La función push() en Java
-
Utilice la función
stack.push()
en Java -
Utilice la función
LinkedList.push()
en Java -
Utilice la función
ArrayList.add()
en Java -
Utilice una función
push()
definida por el usuario para matrices en Java
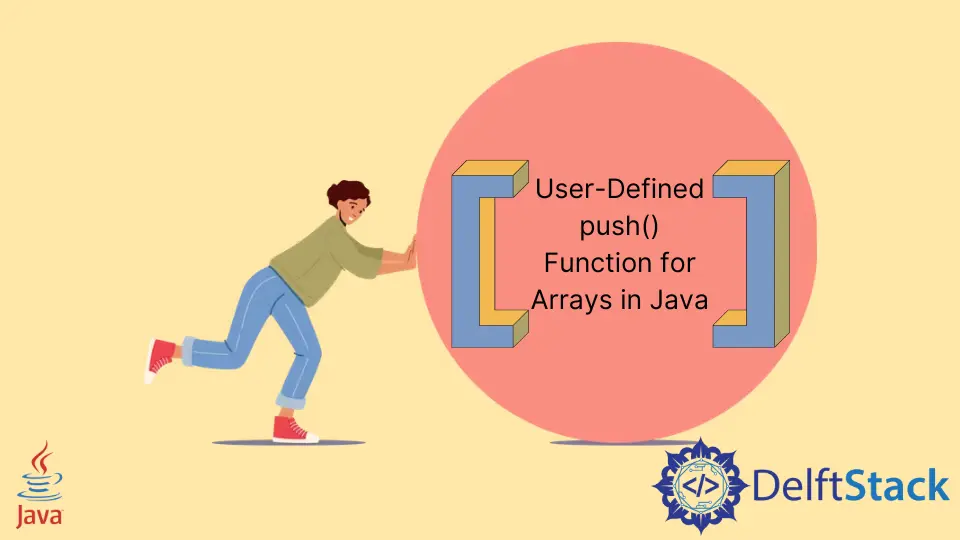
Si hablamos de la definición básica de la función push()
, será una función que inserta un elemento al final de alguna estructura. Esta función está asociada con estructuras de último en entrar, primero en salir, como pilas, listas enlazadas y más. Java no tiene una función push()
para matrices en él.
Dado que la función push()
no está asociada con matrices, podemos usar diferentes estructuras de datos que ya soportan esta función.
Este artículo discutirá la función push()
en Java.
Utilice la función stack.push()
en Java
Podemos usar la función push()
de la clase de pila. Para esto, estaremos importando el paquete java.util
para usar la clase de pila.
Con esta función, podemos agregar elementos al final de la pila. La pila puede ser de algún tipo deseado.
Crearemos el método de pila del tipo de cadena. Agregaremos los elementos uno por uno usando la función push()
.
Vea el código a continuación.
import java.util.*;
public class Push_Example {
public static void main(String args[]) {
Stack<String> st = new Stack<String>();
st.push("Ram");
st.push("shayam");
st.push("sharma");
System.out.println("Stack Elements: " + st);
st.push("monu");
st.push("sonu");
// Stack after adding new elements
System.out.println("Stack after using the push function: " + st);
}
}
Producción :
Stack Elements: [Ram, shayam, sharma]
Stack after using the push function: [Ram, shayam, sharma, monu, sonu]
Utilice la función LinkedList.push()
en Java
En Java, la función push()
también está asociada con Listas vinculadas. Para esto también, estaremos importando el paquete java.util
.
Podemos definir una nueva lista enlazada usando el método LinkedList
. Ahora, podemos agregar elementos uno por uno usando la función push()
.
Por ejemplo,
import java.util.*;
public class Push_Example {
public static void main(String args[]) {
LinkedList<Integer> li = new LinkedList<>();
li.push(10);
li.push(11);
li.push(12);
li.push(13);
li.push(14);
System.out.println("LinkedList Elements: " + li);
// Push new elements
li.push(100);
li.push(101);
System.out.println("LinkedList after using the push function: " + li);
}
}
Producción :
LinkedList Elements: [14, 13, 12, 11, 10]
LinkedList after using the push function: [101, 100, 14, 13, 12, 11, 10]
Utilice la función ArrayList.add()
en Java
Para ArrayLists, podemos usar la función add()
para emular la función push()
. Esta función agregará un elemento al final de la ArrayList dada.
Por ejemplo,
import java.util.*;
public class Push_Example {
public static void main(String args[]) {
ArrayList<Integer> li = new ArrayList<>();
li.add(10);
li.add(11);
li.add(12);
li.add(13);
li.add(14);
System.out.println("ArrayList Elements: " + li);
// Push new elements
li.add(100);
li.add(101);
System.out.println("ArrayList after using the add function: " + li);
}
}
Producción :
ArrayList Elements: [10, 11, 12, 13, 14]
ArrayList after using the add function: [10, 11, 12, 13, 14, 100, 101]
Utilice una función push()
definida por el usuario para matrices en Java
No hay función push()
para matrices en Java. Sin embargo, podemos crear una función para emular esto. Esta función copiará el contenido del array a una nueva matriz de mayor longitud y agregará el nuevo elemento a esta matriz.
Vea el código a continuación.
import java.util.*;
public class Push_Arr {
private static String[] push(String[] array, String push) {
String[] longer = new String[array.length + 1];
for (int i = 0; i < array.length; i++) longer[i] = array[i];
longer[array.length] = push;
return longer;
}
public static void main(String args[]) {
String[] arr = new String[] {"a", "b", "c"};
arr = Push_Arr.push(arr, "d");
System.out.println("Array after using the push function: ");
for (int i = 0; i < arr.length; i++) System.out.println(arr[i]);
}
}
Producción :
ArrayList after using the add function:
a
b
c
d
Este método proporciona la salida deseada, pero rara vez se usa porque ejecuta un bucle para copiar los elementos del array, por lo que tomará mucho tiempo y memoria cuando se trata de matrices más grandes.