How to Override and Overload Static Methods in Java
- Method Overloading
- Method Overriding
- Overload a Static Method in Java
- Override a Static Method in Java
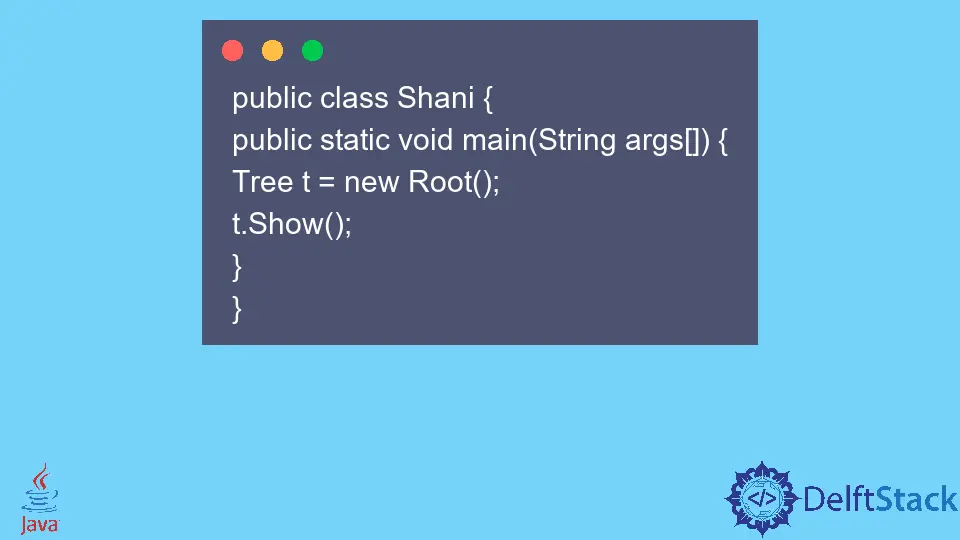
In this article, we will discuss whether or not it is possible to override and overload static methods in Java. But before we go any further, let’s make sure we’re clear on a few key points:
Java’s object-oriented nature makes overriding and overloading crucial features. When a programmer needs versatility, they can utilize this functionality.
Method Overloading
Compile-time polymorphism, also known as static polymorphism, is closely connected to overloading, another characteristic of Object-Oriented Programming languages like Java. This function allows many methods to have the same name while having distinct signatures.
These signatures may vary in various ways, including the number of input arguments and the kind of input parameters.
Static Method
A static method is a method that begins with the static
keyword. Class-level methods are another name for this concept.
The static
function is available to all class instances via a standard implementation.
Method Overriding
Overriding is a feature of object-oriented programming languages like Java that facilitates polymorphism at runtime. A method defined in a superclass is often implemented in a subclass or derived class.
The calling object determines which implementation will be performed at runtime. It’s important to remember that both methods’ signatures must be identical.
After an explanation of method overloading and overriding, we’ll talk about whether or not it is possible to override or overload a static method in Java.
Overload a Static Method in Java
Yes, we can overload static methods. However, it is crucial to remember that the method signature must be unique, or we can have two or more static methods with the same name but distinct input parameters.
Consider the following example to understand this concept better:
-
In the below example, we created two static methods named:
public static void A() {} public static void Z(int b) {}
-
After that, we just filled these methods with some data to be shown when called, such as the following:
public static void A() { System.out.println(" A() method is called: Hi, Muhammad Zeeshan here. "); }
-
Lastly, call both methods in the
main()
method to overload them:public static void main(String args[]) { Shani.A(); Shani.Z(2); }
Complete Code:
public class Shani {
public static void A() {
System.out.println(" A() method is called: Hi, Muhammad Zeeshan here. ");
}
public static void Z(int b) {
System.out.println(" Z(int b) method is called: Hello, Programmers. ");
}
public static void main(String args[]) {
Shani.A();
Shani.Z(2);
}
}
When we overload methods by calling in the main()
method, we’ll get the output as below:
A() method is called: Hi, Muhammad Zeeshan here.
Z(int b) method is called: Hello, Programmers.
Override a Static Method in Java
Static methods are bonded using static binding at compile time. That’s why we cannot override them because method overriding is predicated on dynamic binding at runtime, and the static methods are already bound using dynamic binding at runtime.
Therefore, we are unable to override static methods.
Let’s look at the following example to understand better:
- The
Tree
andRoot
classes below both have the same method signature, a static method calledShow()
.Root
’s hidden method covers the original function from the base class. - Calling a static method on an object of a
Root
class will invoke theRoot
class’s static method. - In the
main()
method, when we invoke a static method by passing in an object of theTree
class, the original static method is invoked, as we can see in the below example:
public class Shani {
public static void main(String args[]) {
Tree t = new Root();
t.Show();
}
}
Complete Code:
public class Shani {
public static void main(String args[]) {
Tree t = new Root();
t.Show();
}
}
class Tree {
public static void Show() {
System.out.println(" Show() method of the *Tree* class ");
}
}
class Root extends Tree {
public static void Show() {
System.out.println(" Overridden static method in *Root* class in Java ");
}
}
Output:
Show() method of the *Tree* class
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedIn