How to Create Pop Window in Java
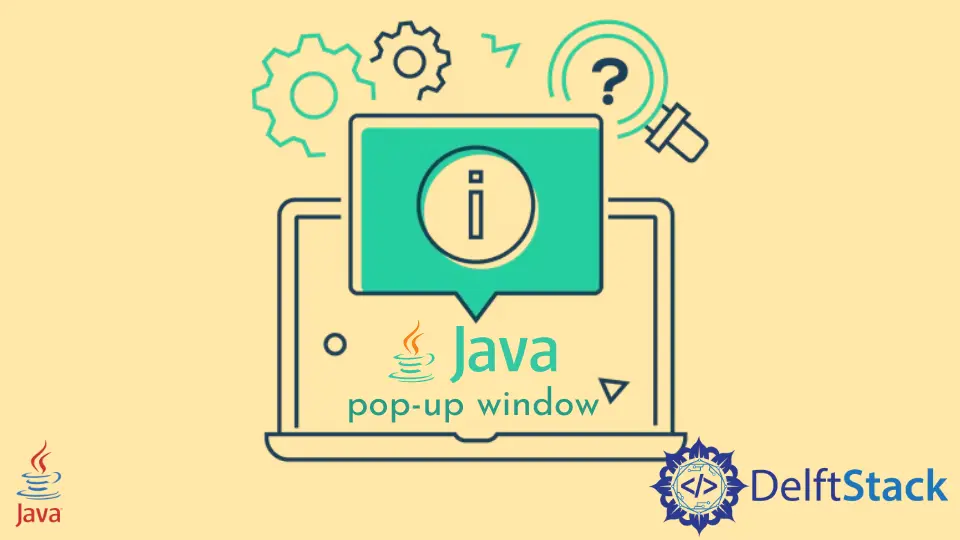
Java allows us to create several types of GUI components using libraries like AWT, Swing and, JavaFX.
In this tutorial, we’re going to look at the dialog and learn how to create it. We will use two classes, JOptionPane
and JDialog
, in the following examples.
Create Pop Up Window in Java Using JOptionPane
The simplest way to create a Java pop up window or a dialog is to use the JOptionPane
class as part of the javax.swing
package, which comes with many useful methods. Some of the common techniques are listed below:
Method | Description |
---|---|
showMessageDialog() | Displays a message inside a specified frame |
showInputDialog() | Get input from the user in the popup window |
showConfirmDialog() | Shows the message and asks the user for confirmation like yes, no, or cancel |
The following are the examples of the methods:
Example 1: Show a message inside a JFrame
by creating a Jframe
object, call the JOptionPane.showMessageDialog()
method, and pass the first jFrame
object as its first argument. The second argument is the message that we want to display in the dialog.
import javax.swing.*;
public class PopUpJava {
public static void main(String[] args) {
JFrame jFrame = new JFrame();
JOptionPane.showMessageDialog(jFrame, "Hello there! How are you today?");
}
}
Output:
Example 2: This example uses the JOptionPane.showInputDialog()
function to show a message with an input field to get the input. Here, to store the input, we use a String
variable and then show it to the user using showMessageDialog()
.
import javax.swing.*;
public class PopUpJava {
public static void main(String[] args) {
JFrame jFrame = new JFrame();
String getMessage = JOptionPane.showInputDialog(jFrame, "Enter your message");
JOptionPane.showMessageDialog(jFrame, "Your message: " + getMessage);
}
}
Output:
Example 3: We use the JOptionPane.showConfirmDialog()
function to show a dialog with three buttons: Yes, No, and Cancel. Just like the other methods, we pass a jFrame
object and a message to show. The showConfirmDialog()
method returns an int
that specifies the button that was clicked: 0 means Yes, 1 means No, and any other integer specifies that the cancel button was clicked.
import javax.swing.*;
public class PopUpJava {
public static void main(String[] args) {
JFrame jFrame = new JFrame();
int result = JOptionPane.showConfirmDialog(jFrame, "Press any button to close the dialog.");
if (result == 0)
System.out.println("You pressed Yes");
else if (result == 1)
System.out.println("You pressed NO");
else
System.out.println("You pressed Cancel");
}
}
Output:
Create Pop Up Window in Java Using JDialog
In the second method, we use the Jdialog
class. To create a frame, we need a jFrame
object that is passed to the constructor of JDialog()
as an argument when we create an object of JDialog
and jd
. Now we need to set the layout of the dialog that specifies how to place the components inside the dialog. We set the FlowLayout()
function that arranges the components in a line using jd.setLayout()
.
To set the position and the size of the pop window, we use the setBounds()
function, where the first two arguments are x and y positions, and the last two arguments are width and height.
We create a jLabel
variable with the text message passed inside the constructor to show a text. To enable the user to close the dialog, we create a JButton
object with the text Close
. Now, we have to set the action to perform when the close button is clicked. To do that, we call the addActionListener()
function that overrides the actionPerformed()
method in which we specify the action to be performed.
At last, we add all the components or objects that we created using jd.add()
.
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.*;
public class PopUpJava {
public static void main(String[] args) {
JFrame jFrame = new JFrame();
JDialog jd = new JDialog(jFrame);
jd.setLayout(new FlowLayout());
jd.setBounds(500, 300, 400, 300);
JLabel jLabel = new JLabel("Press close button to close the dialog.");
JButton jButton = new JButton("Close");
jButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
jd.setVisible(false);
}
});
jd.add(jLabel);
jd.add(jButton);
jd.setVisible(true);
}
}
Output:
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedInRelated Article - Java GUI
- Background Colors in Java
- How to Create Alert Popup in Java
- Button Group in Java
- Best GUI Builders for Java Swing Applications
- How to Create Java Progress Bar Using JProgressBar Class
- How to Create Tic Tac Toe GUI in Java