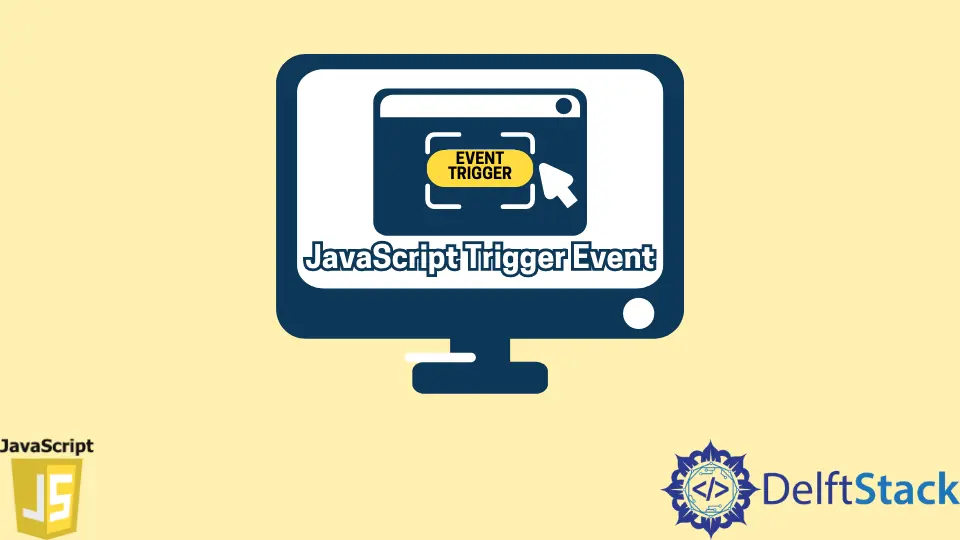
In JavaScript, a primitive method, initEvent()
, was used to create new events. The latest update added the new
keyword for building a custom event. Also, there is an eventful collection of events in the inventory built for JavaScript.
The following section will preview an example of one of the predefined events and how it gets triggered. And also a custom-built event that will have detail as the working mechanism. So, let’s jump in!
Trigger the Predefined Events in JavaScript
Here, we will select an input
textbox for value input and later use the onclick
attribute to ensure it is equivalent to an addEventListener()
method.
So, we take the instance of the input
field and set it through a conditional block. Then, whenever the click
event is triggered, the input
will be fetched and matched accordingly.
And thus, the function of the events gets fired.
Code Snippet:
<!DOCTYPE html>
<html>
<head>
<title>Trigger event</title>
</head>
<body>
<input type="text" id="vals">
<button onclick="abc()">Submit</button>
<script>
function abc(){
var x = document.getElementById("vals").value;
if(x ==='1'){
console.log("1")}
else{
console.log("No!")
}
}
</script>
</body>
</html>
Output:
Create Custom Event and Trigger in JavaScript
We will use a parent-child tree in a bubble event propagation to demonstrate this instance. The primary task is to create form
and textarea
elements, where the form
is the parent.
Next, we create an event with the new CustomEvent()
. The name of our event is cool
, and it takes the object of the bubbles
as true
, and the detail will be the value of the textarea
.
Later, the form
will have an eventListener
that will say that the cool
event will work on its content when fired. And lastly, we fire the event with dispatchEvent()
, and this is an eventListener
for the textarea
.
Let’s preview the code and output.
Code Snippet:
<!DOCTYPE html>
<html>
<head>
<title>Trigger event</title>
</head>
<body>
<form>
<textarea></textarea>
</form>
<script>
const form = document.querySelector('form');
const textarea = document.querySelector('textarea');
const event = new CustomEvent('cool', {
bubbles: true,
detail: { text: () => textarea.value }
});
form.addEventListener('cool', (e) => console.log(e.detail.text()));
textarea.addEventListener('input', (e) => e.target.dispatchEvent(event));
</script>
</body>
</html>
Output:
According to the custom event, we were supposed to print the value of the textarea
inputs. Then, finally, the console shows the output is executed.