How to Use of SwingUtilities.invokeLater() in Java
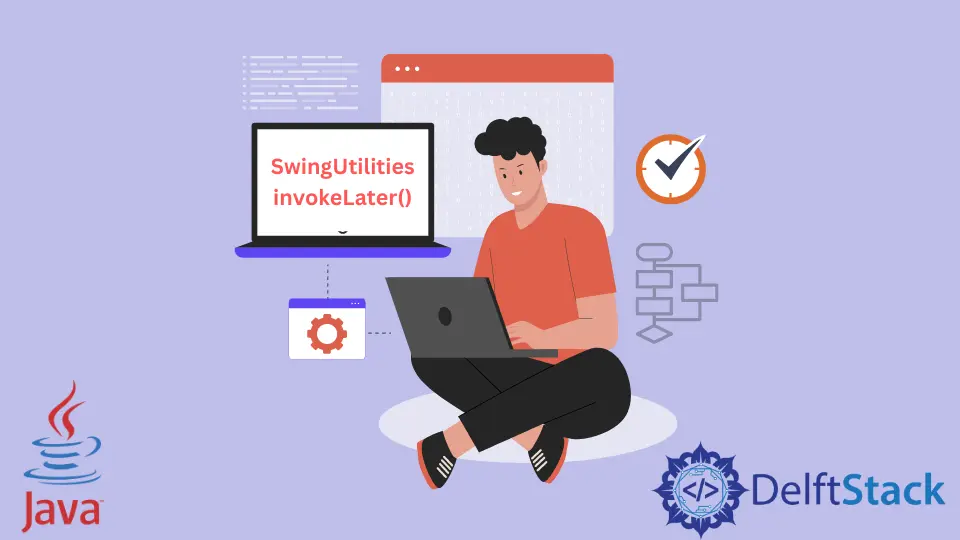
In Java programming, to provide developers with an easily achievable way of preventing concurrent access to them, the Swing
designers provided the rule that all code that accesses should run on the same thread.
The SwingUtilities.invokeLater()
method executes the runnable on the Abstract Window Toolkit (AWT) event-dispatching thread. We do that because Swing
data structures are not thread-safe.
Thread safety means that it may be used parallelly from more than one thread without causing any errors.
Use of the SwingUtilities.invokeLater()
Method in Java
The SwingUtilities.invokeLater()
method is important when using multithreading in Java applications and using Swing
as the user interface for the applications. Any updates in the user interface must happen on the event dispatch thread.
The code in the thread isn’t called straight away from an event handler, but we specifically arrange for our Graphical User Interface (GUI) update code, and in general, that code will be called on the event dispatch thread.
SwingUtilities.InvokLater()
code:
package codes;
import javax.swing.JFrame;
import javax.swing.SwingUtilities;
public class Codes {
protected static void frameUI() {
// Creating JFrame and calling from the main method
JFrame myFrame = new JFrame("Testing SwingUtilities.Invokelater");
myFrame.setBounds(20, 20, 350, 250);
myFrame.setVisible(true);
System.err.println(myFrame.getSize());
myFrame.setResizable(false);
System.err.println(myFrame.getSize());
}
public static void main(String[] args) {
SwingUtilities.invokeLater(new Runnable() {
@Override
public void run() {
frameUI(); // Calling Jframe
}
});
}
}
Event Dispatch Thread
In Java programming, specifically, when working with the Graphical User Interface (GUI) based programming with Swing
, you must have gone through multithreading and heard or used the event dispatch thread.
The event dispatch thread is the thread that calls out the event handlers. Essentially, the entire User Interface (UI) code will be executed by this thread.
It is a good practice to have a single designated thread handling the entire User Interface (UI) because it helps avoid many errors.
Java Threading
Threading is a general concept in computer programming. A thread is a lightweight sub-process of the overall process.
It improves the overall performance of application parallelism. The applications are divided into small executable units that are sometimes run independently and sometimes dependently depending on the nature of the application task.
Syntax of Thread
in Java:
Thread myThread = new Thread();
Each thread has one process, representing a separate flow of control. These are successfully implemented in web servers and network servers.
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedIn