How to Create Java Progress Bar Using JProgressBar Class
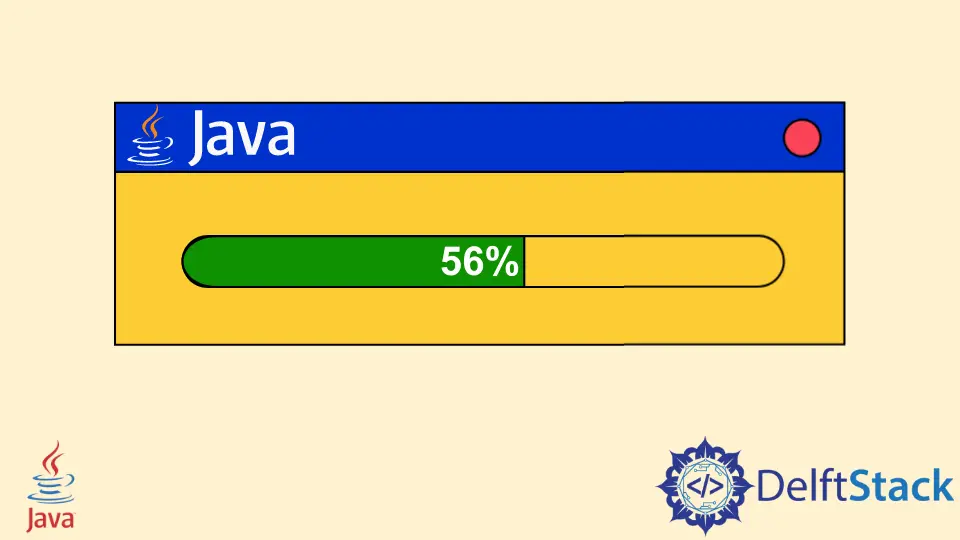
This tutorial aims to understand how we can create a Java progress bar using the JProgressBar
class in the SWING application. We’ll also learn how to display numbers and text considering the level to which the progress bar is filled.
Use JProgressBar
Class to Create Java Progress Bar
Suppose we upload/download a file, and the operation is being performed behind the scene without notifying us. We may sit for hours and let the machine accomplish the upload/download.
This is where the progress bar is helpful, and it tells how much work is done. Start with the horizontal progress bar using the JProgressBar
class, a widget showing a progress bar for time-consuming tasks.
Example Code:
import java.awt.*;
import javax.swing.*;
public class jProgressBarDemoOne {
public static void main(String[] args) {
final int MAXIMUM = 100;
final JFrame jFrame = new JFrame("JProgress Demo One");
// create horizontal progress bar
final JProgressBar progressBar = new JProgressBar();
progressBar.setMinimum(0);
progressBar.setMaximum(MAXIMUM);
progressBar.setStringPainted(true);
// add progress bar to the content pane layer
jFrame.setLayout(new FlowLayout());
jFrame.getContentPane().add(progressBar);
jFrame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
jFrame.setSize(300, 200);
jFrame.setVisible(true);
// update progressbar staring from 0 to 100
for (int i = 0; i <= MAXIMUM; i++) {
final int currentNumber = i;
try {
SwingUtilities.invokeLater(new Runnable() {
public void run() {
progressBar.setValue(currentNumber);
}
});
java.lang.Thread.sleep(100);
} catch (InterruptedException event) {
JOptionPane.showMessageDialog(jFrame, event.getMessage());
}
}
}
}
Output:
Inside the main
function, we assign 100
to the MAXIMUM
variable, which denotes the task completion if this number is visible on the progress bar.
The JFrame
constructor is created to show a window on the computer screen, and it is a base window for other components on which they rely, for instance, panels, buttons, menu bar etc.
Next, we create a construction of the JProgressBar
class to create a horizontal progress bar. The setMinimum()
and setMaximum()
methods take an integer as an argument used to set the minimum and maximum value stored in the progress bar’s data model.
The setStringPainted()
method takes a Boolean value to decide whether the progress bar should render a progress string or not. The string is rendered if it got true
; otherwise, not.
The setLayout()
method allows us to set the container’s layout as a FlowLayout
for this tutorial. The FlowLayout
adds the elements in a sequential flow (one element after the other in a line) in the container and moves the element to the next line if it can’t fit on the current line.
For now, we have created a window, set the container’s layout, and created a horizontal bar with minimum and maximum value, and it’s time to add the progress bar to the container.
We are using the getContentPane()
method, which is used to access the content pane layer and allow us to add the object to it.
When the user clicks the cross button (X
), the close window event is fired, allowing the setDefaultCloseOperation()
function to close the application. The setSize()
is used to set the width
and height
of the window while setvisible()
shows the window to the users if it gets true
.
Finally, the for
loops update the progress bar that starts from 0% and goes to 100%. The next example code will display text on the progress bar instead of numbers.
Example Code:
import java.awt.*;
import javax.swing.*;
public class jProgressBarDemoTwo {
static int MAXIMUM = 100;
static JFrame jFrame = new JFrame("JProgress Demo");
// create horizontal progress bar
static JProgressBar progressBar = new JProgressBar();
public static void main(String[] args) {
progressBar.setMinimum(0);
progressBar.setMaximum(MAXIMUM);
progressBar.setStringPainted(true);
// add progress bar to the content pane layer
jFrame.setLayout(new FlowLayout());
jFrame.getContentPane().add(progressBar);
jFrame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
jFrame.setSize(300, 200);
jFrame.setVisible(true);
// update progressbar by displaying text on it
int currentNumber = 0;
try {
while (currentNumber <= MAXIMUM) {
// set text considering the level to which the bar is colored/filled
if (currentNumber > 30 && currentNumber < 70)
progressBar.setString("wait for sometime");
else if (currentNumber > 70)
progressBar.setString("almost finished loading");
else
progressBar.setString("loading started");
// color/fill the menu bar
progressBar.setValue(currentNumber + 10);
// delay thread
Thread.sleep(3000);
currentNumber += 20;
}
} catch (Exception e) {
System.out.println(e);
}
}
}
Output::