Java Swing を使用して Canvas を作成する
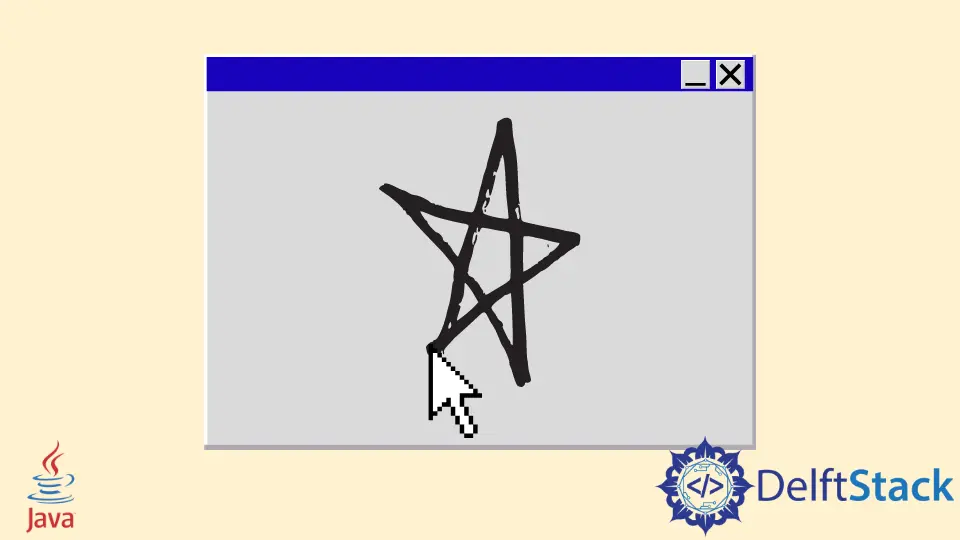
Java では、Java AWT または Java Swing を使用して、2つの方法でキャンバスを作成できます。今日は、Java Swing を使用してキャンバスを作成して図形を描画する方法を学習します。
Java Swing を使用してキャンバスを作成する
サンプルコード(PaintPanel.java
クラス):
// write your package here
package com.voidtesting.canvas;
// import necessary libraries
import java.awt.Graphics;
import java.awt.Point;
import java.awt.event.MouseEvent;
import java.awt.event.MouseMotionAdapter;
import javax.swing.JPanel;
public class PaintPanel extends JPanel {
// count the number of points
private int pointCounter = 0;
// array of 10000 Point references
private Point[] points = new Point[10000];
// make GUI and register the mouse event handler
public PaintPanel() {
// handles frame mouse motion event
addMouseMotionListener(new MouseMotionAdapter() {
// store the drag coordinates and repaint
@Override
public void mouseDragged(MouseEvent event) {
if (pointCounter < points.length) {
// find points
points[pointCounter] = event.getPoint();
// increment point's number in the array
++pointCounter;
// repaint JFrame
repaint();
} // end if
} // end mouseDragged method
} // end anonymous inner class
); // end call to the addMouseMotionListener
} // end PaintPanel constructor
/*
draw oval in a 5 by 5 bounding box at the given location
on the window
*/
@Override
public void paintComponent(Graphics g) {
// clear drawing area
super.paintComponent(g);
// draw all points that we have in array
for (int i = 0; i < pointCounter; i++) g.fillOval(points[i].x, points[i].y, 5, 5);
} // end paintComponent method
} // end PaintPanel Class
サンプルコード(Canvas.java
クラス):
// write your package here
package com.voidtesting.canvas;
// import necessary libraries
import java.awt.BorderLayout;
import java.awt.Label;
import javax.swing.JFrame;
public class Canvas {
public static void main(String[] args) {
// create JFrame Object
JFrame jFrame = new JFrame("Canvas Using Java Swing");
// create PaintPanel Object
PaintPanel paintPanel = new PaintPanel();
// add paintPanel in center
jFrame.add(paintPanel, BorderLayout.CENTER);
// place the created label in the south of BorderLayout
jFrame.add(new Label("Drag the mouse to draw"), BorderLayout.SOUTH);
// exit on close
jFrame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
// set frame size
jFrame.setSize(500, 400);
// set jFrame location to center of the screen
jFrame.setLocationRelativeTo(null);
// display frame
jFrame.setVisible(true);
}
}
出力:
PaintPanet.java
クラスでは、JPanel
を拡張
して、描画用のコミットされた領域を作成します。クラス Point
は x 座標と y 座標を表します。
Point
クラスのオブジェクトを作成して、すべてのマウスドラッグイベントのすべての座標を保存し、Graphics
クラスを使用して描画します。この例では、すべてのマウスドラッグイベントが発生する場所を格納する 10,000 ポイント/座標を含むポイント
タイプの配列を使用します。
paintComponent
がこれらの座標を使用して描画していることがわかります。pointCounter
という名前のインスタンス変数は、マウスドラッグイベントを介してキャプチャされたポイントの総数を引き続き追跡することに注意してください。
10,000 の制限に達すると、もう描画できなくなります。
次に、PaintPanel
クラスのマウスモーションイベントをリッスンできる MouseMotionListener
を登録します。addMouseMotionListener()
リスナー内に、MouseMotionAdapter
という名前のアダプタクラスを拡張する匿名内部クラスのオブジェクトを作成します。
なぜ mouseDragged
をオーバーライドするのですか?MouseMotionAdapter
は MouseMotionListener
を実装しているため、匿名の内部クラスオブジェクトは MouseMotionListener
です。匿名内部クラスは、デフォルトの mouseMoved
および mouseDragged
実装を継承します。
したがって、それはすでにインターフェースのすべてのメソッドを実装しています。ただし、デフォルトのメソッドは、それらが呼び出されるたびに何も実行しません。そのため、mouseDragged
をオーバーライドして、マウスドラッグイベントのポイントをキャプチャし、それらを Point
オブジェクトとして保存します。
if
ステートメントは、容量がある場合にのみ配列内のポイントを保存することを保証します。getPoint()
メソッドを呼び出して、イベントが発生した座標を取得し、それらをインデックス pointCounter
の points
配列に保存してから、pointCounter
もインクリメントします。
if
ステートメントを取得する前に、ペイントサイクルの更新を処理する repaint()
メソッドを使用します。次に、paintComponent
メソッドは、PaintPanel
をコンピューター画面に表示する必要があるときに自動的に呼び出される Graphics
のパラメーターを受け取ります。
paintComponent
メソッド内で、paintComponent
のスーパークラスを呼び出して、描画領域をクリアします。super
キーワードを使用して、スーパークラスのメソッドとインスタンスにアクセスすることを忘れないでください。
fillOval()
メソッドが実線の楕円を描画している間、pointCounter
まで上がることができる配列内のすべてのポイントによって、指定された場所に 5x5
の楕円を描画します。
さて、メインクラスの Canvas.java
に来てください。JFrame
と PaintPanel
のオブジェクトを作成します。
次に、add()
メソッドを使用して、PaintPanel
のオブジェクトを JFrame
ウィンドウの中央に追加します。BorderLayout.CENTER
を使用して、JFrame
ウィンドウの中央に追加します。
次に、BorderLayout.SOUTH
を使用して、JFrame
ウィンドウの南に Label
を追加します。その後、setDefaultCloseOperation()
、setSize()
、setLocationRelativeTo()
、および setVisible()
メソッドを使用して、ユーザーが十字記号(X
)をクリックしたときにウィンドウを閉じ、JFrame
ウィンドウのサイズで、JFrame
ウィンドウをコンピューター画面の中央に移動し、それぞれ表示します。
キャンバスに手動で描画する代わりに、プログラムで描画することができます。
サンプルコード(Draw.java
クラスには main
メソッドがあります):
// write your package here
package com.voidtesting.canvas.draw;
// import necessary libraries
import java.awt.BorderLayout;
import java.awt.Color;
import java.awt.Graphics;
import javax.swing.JFrame;
import javax.swing.JPanel;
public class Draw extends JPanel {
@Override
public void paintComponent(Graphics g) {
// call method of the super class
super.paintComponent(g);
// set background color
this.setBackground(Color.cyan);
// set color of the shape
g.setColor(Color.red);
// draw line
g.drawLine(5, 30, 380, 30);
// set color of the shape
g.setColor(Color.blue);
// draw rectangular
g.drawRect(5, 40, 90, 55);
// set color of the shape
g.setColor(Color.BLACK);
// draw string
g.drawString("Hi, how are you?", 100, 50);
// set color of the shape
g.setColor(Color.green);
// draw filled rectangular
g.fill3DRect(5, 100, 90, 55, true);
// draw filled oval
g.fillOval(150, 100, 90, 55);
}
public static void main(String[] args) {
// create JFrame Object
JFrame jFrame = new JFrame("Canvas");
// add the object of Draw Class in center
jFrame.add(new Draw(), BorderLayout.CENTER);
// exit on close
jFrame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
// set frame size
jFrame.setSize(300, 200);
// set jFrame location to center of the screen
jFrame.setLocationRelativeTo(null);
// display frame
jFrame.setVisible(true);
}
}
出力: