How to Create Canvas Using Java Swing
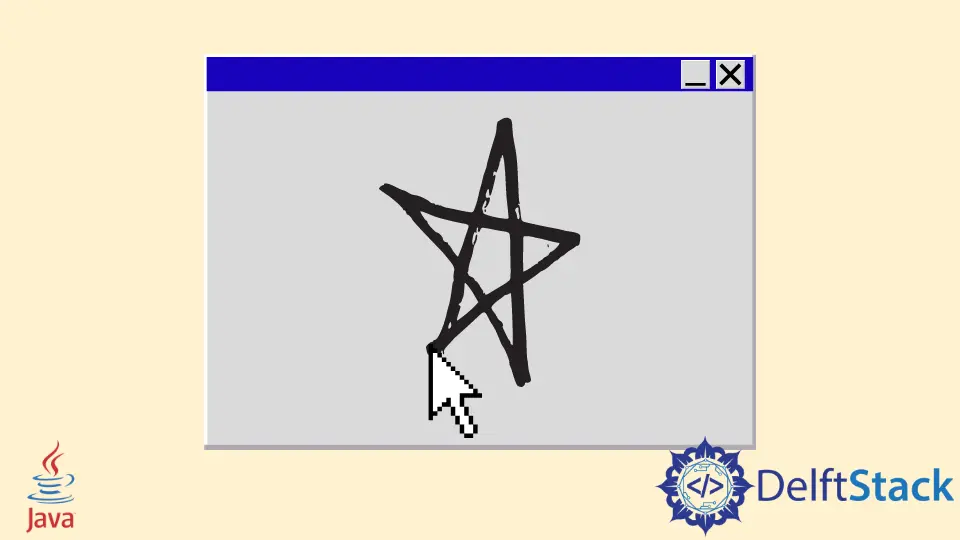
In Java, we can make a canvas in two ways, using Java AWT or Java Swing. Today, we will learn how to use Java Swing to make a canvas and draw shapes.
Use Java Swing To Create a Canvas
Example Code (the PaintPanel.java
Class):
// write your package here
package com.voidtesting.canvas;
// import necessary libraries
import java.awt.Graphics;
import java.awt.Point;
import java.awt.event.MouseEvent;
import java.awt.event.MouseMotionAdapter;
import javax.swing.JPanel;
public class PaintPanel extends JPanel {
// count the number of points
private int pointCounter = 0;
// array of 10000 Point references
private Point[] points = new Point[10000];
// make GUI and register the mouse event handler
public PaintPanel() {
// handles frame mouse motion event
addMouseMotionListener(new MouseMotionAdapter() {
// store the drag coordinates and repaint
@Override
public void mouseDragged(MouseEvent event) {
if (pointCounter < points.length) {
// find points
points[pointCounter] = event.getPoint();
// increment point's number in the array
++pointCounter;
// repaint JFrame
repaint();
} // end if
} // end mouseDragged method
} // end anonymous inner class
); // end call to the addMouseMotionListener
} // end PaintPanel constructor
/*
draw oval in a 5 by 5 bounding box at the given location
on the window
*/
@Override
public void paintComponent(Graphics g) {
// clear drawing area
super.paintComponent(g);
// draw all points that we have in array
for (int i = 0; i < pointCounter; i++) g.fillOval(points[i].x, points[i].y, 5, 5);
} // end paintComponent method
} // end PaintPanel Class
Example Code (The Canvas.java
Class):
// write your package here
package com.voidtesting.canvas;
// import necessary libraries
import java.awt.BorderLayout;
import java.awt.Label;
import javax.swing.JFrame;
public class Canvas {
public static void main(String[] args) {
// create JFrame Object
JFrame jFrame = new JFrame("Canvas Using Java Swing");
// create PaintPanel Object
PaintPanel paintPanel = new PaintPanel();
// add paintPanel in center
jFrame.add(paintPanel, BorderLayout.CENTER);
// place the created label in the south of BorderLayout
jFrame.add(new Label("Drag the mouse to draw"), BorderLayout.SOUTH);
// exit on close
jFrame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
// set frame size
jFrame.setSize(500, 400);
// set jFrame location to center of the screen
jFrame.setLocationRelativeTo(null);
// display frame
jFrame.setVisible(true);
}
}
Output:
In the PaintPanet.java
class, it extends
the JPanel
to create a committed area for drawing. The class Point
represent the x and y coordinates.
We create an object of the Point
class to save all the coordinates of every mouse drag event while the Graphics
class is used to draw. For this example, we use an array of Point
type containing the 10,000 points/coordinates that stores the location where every mouse drag event happens.
We can see that the paintComponent
use these coordinates to draw. Please note that the instance variable named pointCounter
still keeps track of the total number of points captured via mouse drag event.
As soon as it reaches the limit of 10,000, we will not be able to draw anymore.
Then, we register the MouseMotionListener
that can listen to the mouse motion event of the PaintPanel
class. Inside the addMouseMotionListener()
listener, we create an object of the anonymous inner class that extends the adapter class named MouseMotionAdapter
.
Why do we override mouseDragged
? Because the MouseMotionAdapter
implements the MouseMotionListener
, the anonymous inner class object is the MouseMotionListener
. The anonymous inner class inherits the default mouseMoved
and mouseDragged
implementations.
So, it already implements all the methods of the interface. However, the default method performs nothing whenever those are called, which is why we override the mouseDragged
to capture the points of the mouse drag event and save them as the Point
object.
The if
statement ensures that we only save the points in the array if we have the capacity. The getPoint()
method is invoked to retrieve the coordinates where the event happened, save them in the points
array at index pointCounter
, and then increment the pointCounter
as well.
Before getting out the if
statement, we use the repaint()
method that handles the updates to the paint cycle. Next, the paintComponent
method receives the parameter of Graphics
which is called automatically whenever the PaintPanel
must be displayed on the computer screen.
Inside the paintComponent
method, we invoke the superclass of the paintComponent
to the clear drawing area. Remember that we use the super
keyword to access the superclass’ methods and instances.
We draw a 5 by 5
oval at the given location by every point in an array which can go up to the pointCounter
while the fillOval()
method draws the solid oval.
Now, coming to the Canvas.java
, the main class. It creates the objects of JFrame
and PaintPanel
.
Then, we use the add()
method to add the object of the PaintPanel
to the center of the JFrame
window. We use BorderLayout.CENTER
to add it in the center of the JFrame
window.
Next, we add a Label
in the south of the JFrame
window using BorderLayout.SOUTH
. After that, we use setDefaultCloseOperation()
, setSize()
, setLocationRelativeTo()
, and setVisible()
methods to close the window when the user clicks on the cross sign (X
), set the size of the JFrame
window, move the JFrame
window to the center of the computer screen and display it respectively.
Instead of manually drawing on the canvas, we can draw programmatically.
Example Code (the Draw.java
Class has the main
method):
// write your package here
package com.voidtesting.canvas.draw;
// import necessary libraries
import java.awt.BorderLayout;
import java.awt.Color;
import java.awt.Graphics;
import javax.swing.JFrame;
import javax.swing.JPanel;
public class Draw extends JPanel {
@Override
public void paintComponent(Graphics g) {
// call method of the super class
super.paintComponent(g);
// set background color
this.setBackground(Color.cyan);
// set color of the shape
g.setColor(Color.red);
// draw line
g.drawLine(5, 30, 380, 30);
// set color of the shape
g.setColor(Color.blue);
// draw rectangular
g.drawRect(5, 40, 90, 55);
// set color of the shape
g.setColor(Color.BLACK);
// draw string
g.drawString("Hi, how are you?", 100, 50);
// set color of the shape
g.setColor(Color.green);
// draw filled rectangular
g.fill3DRect(5, 100, 90, 55, true);
// draw filled oval
g.fillOval(150, 100, 90, 55);
}
public static void main(String[] args) {
// create JFrame Object
JFrame jFrame = new JFrame("Canvas");
// add the object of Draw Class in center
jFrame.add(new Draw(), BorderLayout.CENTER);
// exit on close
jFrame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
// set frame size
jFrame.setSize(300, 200);
// set jFrame location to center of the screen
jFrame.setLocationRelativeTo(null);
// display frame
jFrame.setVisible(true);
}
}
Output: