How to Create Alert Popup in Java
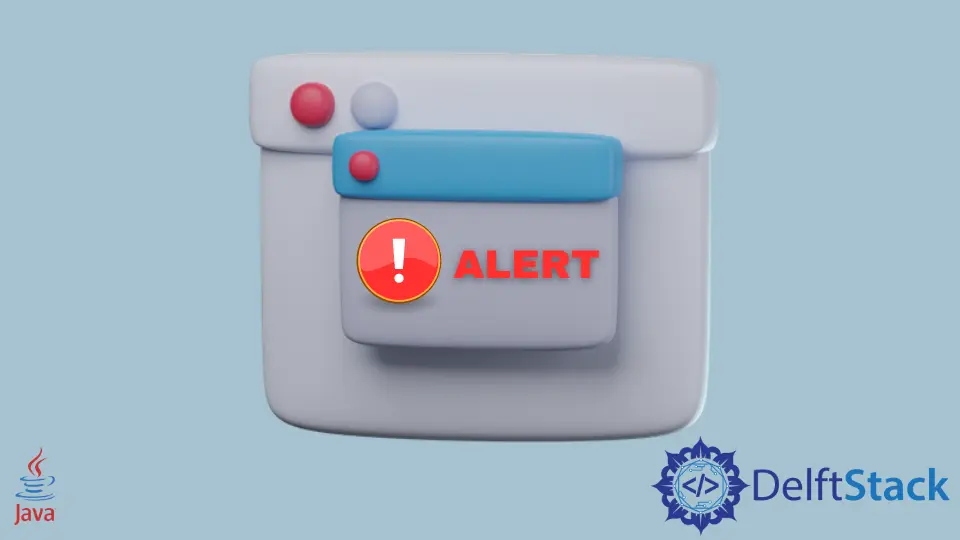
The Swing
library shows the alert popups in Java. This tutorial demonstrates how to create an alert message in Java.
In Java, an alert popup is a graphical user interface element used to notify users of important information, prompt for input, or confirm an action. Typically implemented using dialog boxes, these popups provide a non-intrusive way for Java applications to interact with users.
The alert popup can be customized to display messages, receive user input, or request confirmation through buttons such as OK
, Cancel
, or Yes/No
. By leveraging classes like JOptionPane
in Swing
, developers can easily integrate alert popups into their applications, enhancing user experience and facilitating clear communication between the software and its users.
Alert Popup in Java
One of the fundamental ways to communicate with users is through alert popups. These popups serve as a means to convey important information, receive user input, or prompt decisions.
In this article, we will delve into the concept of creating alert popups in Java using the JOptionPane
class from the Swing
library. Swing
provides a simple and effective way to display message dialogs that can be used for informational messages, user input, and confirmations.
Example:
import javax.swing.JOptionPane;
public class AlertPopupExample {
public static void main(String[] args) {
// Display a simple message dialog
JOptionPane.showMessageDialog(null, "Welcome to Java Swing!");
// Get user input through an input dialog
String userInput = JOptionPane.showInputDialog("Enter your name:");
// Display a confirmation dialog
int userChoice = JOptionPane.showConfirmDialog(
null, "Do you want to continue?", "Confirmation", JOptionPane.YES_NO_OPTION);
if (userChoice == JOptionPane.YES_OPTION) {
JOptionPane.showMessageDialog(null, "Hello, " + userInput + "! You chose to continue.");
} else {
JOptionPane.showMessageDialog(null, "Goodbye, " + userInput + "! You chose not to continue.");
}
}
}
In the provided Java code example, we begin by importing the JOptionPane
class from the Swing
library, a necessary step for utilizing its methods to create and manage dialogs. The main class, named AlertPopupExample
, serves as the container for our demonstration.
Subsequently, the code showcases the creation and display of a message dialog featuring the welcoming message Welcome to Java Swing!
—an illustrative instance of conveying information to users.
To engage users further, an input dialog is employed, prompting them to enter their name. The inputted value is then captured and stored in the userInput
variable, demonstrating the versatility of the JOptionPane
class in collecting user data.
Following this, a confirmation dialog is presented, posing the question, Do you want to continue?
with options for both Yes
and No
. The user’s decision is stored in the userChoice
variable, showcasing the utility of confirmation dialogs in obtaining user preferences.
The subsequent conditional handling section demonstrates how to tailor the program’s response based on the user’s choice. Depending on whether the user selects Yes
or No
, a corresponding message is displayed, underscoring the integration of user choices into the application’s logic.
Output:
![]() |
![]() |
![]() |
---|
![]() |
![]() |
---|
When you run this program, it will first display the welcome message, prompt you to enter your name, and then ask if you want to continue. Depending on your choices, personalized messages will be displayed, showcasing the effective use of message dialogs for user interaction.
Conclusion
The JOptionPane
class in Java Swing
simplifies the process of creating alert popups for user interaction. Whether it’s displaying information, collecting user input, or seeking confirmation, the JOptionPane
message dialog method provides a versatile and user-friendly approach.
By integrating these dialogs into your Java applications, you can enhance the user experience and create more interactive and responsive software.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook