Java 中的 push() 函式
Siddharth Swami
2023年10月12日
Java
Java Array
-
在 Java 中使用
stack.push()
函式 -
在 Java 中使用
LinkedList.push()
函式 -
在 Java 中使用
ArrayList.add()
函式 -
在 Java 中為陣列使用使用者定義的
push()
函式
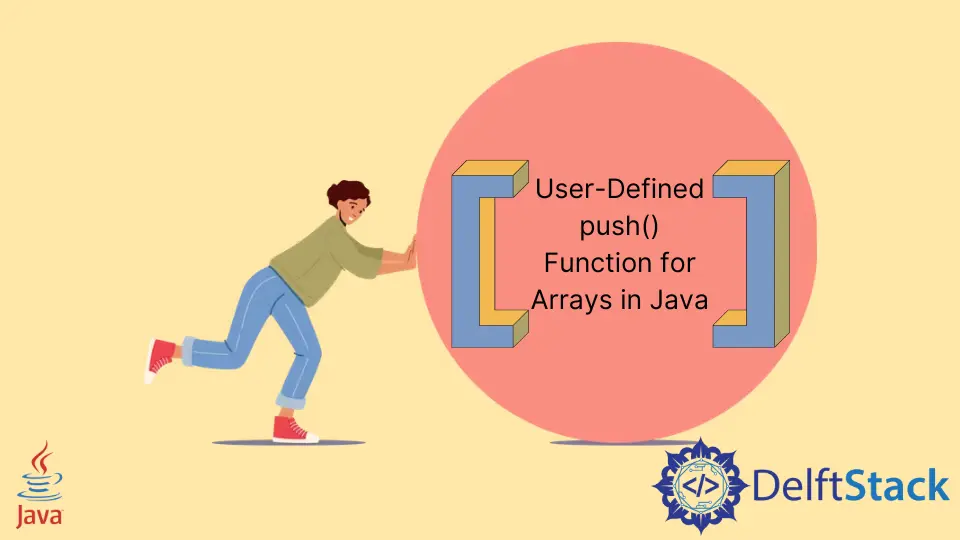
如果我們談論 push()
函式的基本定義,它將是一個將元素插入到某個結構末尾的函式。此函式與後進先出結構(如堆疊、連結串列等)相關聯。Java 中沒有用於陣列的 push()
函式。
由於 push()
函式與陣列無關,我們可以使用已經支援該函式的不同資料結構。
本文將討論 Java 中的 push()
函式。
在 Java 中使用 stack.push()
函式
我們可以使用 stack 類中的 push()
函式。為此,我們將匯入 java.util
包以使用堆疊類。
使用此函式,我們可以將元素新增到堆疊的末尾。堆疊可以是某種所需的型別。
我們將建立字串型別的堆疊方法。我們將使用 push()
函式一一新增元素。
請參閱下面的程式碼。
import java.util.*;
public class Push_Example {
public static void main(String args[]) {
Stack<String> st = new Stack<String>();
st.push("Ram");
st.push("shayam");
st.push("sharma");
System.out.println("Stack Elements: " + st);
st.push("monu");
st.push("sonu");
// Stack after adding new elements
System.out.println("Stack after using the push function: " + st);
}
}
輸出:
Stack Elements: [Ram, shayam, sharma]
Stack after using the push function: [Ram, shayam, sharma, monu, sonu]
在 Java 中使用 LinkedList.push()
函式
在 Java 中,push()
函式也與連結串列相關聯。為此,我們將匯入 java.util
包。
我們可以使用 LinkedList
方法定義一個新的連結串列。現在,我們可以使用 push()
函式一一新增元素。
例如,
import java.util.*;
public class Push_Example {
public static void main(String args[]) {
LinkedList<Integer> li = new LinkedList<>();
li.push(10);
li.push(11);
li.push(12);
li.push(13);
li.push(14);
System.out.println("LinkedList Elements: " + li);
// Push new elements
li.push(100);
li.push(101);
System.out.println("LinkedList after using the push function: " + li);
}
}
輸出:
LinkedList Elements: [14, 13, 12, 11, 10]
LinkedList after using the push function: [101, 100, 14, 13, 12, 11, 10]
在 Java 中使用 ArrayList.add()
函式
對於 ArrayLists,我們可以使用 add()
函式來模擬 push()
函式。此函式將在給定 ArrayList 的末尾新增一個元素。
例如,
import java.util.*;
public class Push_Example {
public static void main(String args[]) {
ArrayList<Integer> li = new ArrayList<>();
li.add(10);
li.add(11);
li.add(12);
li.add(13);
li.add(14);
System.out.println("ArrayList Elements: " + li);
// Push new elements
li.add(100);
li.add(101);
System.out.println("ArrayList after using the add function: " + li);
}
}
輸出:
ArrayList Elements: [10, 11, 12, 13, 14]
ArrayList after using the add function: [10, 11, 12, 13, 14, 100, 101]
在 Java 中為陣列使用使用者定義的 push()
函式
Java 中沒有用於陣列的 push()
函式。但是,我們可以建立一個函式來模擬這一點。該函式將把陣列內容複製到一個長度更長的新陣列中,並將新元素新增到這個陣列中。
請參閱下面的程式碼。
import java.util.*;
public class Push_Arr {
private static String[] push(String[] array, String push) {
String[] longer = new String[array.length + 1];
for (int i = 0; i < array.length; i++) longer[i] = array[i];
longer[array.length] = push;
return longer;
}
public static void main(String args[]) {
String[] arr = new String[] {"a", "b", "c"};
arr = Push_Arr.push(arr, "d");
System.out.println("Array after using the push function: ");
for (int i = 0; i < arr.length; i++) System.out.println(arr[i]);
}
}
輸出:
ArrayList after using the add function:
a
b
c
d
這個方法給出了想要的輸出但是很少使用,因為它執行一個迴圈來複制陣列元素,所以在處理更大的陣列時會花費大量的時間和記憶體。
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe