How to Create ArrayList From Array in Java
- What Is an Array in Java?
-
What Is
ArrayList
in Java? -
Difference Between Array and
ArrayList
in Java -
Conversion of an Array to
ArrayList
UsingArrays.asList()
-
Conversion of an Array to
ArrayList
UsingCollections.addAll()
-
Conversion of an Array to
ArrayList
Usingadd()
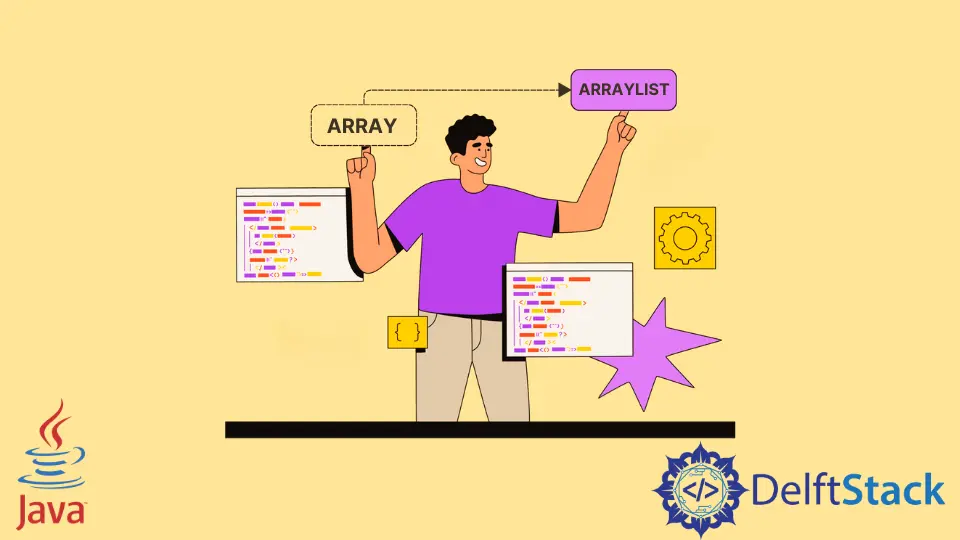
This tutorial article will introduce different ways to create ArrayList
from array in Java. There are three different methods to convert an array to ArrayList
in Java such as Arrays.asList()
, Collections.addAll()
and add()
.
Before proceeding with the demonstration, let us understand what is an array and ArrayList
and how they differ from each other.
What Is an Array in Java?
An array is a collection of a fixed number of similar types of data. For example, if we want to store the data of 50 books, we can create an array of the string type that can hold 50 books. After creation, the length of the array is fixed. An array is the basic built-in functionality of Java.
String[] array = new String[50];
What Is ArrayList
in Java?
The ArrayList
is a resizable array that stores a dynamic collection of elements found within the java.util
package.
Difference Between Array and ArrayList
in Java
The main difference between an array and ArrayList
is that the length of an array cannot be modified or extended. To add or remove elements to/from an array, we have to create a new list. Whereas, elements can be added or removed to/from ArrayList
at any point due to its resizable nature.
Conversion of an Array to ArrayList
Using Arrays.asList()
Using Arrays.asList()
, the array is passed to this method and a list
object is obtained, which is again passed to the constructor of the ArrayList
class as a parameter. The syntax of the Arrays.asList()
is as below:
ArrayList<T> arraylist = new ArrayList<T>(Arrays.asList(arrayname));
Let us follow the below example.
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
public class Method1 {
public static void main(String[] args) {
String[] subjects = {"maths", "english", "history", "physics"};
ArrayList<String> sublist = new ArrayList<String>(Arrays.asList(subjects));
sublist.add("geography");
sublist.add("chemistry");
for (String str : sublist) {
System.out.println(str);
}
}
}
Output:
maths
english
history
physics
geography
chemistry
Conversion of an Array to ArrayList
Using Collections.addAll()
This method lists all the array elements in a definite collection almost similar to Arrays.asList()
. However, Collections.addAll()
is much faster as compared to Arrays.asList()
method on performance basis. The syntax of Collections.addAll()
is as below:
Collections.addAll(arraylist, new Element(1), new Element(2), new Element(3), new Element(4));
Let us understand the below example.
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
public class Method2 {
public static void main(String[] args) {
String[] names = {"john", "emma", "rick", "tim"};
ArrayList<String> namelist = new ArrayList<String>();
Collections.addAll(namelist, names);
namelist.add("jenny");
namelist.add("rob");
for (String str : namelist) {
System.out.println(str);
}
}
}
Output:
john
emma
rick
tim
jenny
rob
Conversion of an Array to ArrayList
Using add()
Using this method, we can create a new list and add the list elements in a much simpler way. The syntax for the add()
method is as below:
arraylist.add(element);
Let us check the below example.
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
public class Method3 {
public static void main(String[] args) {
ArrayList<String> planetlist = new ArrayList<String>();
String[] planets = {"earth", "mars", "venus", "jupiter"};
for (int i = 0; i < planets.length; i++) {
planetlist.add(planets[i]);
}
for (String str : planetlist) {
System.out.println(str);
}
}
}
Output:
earth
mars
venus
jupiter
Following the above methods, we can now easily convert an array to ArrayList
.
Related Article - Java Array
- How to Concatenate Two Arrays in Java
- How to Convert Byte Array in Hex String in Java
- How to Remove Duplicates From Array in Java
- How to Count Repeated Elements in an Array in Java
- Natural Ordering in Java
- How to Slice an Array in Java