How to Convert String to JSON Object in Java
-
Use
JSONObject
to Convert a String to JSON Object in Java - Use Google Gson to Convert a String to JSON Object in Java
-
Use
Jackson
to Convert a String to JSON Object
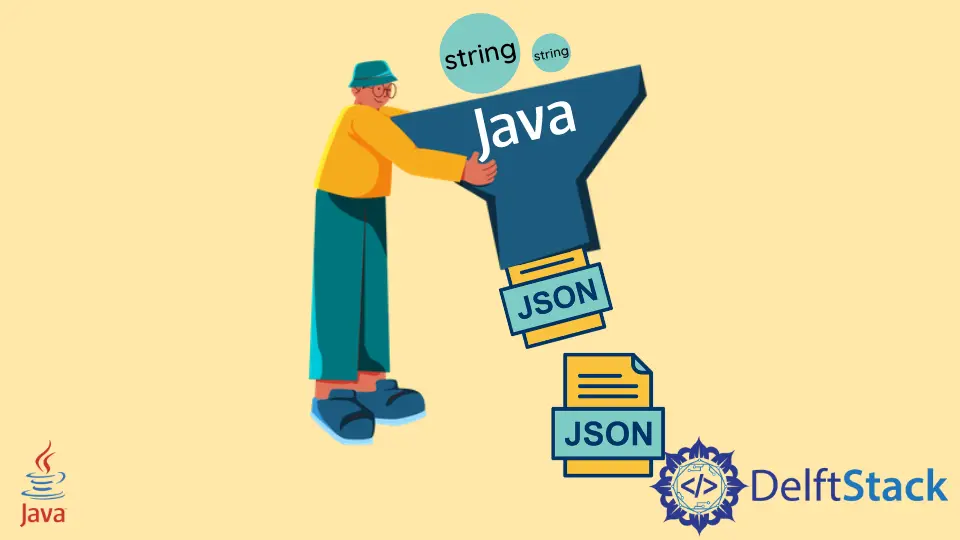
In this tutorial, we are going to discuss how to convert a string into a JSON object in Java. We have different libraries available to perform this task.
Use JSONObject
to Convert a String to JSON Object in Java
JSONObject
can parse a string into a map-like object. It stores unordered key-value pairs. JSON-java library, commonly known as org.json
, is used here with required maven dependency. The maven dependency which we used is given below.
<!-- https://mvnrepository.com/artifact/org.json/json -->
<dependency>
<groupId>org.json</groupId>
<artifactId>json</artifactId>
<version>20201115</version>
</dependency>
To parse a JSON string to JSONObject
, we pass the string to the constructor as shown below.
import org.json.JSONException;
import org.json.JSONObject;
public class StringToJsonObject {
public static void main(String[] args) {
try {
String str = "{\"name\":\"John\",\"age\":\"30\"}";
JSONObject jsonObject = new JSONObject(str);
System.out.println("OBJECT : " + jsonObject.toString());
} catch (JSONException err) {
System.out.println("Exception : " + err.toString());
}
}
}
Output:
OBJECT : {"age":"30","name":"John"}
Use Google Gson to Convert a String to JSON Object in Java
Google Gson is a java library to serialize/deserialize Java Objects to JSON or vice-versa. It can also be used to convert Java string to its equivalent JSON Object.
The maven dependency that is required for this library is given below.
<dependency>
<groupId>com.google.code.gson</groupId>
<artifactId>gson</artifactId>
<version>2.8.6</version>
</dependency>
In this code, we first create an instance of Gson using GsonBuilder by calling its create()
method. We can also enable various configuration settings on builder
. As shown below we use its setPrettyPrinting()
method. As the name suggests it pretty prints the JSON output.
Later we used the fromJson
method of Gson Object, which parses the JSON string to the User
object. The toJson()
method uses Gson to convert the User
object back to the JSON string. Thus string str
is converted into a JSON Object using the Gson library.
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonIOException;
public class StringToJsonObject {
public static void main(String[] args) {
try {
String str = "{\"name\":\"John\", \"age\":21 , \"place\":\"Nevada\"}";
GsonBuilder builder = new GsonBuilder();
builder.setPrettyPrinting();
Gson gson = builder.create();
User user = gson.fromJson(str, User.class);
System.out.println(user.ShowAsString());
str = gson.toJson(user);
System.out.println("User Object as string : " + str);
} catch (JsonIOException err) {
System.out.println("Exception : " + err.toString());
}
}
}
class User {
public String name;
public int age;
public String place;
public String ShowAsString() {
return "User [" + name + ", " + age + ", " + place + "]";
}
}
Output:
User [John, 21, Nevada]
User Object as string : {
"name": "John",
"age": 21,
"place": "Nevada"
}
Use Jackson
to Convert a String to JSON Object
Jackson is also known as the Java JSON library. The ObjectMapper
is used to map JSON into Java Objects or Java Objects into JSON. The maven dependency used for this library is shown below.
<!-- https://mvnrepository.com/artifact/com.fasterxml.jackson.core/jackson-databind -->
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-databind</artifactId>
<version>2.11.3</version>
</dependency>
The Jackson has a built-in tree model to represent JSON Object. JsonNode
is the class that represents the tree model. The ObjectMapper instance mapper
parses JSON into a JsonNode
tree model calling readTree()
.
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.ObjectMapper;
public class StringToJsonObject {
public static void main(String[] args) {
String json_str = "{\"name\":\"John\", \"age\":21 , \"place\":\"Nevada\"}";
ObjectMapper mapper = new ObjectMapper();
try {
JsonNode node = mapper.readTree(json_str);
String name = node.get("name").asText();
String place = node.get("age").asText();
System.out.println("node" + node);
System.out.println("name: " + name + ", place: " + place);
} catch (JsonProcessingException e) {
e.printStackTrace();
}
}
}
Output:
node{"name":"John","age":21,"place":"Nevada"}
name: John, place: 21
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedInRelated Article - Java String
- How to Perform String to String Array Conversion in Java
- How to Remove Substring From String in Java
- How to Convert Byte Array in Hex String in Java
- How to Convert Java String Into Byte
- How to Generate Random String in Java
- The Swap Method in Java