Button Group in Java
- Understanding Button Groups in Java
- Creating a Basic Button Group
- Adding Action Listeners to Button Groups
- Customizing Button Groups with Icons
- Conclusion
- FAQ
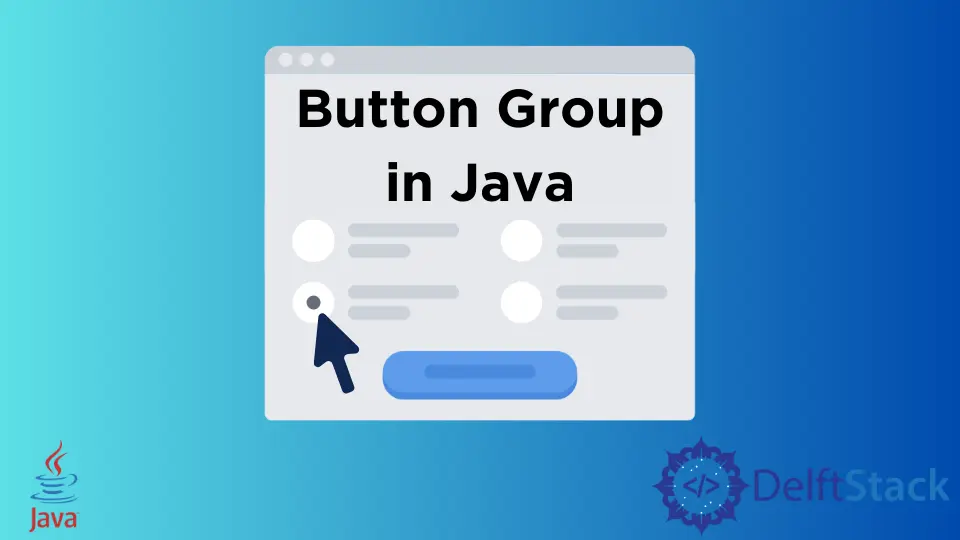
Creating a button group in Java can significantly enhance the user experience of your applications. Button groups allow you to manage a set of buttons, ensuring that only one button can be selected at a time. This feature is particularly useful in scenarios where you want users to make a single choice from multiple options, such as selecting a payment method or a survey response.
In this tutorial, we will walk you through the process of creating button groups in Java using the Swing framework. With clear examples and detailed explanations, you’ll be able to implement this functionality in your applications seamlessly.
Understanding Button Groups in Java
Before diving into the code, it’s essential to understand what a button group is and how it functions within a Java application. A button group is a component that groups together multiple buttons, allowing for exclusive selection. When a user selects one button in the group, any previously selected button is automatically deselected. This behavior is crucial for maintaining a clear and intuitive user interface.
In Java, the ButtonGroup
class is used to create a button group. It can contain instances of JRadioButton
or JCheckBox
, but typically, JRadioButton
is used for exclusive selection. The ButtonGroup
class is part of the Swing library, which provides a rich set of GUI components for building desktop applications.
Creating a Basic Button Group
To get started, let’s create a simple button group using JRadioButton
. This example will demonstrate how to set up a JFrame with a button group containing three radio buttons.
import javax.swing.*;
import java.awt.*;
public class ButtonGroupExample {
public static void main(String[] args) {
JFrame frame = new JFrame("Button Group Example");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize(300, 200);
JPanel panel = new JPanel();
JRadioButton option1 = new JRadioButton("Option 1");
JRadioButton option2 = new JRadioButton("Option 2");
JRadioButton option3 = new JRadioButton("Option 3");
ButtonGroup group = new ButtonGroup();
group.add(option1);
group.add(option2);
group.add(option3);
panel.add(option1);
panel.add(option2);
panel.add(option3);
frame.add(panel);
frame.setVisible(true);
}
}
Output:
A window will open displaying three radio buttons: Option 1, Option 2, and Option 3.
In this example, we first import the necessary Swing classes. We create a JFrame
to hold our button group and set its size and default close operation. Next, we create a JPanel
to contain our radio buttons. We instantiate three JRadioButton
objects and add them to a ButtonGroup
. Finally, we add the panel to the frame and make it visible.
This simple setup allows users to select only one option at a time, ensuring that your application remains user-friendly.
Adding Action Listeners to Button Groups
While the previous example shows how to create a button group, it’s often necessary to respond to user selections. To achieve this, we can add action listeners to our radio buttons. Let’s modify the previous example to include action listeners that display the selected option.
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class ButtonGroupWithListener {
public static void main(String[] args) {
JFrame frame = new JFrame("Button Group with Listener");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize(300, 200);
JPanel panel = new JPanel();
JRadioButton option1 = new JRadioButton("Option 1");
JRadioButton option2 = new JRadioButton("Option 2");
JRadioButton option3 = new JRadioButton("Option 3");
ButtonGroup group = new ButtonGroup();
group.add(option1);
group.add(option2);
group.add(option3);
ActionListener listener = new ActionListener() {
public void actionPerformed(ActionEvent e) {
String selectedOption = e.getActionCommand();
JOptionPane.showMessageDialog(frame, "You selected: " + selectedOption);
}
};
option1.addActionListener(listener);
option2.addActionListener(listener);
option3.addActionListener(listener);
panel.add(option1);
panel.add(option2);
panel.add(option3);
frame.add(panel);
frame.setVisible(true);
}
}
Output:
In this example, we added an ActionListener
to each radio button. When a button is selected, the listener triggers a dialog box displaying the selected option. This interactivity enhances user engagement and provides immediate feedback.
Customizing Button Groups with Icons
To make your button groups more visually appealing, you can customize the buttons with icons. This method is particularly useful in applications where aesthetics matter, such as games or multimedia apps. Let’s see how to add icons to our radio buttons.
import javax.swing.*;
import java.awt.*;
public class ButtonGroupWithIcons {
public static void main(String[] args) {
JFrame frame = new JFrame("Button Group with Icons");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize(300, 200);
JPanel panel = new JPanel();
JRadioButton option1 = new JRadioButton("Option 1", new ImageIcon("icon1.png"));
JRadioButton option2 = new JRadioButton("Option 2", new ImageIcon("icon2.png"));
JRadioButton option3 = new JRadioButton("Option 3", new ImageIcon("icon3.png"));
ButtonGroup group = new ButtonGroup();
group.add(option1);
group.add(option2);
group.add(option3);
panel.add(option1);
panel.add(option2);
panel.add(option3);
frame.add(panel);
frame.setVisible(true);
}
}
Output:
In this code snippet, we replace the text of each JRadioButton
with an icon using the ImageIcon
class. You need to ensure that the image files (icon1.png, icon2.png, and icon3.png) are available in your project directory. This customization not only makes the buttons more attractive but also helps convey meaning through visual representation.
Conclusion
Creating button groups in Java is a straightforward process that can significantly enhance user interaction within your applications. By using the ButtonGroup
class along with JRadioButton
, you can easily implement exclusive selection functionality. Adding action listeners and customizing buttons with icons further enhances the user experience. Whether you’re building a simple form or a complex application, mastering button groups is a valuable skill for any Java developer.
FAQ
-
What is a button group in Java?
A button group in Java is a component that allows for exclusive selection among a set of buttons, typically usingJRadioButton
. -
How do I create a button group in Java?
You can create a button group using theButtonGroup
class and addingJRadioButton
instances to it. -
Can I add action listeners to button groups?
Yes, you can add action listeners to individual buttons in a button group to respond to user selections. -
How do I customize buttons in a button group?
You can customize buttons by setting icons using theImageIcon
class when creatingJRadioButton
instances. -
Are button groups only for radio buttons?
While button groups are commonly used with radio buttons, they can also work with checkboxes, though the behavior differs.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook