How to Clear Text Field in Java
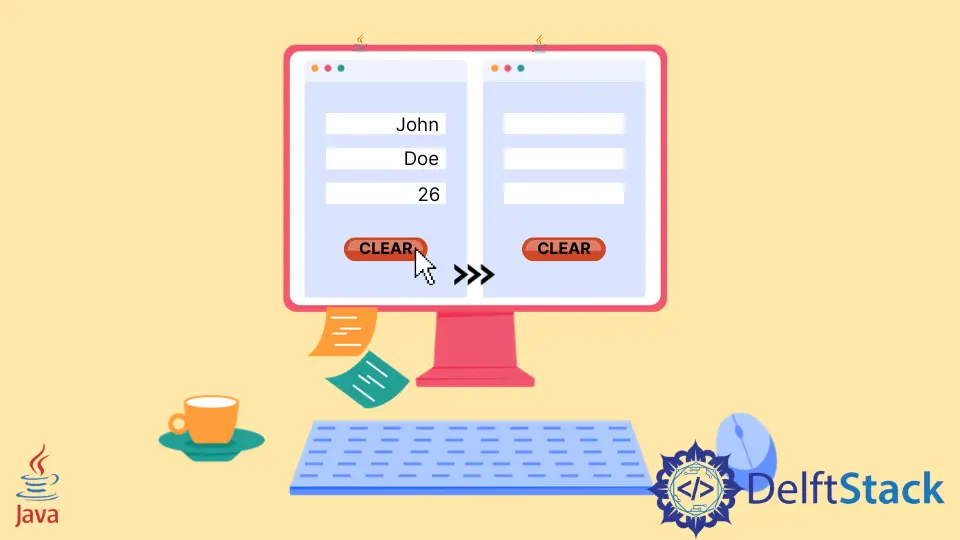
This topic is focused on how to clear the text fields using buttons in Java.
Text Field in Java
A text field is s component of GUI that allows you to enter data or information, which you can access and use according to your needs. In Java, we have a method clear()
of the JTextField
class, which helps us to clear all the data from the text fields.
Text fields are standard in both computer and mobile applications. Text fields can be used in applications like word processing and spreadsheets.
In a word processing document, the text field might allow the user to enter the body text of a letter, and in a spreadsheet, a text field might be used to enter the value of a cell.
Use a Button to Clear Text Field in Java
The Java Class Library has provided a way to clear text fields using the clear()
method of the JTextField
class. The JTextField
class is one of the classes we use to create a text field in a Java Swing application.
The clear()
method is defined in the JTextField
class and is used to clear the text inside the text field. The following program is used to clear the text field.
We create a text field object and then call the clear()
method to clear the text field.
Syntax:
JButton jb_clear = new JButton("Clear");
Many times you have to clear the text field in java. This can be done by calling the clear()
method of the JTextField
class.
Code Example:
package codes;
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class Codes implements ActionListener {
private final int size = 8;
private int sindex = 0;
JFrame jrf = new JFrame("Student Registration Form");
JMenuBar jmb = new JMenuBar();
JMenuItem jmi_new = new JMenuItem("New");
JMenuItem jmi_set = new JMenuItem("Setting");
JLabel jlfname = new JLabel("Email ");
JLabel jlname = new JLabel("First Name ");
JLabel jlpass = new JLabel("Password ");
JTextField jtname = new JTextField(20);
JTextField jtfname = new JTextField(20);
JTextField[] jtempty = new JTextField[size];
JPasswordField jp = new JPasswordField(20);
JButton jb_sub = new JButton("Submit");
JButton jb_clear = new JButton("Clear");
JButton jb_view = new JButton("View All");
Codes() {
createForm();
}
public void emptyTextField() {
for (int i = 0; i < size; i++) {
jtempty[i] = new JTextField(25);
jtempty[i].setEditable(false);
jtempty[i].setBorder(null);
jtempty[i].setBackground(Color.ORANGE);
}
}
public void createForm() {
emptyTextField();
jrf.setJMenuBar(jmb);
jrf.add(jlname);
jrf.add(jtname);
jrf.add(jtempty[0]);
jrf.add(jlfname);
jrf.add(jtfname);
jrf.add(jtempty[1]);
jrf.add(jlpass);
jrf.add(jp);
jrf.add(jtempty[2]);
jtempty[7].setColumns(30);
jrf.add(jtempty[7]);
jrf.add(jtempty[3]);
jrf.add(jtempty[4]);
jrf.add(jtempty[5]);
jrf.add(jtempty[6]);
jrf.add(jb_sub);
jrf.add(jb_clear);
jrf.add(jb_view);
jb_sub.addActionListener(this);
jb_clear.addActionListener(this);
jb_view.addActionListener(this);
jrf.setLayout(new FlowLayout());
jrf.setSize(350, 600);
// setResizable allow to not extend the frame size
jrf.setResizable(false);
// jrf.setBounds(100,0,350,700);
jrf.setVisible(true);
jrf.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
jrf.getContentPane().setBackground(Color.ORANGE);
}
@Override
public void actionPerformed(ActionEvent e) {
if (e.getSource().equals(jb_sub)) {
// JOptionPane.showMessageDialog(null, "Do you want to Submit Application");
submitData();
} else if (e.getSource().equals(jb_clear)) {
// JOptionPane.showMessageDialog(null,"It will remove Data","Student Regirstaion
// Data",JOptionPane.WARNING_MESSAGE);
clearForm();
}
}
void clearForm() {
jtname.setText("");
jtfname.setText("");
jp.setText("");
;
}
void submitData() {
String name = jtname.getText();
String fname = jtfname.getText();
String pass = String.valueOf(jp.getPassword());
clearForm();
jrf.setVisible(false);
}
public static void main(String[] args) {
Codes rf = new Codes();
}
}
Output:
The screen will show a registration form when we run the code above. After putting the information, you can remove that information by clicking on the clear button.
Conclusion
We discussed the different algorithms to clear the Java text field and have concluded that the fastest way to clear a text field is to create a new instance of the TextField
and assign it to the TextField
you want to clear. Then, we can call the clear()
method on the new TextField
, which will clear the original TextField
.
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedIn