How to Pass an Array to a Method in Java
- Arrays in Java
- Methods in Java
- Pass Arrays to Methods in Java
- Pass Arrays to a Method in Java
- Pass a Multidimensional Array to a Method in Java
- Understand How Arrays are Passed to Methods in Java
- Pass an Array to a Method in Java
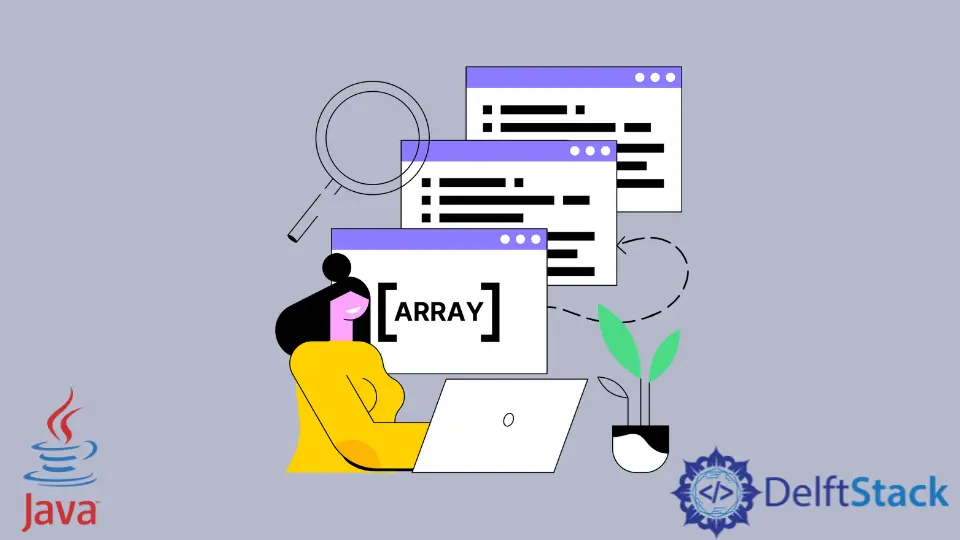
This tutorial introduces how to pass an array to a method in Java. We also listed some example codes to help you understand the topic.
Java is a powerful object-oriented programming language; it is a general-purpose programming language that can be used for various purposes. Let’s learn about arrays and methods in Java and understand how to pass an array to a method.
Arrays in Java
Arrays are a fixed-sized collection of the same data type. They are stored in the memory as contiguous blocks, and it enables us to randomly access any element of the array at a constant time.
This random access is possible because each element in an array has a dedicated index associated with it. We don’t have to traverse the whole array to reach a particular element. Array indices start from 0
and go to n-1
, where n
is the array’s length.
The following lines of code explain how to create an array and access its elements.
public class Main {
public static void main(String[] args) {
int[] arr; // Declaration
arr = new int[5]; // Creation
// Initialization
arr[0] = 1;
arr[1] = 3;
arr[2] = 5;
arr[3] = 7;
arr[4] = 9;
// Accessing Array Elements
System.out.println("Second Element: " + arr[1]);
System.out.println("Fourth Element: " + arr[3]);
}
}
Output:
Second Element: 3
Fourth Element: 7
Methods in Java
A method is defined as a set of instructions that can be used to accomplish a particular task. They are used to increase the reusability of our code.
For example, if we want to find the factorial of all numbers between 1
and 10
, it would be much better to define a method for factorial and call that method 10 times first instead of rewriting the entire logic of the factorial 10 different times.
Methods in Java are very similar to functions in other programming languages. The only difference is that methods are associated with an object while functions are not. Since Java is a completely object-oriented language, we only have methods in Java.
Pass Arrays to Methods in Java
A method may or may not take a fixed set of parameters. A parameter can be any variable that we need to use to define the body of the method.
In the example of a factorial method, a parameter can be the number whose factorial we need to find. But what if we need to pass an entire array to a method?
In the method declaration, we need to tell Java that the method must accept an array of a certain data type to pass an array to a method. Use the data type of the array and square brackets to denote that the parameter is an array.
// Method Declaration
public static void addTen(int[] arr) {
// Method Body
}
Whenever the method is called, we need to pass the array’s name to the method. The following example shows a complete code with a method that accepts an array and calls that method.
public class Main {
public static void addTen(int[] arr) // int[] denotes that the parameter is an array
{
for (int i = 0; i < arr.length; i++) {
arr[i] += 10;
}
}
public static void main(String[] args) {
int[] arr = {1, 3, 5, 7, 9};
addTen(arr); // Simply pass the name of the array to the method
for (int i = 0; i < arr.length; i++) {
System.out.print(arr[i] + " ");
}
}
}
Output:
11 13 15 17 19
Pass Arrays to a Method in Java
Consider another example where we pass two arrays of the same length to a method. The method should print the sum of the two arrays.
public class Main {
public static void addArrays(
int[] arr1, int[] arr2) // Two arrays are mentioned in the method declaration
{
for (int i = 0; i < arr1.length; i++) {
int sum = arr1[i] + arr2[i];
System.out.print(sum + " ");
}
}
public static void main(String[] args) {
int[] arr1 = {1, 2, 3, 4, 5};
int[] arr2 = {2, 4, 6, 8, 10};
addArrays(arr1, arr2); // Passing two arrays and calling the method
}
}
Output:
3 6 9 12 15
Pass a Multidimensional Array to a Method in Java
We can also pass a multi-dimension array to a method in Java. We need to specify the data type of the array elements and square brackets according to the dimension of the array.
Consider the following example where we find the sum of all the elements present in a 2-D array.
public class Main {
public static void sum(int[][] arr) {
int sum = 0;
for (int i = 0; i < arr.length; i++) {
for (int j = 0; j < arr[0].length; j++) {
sum = sum + arr[i][j];
}
}
System.out.print("Sum of all elements is: " + sum);
}
public static void main(String[] args) {
int[][] arr = {{1, 2, 3, 4, 5}, {2, 4, 6, 8, 10}, {1, 3, 5, 7, 9}};
sum(arr);
}
}
Output:
Sum of all elements is: 70
Understand How Arrays are Passed to Methods in Java
Let’s take a look and try to understand what happens behind the scenes when we pass a parameter to a method.
In Java, parameters are a pass by value type. It means that whenever we pass a variable to a method, a copy of the value of that variable is passed by what the method uses and not the original variable itself.
For example, let’s consider the following case where a method accepts an integer and adds 10
to that integer.
public class Main {
public static void addTen(int num) {
num = num + 10;
}
public static void main(String[] args) {
int a = 12;
addTen(a);
System.out.print(a);
}
}
Output:
12
What will you think the output of the code above will be? The value of the number should be incremented by 10, right?
What happens is that even though the integer is passed to the method, a copy of the integer value is what the method actually receives. So all the changes are made to that copy, and no change is made to the original integer. However, this only happens for primitive data types like int
.
This is not the case for arrays because arrays are not primitive data types and are considered container
objects that reference a memory location in the heap memory. As a result, they store the value of a memory location and not the actual data.
Whenever we change something in that memory location, that change will be visible to all the pointers(or references) pointing to that memory location. Remember that arrays are also passed by value in Java, but that value is actually a memory location.
Consider the code mentioned above, where we have created a method to add 10 to each element of an array.
Pass an Array to a Method in Java
We frequently need to pass a collection of the same type of data to a method. Arrays are best suited for these tasks, and we can pass an array to the method.
In the method declaration, we need to clearly specify that the method should accept an array of the mentioned data type; this is done using the data type and square brackets(for example, int[] arrayName).
While calling the method, we can enter the name of the array. In this tutorial, we also learned how methods treat arrays and how the memory location storing the elements of an array is updated.
Related Article - Java Array
- How to Concatenate Two Arrays in Java
- How to Convert Byte Array in Hex String in Java
- How to Remove Duplicates From Array in Java
- How to Count Repeated Elements in an Array in Java
- Natural Ordering in Java
- How to Slice an Array in Java