How to Custom Helper Method in Java
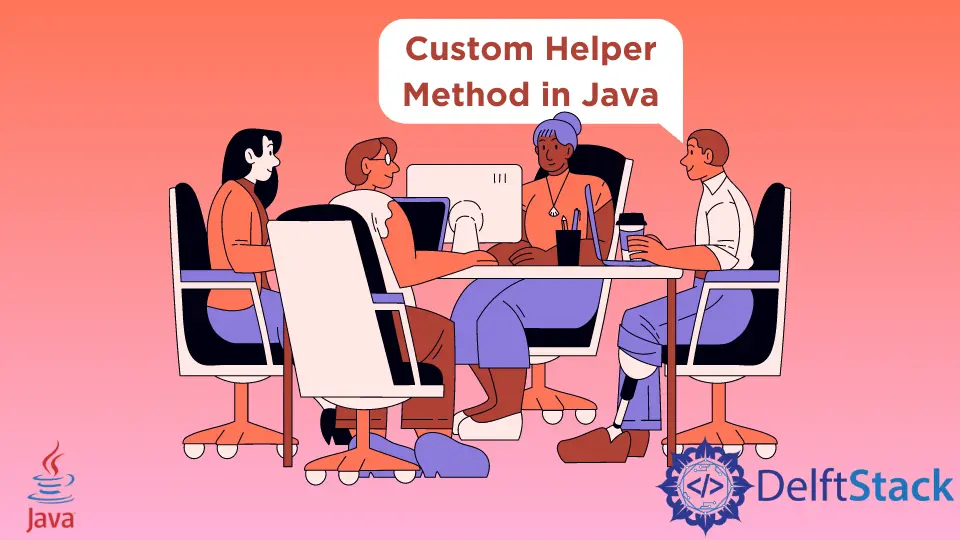
We create a helper class to help provide capabilities that are not the primary goal of the implementation or class to which it is implemented.
In brief, the helper objects are instances of a helper class. In the delegation pattern, the same helper object has been used.
This demonstration will help you understand such a helper method by showing you the implementation from scratch.
Helper Classes in Java
We can create a custom helper class by making all its functions static and its function constructor private. Besides, we have the option to make the class final (if needed).
Hence, it cannot be initialized, but all methodologies can be directly accessed. In Java, a helper method is used to perform a specific repetitive task shared between multiple classes.
This restricts us from reiterating the same piece of code in multiple courses. At the same time, the class-specific methods define its conduct and the helper methods aid in that process.
Please check Helper Class for more info.
Relationship of Utility Class With Helper Class in Java
A utility class is a subset of a helper class in which all of the methods are static. In general, helper classes are not necessary to have all static methods, but they may encompass instance variables.
There may be lots of instances of the helper class.
Demonstration of the Utility Class:
public class CustomHelper {
// Static function starts here
public static String funcOne(String str) {
return "An apple a day, keeps doctor away " + str + "!";
}
public static String funcTwo(String str) {
return "Pie in the sky " + str + "!";
}
public static String funcThree(String str) {
return "ABCDEFGHIJKLMNOPQRSTUVWXYZ " + str + "!";
}
}
Since we will create our helper method using the helper class and the same corresponding method discussed so far. Then only you will be able to understand it fully.
Implementation of Helper Class in Java
We will show you an elementary-level implementation of a helper method in Java. In total, there are two files here.
DefineHelper.java
RunHelper.java
Before further explaining, please look at the following code block of both files.
Code (1):
package helper.classdelfstack;
public class DefineHelper {
public static String str1(String print) {
return "An apple a day" + print;
}
public static String str2(String print) {
return "Out from the sky " + print;
}
public static String str3(String print) {
return "Money makes " + print;
}
}
We have used public static
for our main methods to initiate them with the main class.
Code (2):
package helper.classdelfstack;
public class RunHelper {
public static void main(String[] args) {
String print = " keeps doctor away!";
String print2 = " into the frying pan!";
String print3 = " mere go!";
System.out.println(DefineHelper.str1(print)); // An apple a day, keeps doctor away!
System.out.println(DefineHelper.str2(print2));
System.out.println(DefineHelper.str3(print3)); //
}
}
We run this custom helper class with the main function of RunHelper.java
.
Output:
An apple a day keeps doctor away!
Out from the sky into the frying pan!
Money makes mere go!
If you look closely, you’ll notice that we created a simple class, DefineHelper
, consisting of helper methods. This class could be used in multiple classes.
Now, if you look at the RunHelper
class, you’ll notice that we’ve added the following:
DefineHelper.str1()
DefineHelper.str2()
DefineHelper.str1()
Because the former class already has three string variables:
print
print2
print3
So, when we run it, we can access the RunHelper
class, but we can also prepend the DefineHelper
strings with constructors.
For example:
If we want to create more classes like these, all we have to do is implement the same helper methods for them. So, we would be capable of creating a personalized library of methods for use in our projects.
This type of elementary-level program can be expanded into a comprehensive library. This way, you can put a Java helper class to good use.
Sarwan Soomro is a freelance software engineer and an expert technical writer who loves writing and coding. He has 5 years of web development and 3 years of professional writing experience, and an MSs in computer science. In addition, he has numerous professional qualifications in the cloud, database, desktop, and online technologies. And has developed multi-technology programming guides for beginners and published many tech articles.
LinkedIn