JFileChooser Examples in Java
-
Using
JFileChooser
to Open a File and Show Its Name and Path in the Output in Java -
Using
JFileChooser
to Open an Image and Show It in aJFrame
Component in Java -
Using
JFileChooser
to Open a Text File and Show It in aJFrame
Component in Java
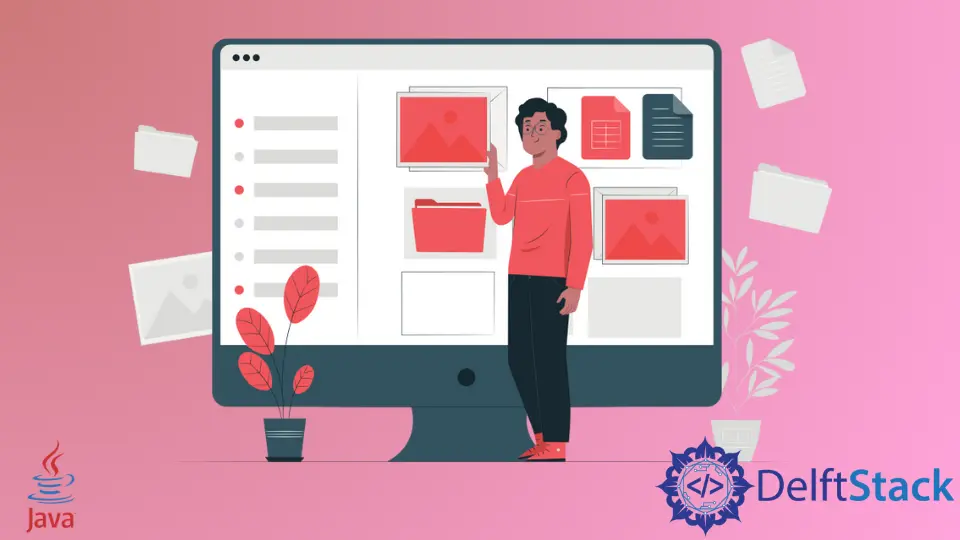
JFileChooser
provides us a window or a prompt that we can use to choose files or folders. It is a part of the Swing package.
We will see three examples of JFileChooser
in the following sections.
Using JFileChooser
to Open a File and Show Its Name and Path in the Output in Java
In the first example, we create a JFileChooser
class object. If we want to open a specific directory when the file chooser prompt is opened, we can pass the path to the constructor of JFileChooser
.
Now we call the showOpenDialog()
function that takes an argument which is the parent of the GUI of the file chooser; we pass null
in it because we only want the prompt.
According to the user input, the call to showOpenDialog()
returns an int
attached to a static field.
If the user clicks on OK on the dialog showOpenDialog()
, it returns an int
that represents the field APPROVE_OPTION
, and when it is returned, we check it in a condition.
In the conditional statement, we fetch the file that we select from the prompt using the getSelectedFile()
function that gives us a File
object. Now we can get the file’s name and its path.
import java.io.File;
import javax.swing.*;
public class JavaExample {
public static void main(String[] args) {
JFileChooser jFileChooser = new JFileChooser();
int checkInput = jFileChooser.showOpenDialog(null);
if (checkInput == JFileChooser.APPROVE_OPTION) {
File openedFile = jFileChooser.getSelectedFile();
System.out.println("File Name: " + openedFile.getName());
System.out.println("File Location: " + openedFile.getAbsolutePath());
}
}
}
Output:
File Name: New Text Document.txt
File Location: C:\Users\User\Documents\New Text Document.txt
Using JFileChooser
to Open an Image and Show It in a JFrame
Component in Java
We can choose and open different files using the JFileChooser
class, but we must manually process the files.
In the example, we choose and open an image using the JFileChooser
prompt and then display the image in a JFrame
component.
We create a JFrame
and two components: JButton
and JLabel
. We attach an ActionListener
to the JButton
by creating an anonymous class and overriding the actionPerformed()
function.
Inside the actionPerformed()
method, we create an object of JFileChooser
class.
For this example, we only want to choose images and restrict the file chooser prompt to only select image files, and for that, we need to create a filter.
First, we set the setAcceptAllFileFilterUsed()
to false to disable the prompt to choose all files, and then we create an object of FileNameExtensionFilter
.
In the constructor of FileNameExtensionFilter
, we pass two arguments: the description shown in the prompt and the extensions to be used, not that we can pass multiple extensions, as the second argument is a variable.
Next, we call the addChoosableFileFilter()
function and pass the FileNameExtensionFilter
object as its argument.
Like the previous example, we call the showOpenDialog()
function and then check if it returned the value representing the field APPROVE_OPTION
. If it did, we would execute the true block of the condition.
We call the getSelectedFile()
method and store it as a File
class reference to get the selected file.
We need to use the ImageIO
class to read the selected image file by calling its read()
method and passing the absolute path of the openedFile
.
The ImageIO.read()
function returns an Image
object that we use as an argument for the setIcon()
method of the JLabel
component, which sets the image as an icon for the label.
We add the components to the JFrame
and set its visibility to true,
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.io.File;
import java.io.IOException;
import javax.imageio.ImageIO;
import javax.swing.*;
import javax.swing.filechooser.FileNameExtensionFilter;
public class JavaExample {
public static void main(String[] args) {
JFrame jFrame = new JFrame("JFileChooser Example");
JButton jButton = new JButton("Open an Image");
jButton.setBounds(200, 20, 100, 30);
JLabel jLabel = new JLabel("");
jLabel.setBounds(10, 60, 400, 400);
ImageIcon imageIcon = new ImageIcon();
jButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
JFileChooser jFileChooser = new JFileChooser();
jFileChooser.setAcceptAllFileFilterUsed(false);
FileNameExtensionFilter fileNameExtensionFilter =
new FileNameExtensionFilter("Image file", "jpg", "jpeg", "PNG");
jFileChooser.addChoosableFileFilter(fileNameExtensionFilter);
int checkInput = jFileChooser.showOpenDialog(null);
if (checkInput == JFileChooser.APPROVE_OPTION) {
File openedFile = jFileChooser.getSelectedFile();
try {
Image image = ImageIO.read(openedFile.getAbsoluteFile());
imageIcon.setImage(image);
jLabel.setIcon(imageIcon);
} catch (IOException ioException) {
ioException.printStackTrace();
}
}
}
});
jFrame.add(jButton);
jFrame.add(jLabel);
jFrame.setSize(500, 500);
jFrame.setLayout(null);
jFrame.setVisible(true);
}
}
Output:
JFrame
Window with a JButton
:
JFileChooser
prompt:
JFrame
Window with a JButton
and the chosen image:
Using JFileChooser
to Open a Text File and Show It in a JFrame
Component in Java
In this example, we open a text file. Instead of a JLabel
component, we take a JtextArea
component to show the text from the chosen text file.
In the actionPerformed()
method, we create the JFileChooser
object and set the filter for the text file using the extension txt
. We get the selected file using the getSelectedFile()
function.
To read the text from the file, we first use a FileReader
passed in the constructor of BufferedReader
that returns a BufferedReader
object.
We get every line using the readLine()
method with this object. We create a string variable string1
and a StringBuilder
object string2
.
We use the readLine()
method and store every line in string1
and check if the line is null or not; if the line is null, that means there is no content in that line.
We append every line with string2
and a line break after every addition in the loop.
Now we set the string2
as the text of JTextArea
and close the BufferedReader
using the close()
method.
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.io.*;
import javax.swing.*;
import javax.swing.filechooser.FileNameExtensionFilter;
public class JavaExample {
public static void main(String[] args) {
JFrame jFrame = new JFrame("JFileChooser Example");
JButton jButton = new JButton("Open a text file");
jButton.setBounds(180, 20, 150, 30);
JTextArea jTextArea = new JTextArea("");
jTextArea.setBounds(10, 60, 480, 400);
jButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
JFileChooser jFileChooser = new JFileChooser();
jFileChooser.setAcceptAllFileFilterUsed(false);
FileNameExtensionFilter fileNameExtensionFilter =
new FileNameExtensionFilter("Text File", "txt");
jFileChooser.addChoosableFileFilter(fileNameExtensionFilter);
int checkInput = jFileChooser.showOpenDialog(null);
if (checkInput == JFileChooser.APPROVE_OPTION) {
File openedFile = jFileChooser.getSelectedFile();
try {
FileReader fileReader = new FileReader(openedFile);
BufferedReader bufferedReader = new BufferedReader(fileReader);
String string1 = "";
StringBuilder string2 = new StringBuilder();
while ((string1 = bufferedReader.readLine()) != null) {
string2.append(string1).append("\n");
}
jTextArea.setText(string2.toString());
bufferedReader.close();
} catch (IOException fileNotFoundException) {
fileNotFoundException.printStackTrace();
}
}
}
});
jFrame.add(jButton);
jFrame.add(jTextArea);
jFrame.setSize(500, 500);
jFrame.setLayout(null);
jFrame.setVisible(true);
}
}
Output:
JFrame
Window with a JButton
:
JFileChooser
prompt:
JFrame
Window with a JButton
and JTextArea
:
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedInRelated Article - Java Swing
- How to Clear Text Field in Java
- How to Create Canvas Using Java Swing
- How to Use of SwingUtilities.invokeLater() in Java
- How to Center a JLabel in Swing
- How to Change the JLabel Text in Java Swing
- Java Swing Date