How to Use the wait() and notify() Methods in Java
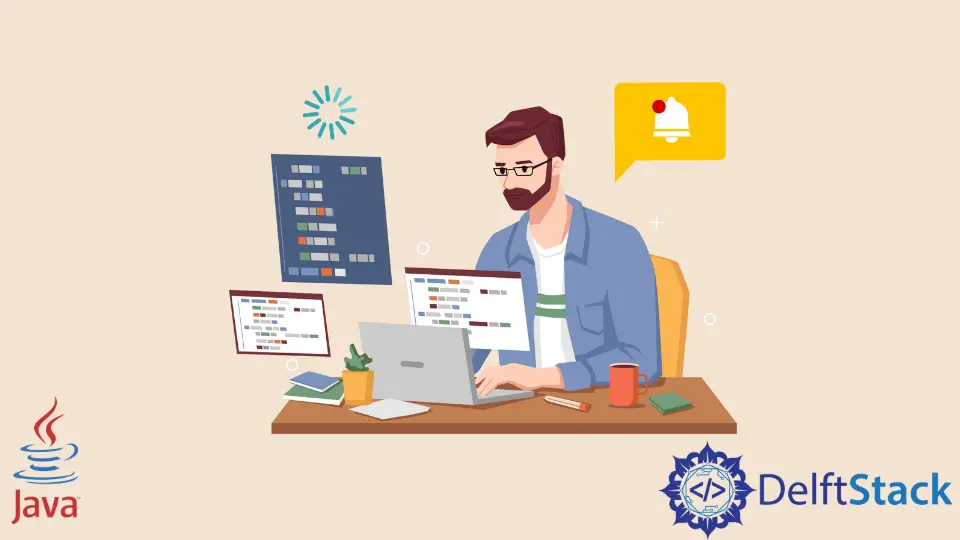
This tutorial demonstrates the wait()
and notify()
methods in Java.
Use the wait()
and notify()
Methods in Java
The wait()
and notify()
methods provide a mechanism to allow the thread to wait until a specific condition is met. For example, when you want to write blocking queue implementation for some fixed size backing store of elements.
The wait()
and notify()
are used in the guarded blocks in Java, which coordinate the actions of multiple threads. The wait()
method is used to suspend a thread and notify()
to wake up the thread.
the wait()
Method
The wait()
method will force the current thread to wait until some other threads are completed, or they invoke notify()
or notifyAll()
methods on the same object. The wait()
methods can be used in the following different ways:
-
Using
wait()
:This is the simple calling of the
wait()
method, which will cause the current thread to wait indefinitely until the other threads invoke thenotify()
ornotifyAll()
methods. -
Using
wait(long timeout)
:With this call of the
wait()
method, we can specify a timeout, after which the thread will be woken up automatically, where thetimeout
is a long time value.The thread can also be woken up before the timeout using the
notify()
andnotifyAll()
methods. Putting the value 0 in the timeout parameter will work similarly to thewait()
call. -
Using
wait(long timeout, int nanos)
:This call will also work similarly to the
wait(long timeout)
. The only difference is that we can provide a higher precision.The timeout period is calculated in nanoseconds as
1_000_000*timeout + nanos.
the notify()
and notifyAll()
Methods
The notify()
functionality is used to wake up the threads waiting to access the object monitor. The notify()
functionality can be used in two ways:
- Using
notify()
:
For all waiting threads, the `notify()` method will notify only one specific thread to wake up. Selecting the thread to wake up is not deterministic, which depends on the implementation.
Since the `notify()` can only wake up one thread, it might not be useful when we want to wake multiple threads.
-
Using
notifyAll()
:The
notifyAll()
will wake up all the threads on the object monitor. When the threads are awakened, they will complete in the usual manner like the other threads.
Let’s try an example that blocks the threads using the wait()
method and wakes up using the notify()
method:
package delftstack;
import java.util.LinkedList;
import java.util.Queue;
public class Example<T> {
private Queue<T> Demo_Queue = new LinkedList<T>();
private int Q_Capacity;
public Example(int Q_Capacity) {
this.Q_Capacity = Q_Capacity;
}
public synchronized void put(T Demo_Element) throws InterruptedException {
while (Demo_Queue.size() == Q_Capacity) {
wait();
}
Demo_Queue.add(Demo_Element);
// Wake up the thread. notifyAll() can also be used for multiple threads
notify();
}
public synchronized T take() throws InterruptedException {
while (Demo_Queue.isEmpty()) {
// Ask the thread to wait
wait();
}
T item = Demo_Queue.remove();
// Wake up the thread. notifyAll() can also be used for multiple threads
notify();
return item;
}
}
The code above demonstrates a simple queue blocking operation where we need to ensure that any wait()
and notify()
calls will be in the synchronized region of the code.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook