How to Display an Image in Java
-
Display an Image in Java Using
javax.swing.ImageIcon
-
Display an Image in Java Using the
drawImage()
Method -
Display an Image in Java Using JavaFX’s
ImageView
- Conclusion
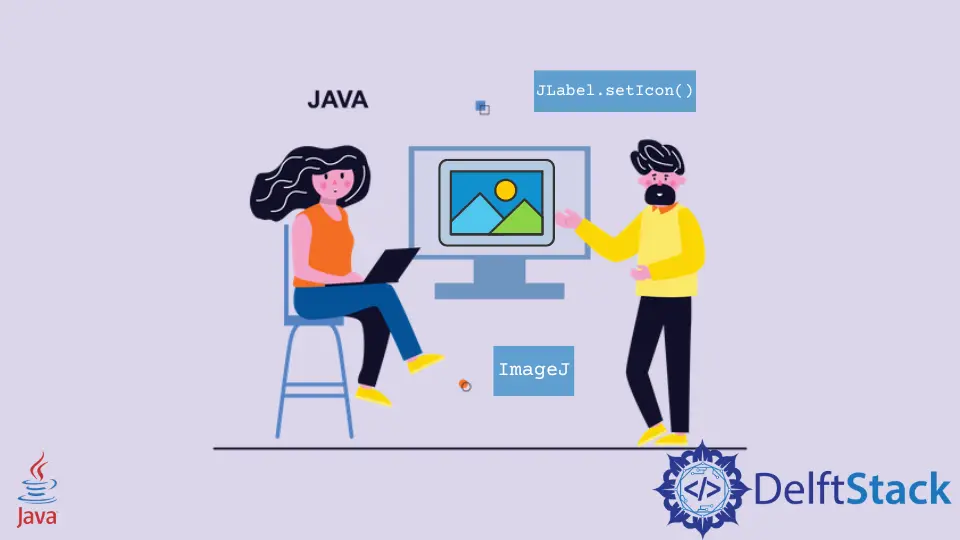
There are several ways to display images in Java, depending on the context and requirements of your application. We can show an image using several ways in Java.
In Java graphical user interface (GUI) applications, the ability to display images is a fundamental requirement. Whether you are developing a simple image viewer, a game, or a complex multimedia application, the process of loading and displaying images is a crucial aspect.
Display an Image in Java Using javax.swing.ImageIcon
The javax.swing.ImageIcon
class is part of the Swing framework, which provides a set of components for building desktop-based GUI applications. The javax.swing.ImageIcon
is a class in the Java Swing framework that represents an image icon.
It is used to display images in graphical user interfaces (GUIs). This class encapsulates image resources and provides a convenient way to load and manage images for use in Swing components such as buttons, labels, and panels.
Developers can create instances of ImageIcon
by specifying the image file’s path or URL and then easily integrate these icons into their Java GUI applications for a visually appealing user experience.
Let’s dive into a complete working example to illustrate how to display an image in Java using the ImageIcon
class. The following code snippet creates a basic Swing application that loads and displays an image within a JFrame
.
import javax.swing.*;
public class ImageDisplayExample extends JFrame {
public ImageDisplayExample() {
// Set the title of the JFrame
setTitle("Image Display Example");
// Create an instance of ImageIcon with the path to the image file
ImageIcon icon = new ImageIcon("path/to/your/image.jpg");
// Create a JLabel and set the ImageIcon as its icon
JLabel label = new JLabel(icon);
// Add the JLabel to the content pane of the JFrame
getContentPane().add(label);
// Pack the components to determine the JFrame size
pack();
// Set the default close operation and make the JFrame visible
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setVisible(true);
}
public static void main(String[] args) {
// Run the application on the Event Dispatch Thread (EDT)
SwingUtilities.invokeLater(() -> new ImageDisplayExample());
}
}
The ImageDisplayExample
class serves as a Swing-based GUI application window by extending the JFrame
class. To establish a recognizable title for the JFrame
, the setTitle
method is used.
An instance of ImageIcon
is created, specifying the path to the image file. This class is capable of loading and representing images.
Subsequently, a JLabel
is generated, and the previously created ImageIcon
is set as its icon. This JLabel
, responsible for displaying images, is added to the content pane of the JFrame
using the getContentPane().add(label)
method.
In order to ensure the appropriate sizing of components, the pack()
method is invoked.
The default close operation is set to EXIT_ON_CLOSE
, indicating the application’s behavior upon clicking the close button.
Finally, the SwingUtilities.invokeLater
method is employed to ensure that the GUI components are manipulated on the Event Dispatch Thread (EDT), a crucial aspect for Swing applications.
Output:
Upon execution, the program showcases the specified image in a dynamically sized JFrame
, offering a straightforward and effective approach to image display in Java applications.
Display an Image in Java Using the drawImage()
Method
In Java, the Graphics
class provides a versatile set of methods for drawing on components, and the drawImage()
method is particularly useful for rendering images.
It efficiently draws images onto graphical surfaces, such as screens or panels. Developers specify the image, destination coordinates, and optional scaling parameters, allowing for flexible and customized rendering.
This method is widely employed in Java graphical programming, providing a straightforward way to incorporate images into applications and games with ease.
In this section, we will explore how to use the drawImage()
method to display an image in a Java application.
Syntax:
void drawImage(Image img, int x, int y, ImageObserver observer)
The drawImage()
method in Java accepts three parameters.
Firstly, Image img
represents the image to be drawn, commonly obtained as an instance of the Image class using Toolkit.getDefaultToolkit().getImage()
.
Secondly, int x
and int y
determine the x and y coordinates where the top-left corner of the image will be positioned on the drawing surface. These coordinates are relative to the coordinate system of the drawing surface.
Lastly, the ImageObserver observer
parameter is an optional object that receives notifications as more of the image is converted.
Let’s dive into a complete working example that demonstrates how to use the drawImage()
method to display an image in Java.
import java.awt.*;
import java.awt.image.ImageObserver;
import javax.swing.*;
public class DrawImageExample extends JFrame {
private Image image;
public DrawImageExample() {
// Set the title of the JFrame
setTitle("drawImage() Example");
// Load the image using Toolkit
image = Toolkit.getDefaultToolkit().getImage("path/to/your/image.jpg");
// Set the default close operation and make the JFrame visible
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setSize(500, 500);
setVisible(true);
}
@Override
public void paint(Graphics g) {
// Call the superclass method to ensure proper rendering
super.paint(g);
// Draw the image on the JFrame using drawImage()
g.drawImage(image, 0, 0, this);
}
public static void main(String[] args) {
// Run the application on the Event Dispatch Thread (EDT)
SwingUtilities.invokeLater(DrawImageExample::new);
}
}
In the process of displaying an image in a Java application, the image is loaded using Toolkit.getDefaultToolkit().getImage
.
The default close operation of the JFrame
is then set to EXIT_ON_CLOSE
, and the JFrame
is made visible.
In order to customize the rendering behavior, the paint
method is overridden, ensuring proper rendering by calling the superclass method.
Subsequently, the loaded image is drawn onto the JFrame
using the drawImage
method, with parameters (0, 0, this)
specifying the position and size of the image.
Lastly, to guarantee proper GUI
component handling, the application runs on the Event Dispatch Thread (EDT)
through the use of the SwingUtilities.invokeLater
method, a crucial practice for Swing applications.
Upon running this program, a JFrame
window titled drawImage() Example
will appear, displaying the specified image.
Output:
The drawImage()
method is used to render the image directly onto the JFrame
, providing a simple yet effective way to display images in a Java application.
Display an Image in Java Using JavaFX’s ImageView
JavaFX’s ImageView
is a versatile UI control for displaying images in Java applications. It seamlessly integrates with the JavaFX scene graph, allowing developers to load and showcase images with minimal code.
With support for various image formats, scaling, and transformations, ImageView
enhances the visual appeal of user interfaces. Developers can dynamically update images, making it a powerful component for creating interactive and visually engaging JavaFX applications.
Let’s delve into a complete working example that demonstrates how to display an image using JavaFX’s ImageView
.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.image.Image;
import javafx.scene.image.ImageView;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
public class ImageViewExample extends Application {
@Override
public void start(Stage stage) {
// Create an Image object with the path to the image file
Image image = new Image("path/to/your/image.jpg");
// Create an ImageView and set the Image object as its image
ImageView imageView = new ImageView(image);
// Create a StackPane and add the ImageView to it
StackPane stackPane = new StackPane();
stackPane.getChildren().add(imageView);
// Create a Scene and set the ImageView as its root node
Scene scene = new Scene(imageView, 400, 300);
// Set the title of the Stage
stage.setTitle("ImageView Example");
// Set the Scene to the Stage
stage.setScene(scene);
// Display the Stage
stage.show();
}
public static void main(String[] args) {
// Launch the JavaFX application
launch(args);
}
}
The ImageViewExample
class extends the Application
class, signifying its nature as a JavaFX application.
The entry point of the application is the overridden start
method, which receives a Stage
parameter representing the main window.
The image to be displayed is loaded using an Image
object with the provided file path.
An ImageView
is then created, and the Image
object is set as its image.
A StackPane
is used as the root node for the scene and adds the ImageView
to it. Now, the Scene
constructor accepts a StackPane
(which is a Parent
).
A Scene
is created, with the ImageView
serving as its root node and specific dimensions defined.
Properties of the Stage
are set, including its title and the association with the created Scene
.
Finally, the Stage
is displayed using the show
method, and the application is launched through the main
method calling the launch
method.
Output:
Executing the program reveals a JavaFX window featuring the specified image, set at 400x300 pixels for optimal viewing. The image is loaded into the ImageView
and seamlessly rendered within the JavaFX application.
Conclusion
The selection of the appropriate image-handling method in Java depends on the specific requirements of the application.
Leverage javax.swing.ImageIcon
for simple image display in Java Swing applications. This versatile method seamlessly integrates images into graphical interfaces, proving essential for various projects.
In contrast, Java’s drawImage()
method provides a customizable approach to image rendering. Manipulating the graphical process with the Graphics
class enhances the visual aspects of Java applications.
JavaFX’s ImageView
class simplifies image display, offering a powerful mechanism for incorporating visuals into Java applications, which is particularly beneficial for enhancing graphical aspects with JavaFX.
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedInRelated Article - Java JLabel
- How to Center a JLabel in Swing
- How to Change the JLabel Text in Java Swing
- JFileChooser Examples in Java
- How to Add ActionListener to JButton in Java
- How to Use setFont in Java