How to Change the JLabel Text in Java Swing
- Method 1: Using ActionListener
- Method 2: Using MouseListener
- Method 3: Using Timer for Dynamic Updates
- Conclusion
- FAQ
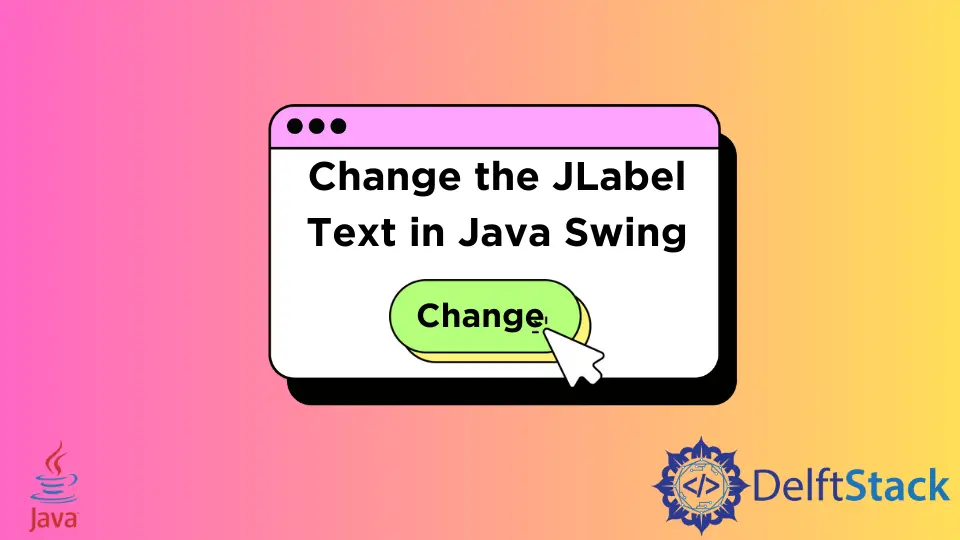
Changing the text of a JLabel in Java Swing is a fundamental skill for any Java developer working on graphical user interfaces (GUIs). Whether you’re building a simple application or a complex system, the ability to dynamically update the UI is crucial.
In this tutorial, we will explore various methods to change the JLabel text in Java Swing. You’ll learn how to do this effectively using event listeners and other techniques, all while keeping your application responsive and user-friendly. By the end of this article, you will have a solid understanding of how to manipulate JLabel text, enhancing your Java Swing applications.
Method 1: Using ActionListener
One of the most common ways to change the text of a JLabel in Java Swing is by using an ActionListener. This method is particularly useful when you want to update the label in response to user actions, such as button clicks. Below is an example demonstrating how to implement this.
import javax.swing.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class JLabelExample {
public static void main(String[] args) {
JFrame frame = new JFrame("JLabel Example");
JLabel label = new JLabel("Original Text");
JButton button = new JButton("Change Text");
button.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
label.setText("Text Changed!");
}
});
frame.setLayout(new java.awt.FlowLayout());
frame.add(label);
frame.add(button);
frame.setSize(300, 200);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
}
}
In this example, we create a simple JFrame with a JLabel and a JButton. The JLabel initially displays “Original Text”. When the button is clicked, the ActionListener triggers, updating the JLabel’s text to “Text Changed!”. This method is straightforward and effective, making it a common choice for dynamic text updates in Swing applications.
Method 2: Using MouseListener
Another approach to change JLabel text is by utilizing a MouseListener. This method allows you to update the label when the user interacts with it, such as hovering over or clicking the label itself. Below is an example demonstrating how to implement this functionality.
import javax.swing.*;
import java.awt.event.MouseAdapter;
import java.awt.event.MouseEvent;
public class JLabelMouseExample {
public static void main(String[] args) {
JFrame frame = new JFrame("JLabel Mouse Example");
JLabel label = new JLabel("Hover over me");
label.addMouseListener(new MouseAdapter() {
@Override
public void mouseClicked(MouseEvent e) {
label.setText("Label Clicked!");
}
@Override
public void mouseEntered(MouseEvent e) {
label.setText("Mouse Over!");
}
@Override
public void mouseExited(MouseEvent e) {
label.setText("Hover over me");
}
});
frame.setLayout(new java.awt.FlowLayout());
frame.add(label);
frame.setSize(300, 200);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
}
}
Output:
Initially, the JLabel shows "Hover over me". When the mouse hovers over it, it changes to "Mouse Over!". After clicking, it updates to "Label Clicked!" and returns to "Hover over me" when the mouse exits.
In this example, we create a JLabel that responds to mouse events. When the mouse enters the label area, it changes the text to “Mouse Over!”. If the label is clicked, it updates to “Label Clicked!”. Finally, when the mouse exits, it reverts to its original text. This method provides an interactive experience, making your GUI more engaging.
Method 3: Using Timer for Dynamic Updates
Sometimes, you may want to change the JLabel text dynamically over time, such as displaying a countdown or a status update. A Timer can be used for this purpose. Below is an example that demonstrates how to use a Timer to update the JLabel text every second.
import javax.swing.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class JLabelTimerExample {
public static void main(String[] args) {
JFrame frame = new JFrame("JLabel Timer Example");
JLabel label = new JLabel("Countdown: 10");
Timer timer = new Timer(1000, new ActionListener() {
int count = 10;
@Override
public void actionPerformed(ActionEvent e) {
if (count > 0) {
label.setText("Countdown: " + --count);
} else {
((Timer) e.getSource()).stop();
}
}
});
timer.start();
frame.setLayout(new java.awt.FlowLayout());
frame.add(label);
frame.setSize(300, 200);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
}
}
In this example, we create a Timer that triggers every second. Initially, the JLabel displays “Countdown: 10”. Each time the timer ticks, the label updates to show the current countdown value. When the countdown reaches zero, the timer stops. This method is particularly useful for applications that require real-time updates without user interaction, such as timers or status indicators.
Conclusion
In this tutorial, we’ve explored various methods to change the text of a JLabel in Java Swing. From using ActionListeners to MouseListeners and Timers, each approach offers unique advantages depending on the user interaction you want to achieve. Mastering these techniques will not only improve your Java GUI applications but also enhance the overall user experience. By implementing dynamic text updates, you can create more engaging and responsive applications that keep users informed and connected.
FAQ
-
How can I change the JLabel text without user interaction?
You can use a Timer to update the JLabel text at regular intervals. -
Can I change the JLabel text based on other events?
Yes, you can use various listeners like ActionListener, MouseListener, or even custom events to change the JLabel text. -
Is it possible to format the text in a JLabel?
Yes, you can use HTML tags within the JLabel text to format it, such as changing colors or font styles. -
What if I want to change multiple JLabels at once?
You can create a method that takes a JLabel as a parameter and updates its text, allowing you to call it for multiple labels. -
Are there any performance concerns with updating JLabel text frequently?
Generally, updating JLabel text is lightweight, but frequent updates in a short time frame may affect performance. Using a Timer with appropriate intervals is advisable.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn FacebookRelated Article - Java Swing
- How to Clear Text Field in Java
- How to Create Canvas Using Java Swing
- How to Use of SwingUtilities.invokeLater() in Java
- How to Center a JLabel in Swing
- Java Swing Date