The Push() Function in Java
-
Use the
stack.push()
Function in Java -
Use the
LinkedList.push()
Function in Java -
Use the
ArrayList.add()
Function in Java -
Use a User-Defined
push()
Function for Arrays in Java -
Use the
ArrayDeque.push()
Function in Java - Conclusion
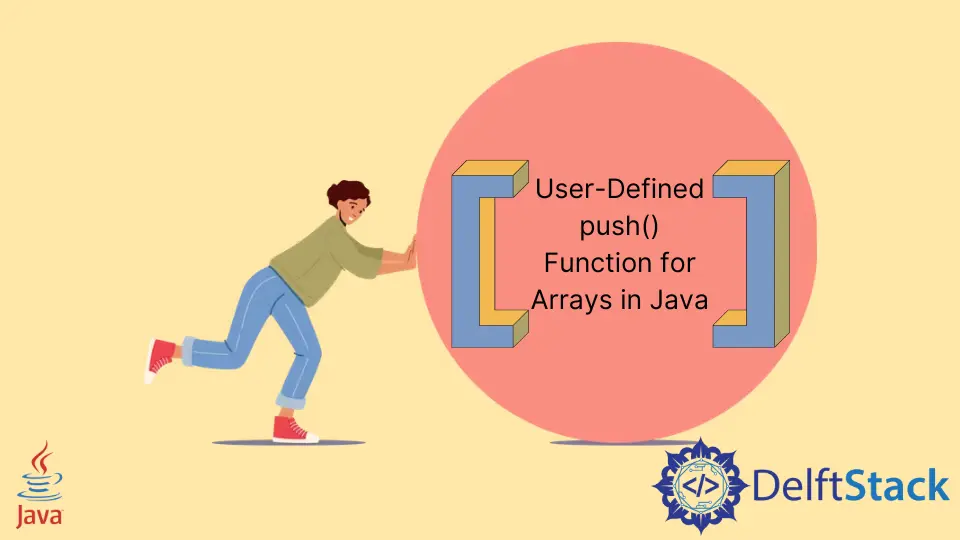
If we talk about the basic definition of the push()
function, it will be a function that inserts an element to the end of some structure. This function is associated with Last In First Out structures like stacks, linked lists, and more.
Since the push()
function is not associated with arrays, we can use different data structures that already support this function.
This article will discuss the push()
function in Java.
Use the stack.push()
Function in Java
We can use the push()
function from the stack class. For this, we will be importing the java.util
package for using the stack class.
With this function, we can add elements to the end of the stack. The stack can be of some desired type.
Let’s explore the push()
method through a practical example. In this scenario, we’ll create a simple program to demonstrate the usage of Stack.push()
:
import java.util.Stack;
public class StackExample {
public static void main(String[] args) {
// Creating a stack
Stack<String> stack = new Stack<>();
// Pushing elements onto the stack
stack.push("Java");
stack.push("is");
stack.push("awesome!");
// Printing the stack
System.out.println("Stack: " + stack);
// Popping elements from the stack
String poppedElement = stack.pop();
System.out.println("Popped Element: " + poppedElement);
// Printing the stack after popping
System.out.println("Stack after popping: " + stack);
}
}
To kick off our stack adventure, we create a new stack capable of holding strings. In Java, this involves instantiating an object of the Stack
class.
With our stack ready, it’s time to add some elements to it. The push()
method comes into play, enabling us to place items onto the top of the stack.
To gain insights into the current state of our stack, we utilize a simple print statement. This reveals the entire stack, providing a visual representation of the elements we’ve recently added.
As our stack story unfolds, we encounter the need to retrieve an element from the top of the stack. This is where the pop()
method comes into play, gracefully extracting the topmost element.
Another print statement allows us to witness the altered state of our stack, reflecting the transformation after the pop operation.
Output:
Stack: [Java, is, awesome!]
Popped Element: awesome!
Stack after popping: [Java, is]
Use the LinkedList.push()
Function in Java
The push()
method is an indispensable tool when working with LinkedLists
in Java. It caters specifically to this structure, allowing for the insertion of elements at the beginning of the list.
We can define a new linked list using the LinkedList
method. Now, we can add elements one by one using the push()
function.
Let’s delve into a practical illustration of the push()
method using a LinkedList
:
import java.util.LinkedList;
public class LinkedListExample {
public static void main(String[] args) {
// Creating a linked list
LinkedList<String> linkedList = new LinkedList<>();
// Pushing elements to the linked list
linkedList.push("Java");
linkedList.push("is");
linkedList.push("powerful!");
// Printing the linked list
System.out.println("Linked List: " + linkedList);
}
}
We embark on our journey by initializing a LinkedList
, a versatile data structure in Java capable of holding elements in a dynamic, linked fashion.
With our LinkedList
ready, we proceed to add elements using the push()
method. This method becomes our ally, facilitating the insertion of elements at the beginning of the LinkedList
, enhancing its dynamic nature.
To capture the evolving state of our LinkedList
, we employ a print statement. This line of code unveils the current composition of the LinkedList
, showcasing the elements added using the push()
method.
Output:
Linked List: [powerful!, is, Java]
Use the ArrayList.add()
Function in Java
ArrayLists
are widely employed in Java due to their versatility and ease of use. The add()
method, a pivotal part of the ArrayList
class, facilitates the addition of elements at the end of the list.
Consider the following Java program, which demonstrates the utilization of the add()
method in an ArrayList
:
import java.util.ArrayList;
public class ArrayListExample {
public static void main(String[] args) {
// Creating an ArrayList
ArrayList<String> arrayList = new ArrayList<>();
// Adding elements to the ArrayList
arrayList.add("Java");
arrayList.add("is");
arrayList.add("awesome!");
// Printing the ArrayList
System.out.println("ArrayList: " + arrayList);
}
}
We begin by initializing an ArrayList
, a dynamic array-based data structure in Java designed to hold elements of a specified type.
With the ArrayList
ready, we proceed to populate it with elements using the add()
method. This method seamlessly inserts elements at the end of the ArrayList
, contributing to its dynamic growth.
To unveil the evolving state of our ArrayList, we turn to a simple print statement. This line of code serves as a window into the current composition of the ArrayList
, offering a visual representation of the elements added using the add()
method.
Output:
ArrayList: [Java, is, awesome!]
Use a User-Defined push()
Function for Arrays in Java
There is no push()
function for arrays in Java. However, we can create a function to emulate this. This function will copy the array contents to a new array of a longer length and add the new element to this array.
In this article, we’ll explore the concept of a user-defined push()
function, a versatile tool that allows developers to seamlessly add elements to arrays in a personalized manner.
Let’s dive into the code to grasp the essence of a user-defined push()
function:
import java.util.*;
public class Push_Arr {
private static String[] push(String[] array, String push) {
String[] longer = new String[array.length + 1];
for (int i = 0; i < array.length; i++) longer[i] = array[i];
longer[array.length] = push;
return longer;
}
public static void main(String args[]) {
String[] arr = new String[] {"a", "b", "c"};
arr = Push_Arr.push(arr, "d");
for (int i = 0; i < arr.length; i++) System.out.println(arr[i]);
}
}
Creating the User-Defined push()
Method:
In this segment, a push()
method is crafted to receive an array (array
) and a string to be pushed (push
). A new array (longer
) is created with a length one greater than the original array.
A loop is then used to copy elements from the original array to the new array, and the push
element is added at the end.
Using the User-Defined push()
Method:
In the main
method, an array arr
is initialized with three elements. The user-defined push()
method is then invoked, adding the string d
to the array.
Finally, the modified array is printed to the console.
Output:
a
b
c
d
Use the ArrayDeque.push()
Function in Java
The ArrayDeque
class in Java is a double-ended queue that supports dynamic resizing. It provides various methods for both stack and queue operations.
The push()
method, typically associated with stacks, adds an element to the beginning of the deque, effectively pushing it onto the stack.
Let’s embark on a hands-on exploration of the ArrayDeque.push()
method through a practical example:
import java.util.ArrayDeque;
public class ArrayDequeExample {
public static void main(String[] args) {
// Creating an ArrayDeque
ArrayDeque<String> arrayDeque = new ArrayDeque<>();
// Pushing elements onto the ArrayDeque
arrayDeque.push("Java");
arrayDeque.push("is");
arrayDeque.push("powerful!");
// Printing the ArrayDeque
for (String element : arrayDeque) {
System.out.println(element);
}
}
}
First, we initialize an ArrayDeque
capable of holding strings. This data structure is well-suited for dynamic collections with fast insertion and removal at both ends.
Next, the push()
method is employed to add elements to the beginning of the deque, simulating a stack behavior. In this case, the strings Java
, is
, and powerful!
are pushed onto the deque.
Finally, the last segment prints the current state of the ArrayDeque
after pushing elements. The enhanced for loop iterates over the deque, printing each element.
Output:
powerful!
is
Java
Conclusion
The exploration of pivotal functions like push()
across contexts—Stack
, LinkedList
, ArrayList
, user-defined
scenarios, and ArrayDeque
—proffers developers a versatile toolkit. Understanding the push()
method in the Stack
class facilitates efficient last-in, first-out operations, extending seamlessly to linked structures through LinkedList.push()
.
The ArrayList.add()
method emerges as a linchpin for dynamic arrays, streamlining element addition crucial for data management. Crafting user-defined push()
functions showcases Java’s flexibility, emphasizing adaptability and expressive power.
ArrayDeque
’s push()
method, designed for dynamic stacks, excels in maintaining a last-in, first-out structure. In conclusion, a nuanced grasp of the push()
methods empowers Java developers to navigate diverse scenarios, fostering efficient, tailored code.