How to Convert an Arraylist to a String in Java
-
Convert
ArrayList
toString
With+
Operator in Java -
Convert
ArrayList
toString
Using theappend()
Method in Java -
Convert
ArrayList
toString
Using thejoin()
Method in Java -
Convert
ArrayList
toString
UsingStringUtils
Class in Java -
Convert
ArrayList
toString
Using thereplaceAll()
Method in Java -
Convert
ArrayList
toString
Using thejoining()
Method in Java -
Convert
ArrayList
toString
Using thedeepToString()
Method in Java
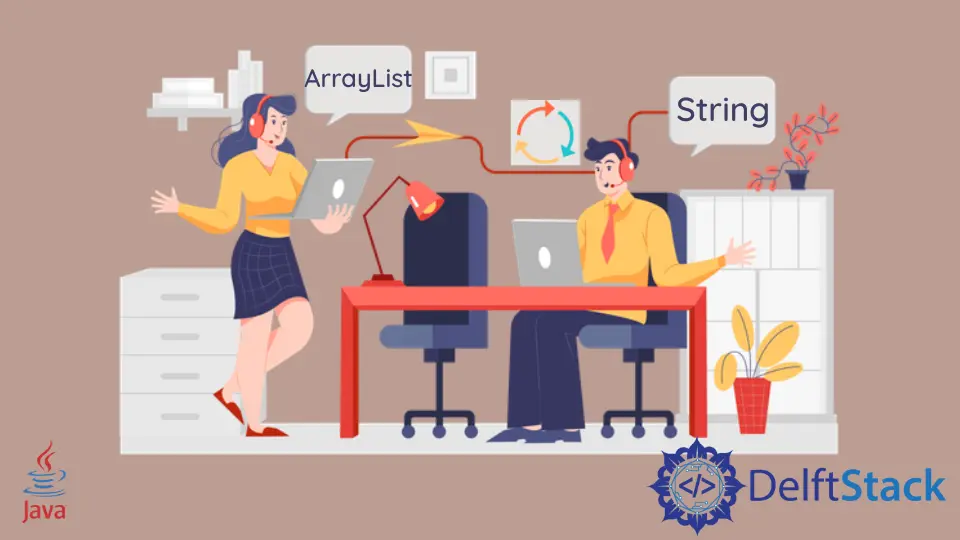
This tutorial introduces how to convert an ArrayList
to a string in Java and lists some example codes to understand the topic. There are several ways to convert ArrayList
like using join()
, append()
method, StringUtils
class, etc. Let’s take a close look at the examples.
Convert ArrayList
to String
With +
Operator in Java
The most straightforward and easy way to convert an ArrayList to String is to use the plus (+) operator. In String, the plus operator concatenates two string objects and returns a single object. Here, we are using the plus operator. See the example below.
import java.util.ArrayList;
import java.util.List;
public class SimpleTesting {
public static void main(String[] args) {
// ArrayList
List<String> fruits = new ArrayList<>();
fruits.add("Apple");
fruits.add("Orange");
fruits.add("Mango");
System.out.println(fruits);
String str = "";
for (String fruit : fruits) {
str += fruit + ",";
}
System.out.println(str);
}
}
Output:
[Apple, Orange, Mango]
Apple,Orange,Mango,
Convert ArrayList
to String
Using the append()
Method in Java
We can use the append()
method of StringBuilder
class to convert ArrayList
to string. This method returns a String object. See the example below.
import java.util.ArrayList;
import java.util.List;
public class SimpleTesting {
public static void main(String[] args) {
// ArrayList
List<String> fruits = new ArrayList<>();
fruits.add("Apple");
fruits.add("Orange");
fruits.add("Mango");
System.out.println(fruits);
StringBuilder str = new StringBuilder();
for (String fruit : fruits) {
str.append(fruit);
str.append(" ");
}
System.out.println(str);
}
}
Output:
[Apple, Orange, Mango]
Apple Orange Mango
Convert ArrayList
to String
Using the join()
Method in Java
We can use the join()
method of the String
class to join ArrayList
elements into a single string. This method returns a string and can be used to convert ArrayList to String in Java.
import java.util.ArrayList;
import java.util.List;
public class SimpleTesting {
public static void main(String[] args) {
// ArrayList
List<String> fruits = new ArrayList<>();
fruits.add("Apple");
fruits.add("Orange");
fruits.add("Mango");
System.out.println(fruits);
String str = String.join(",", fruits);
System.out.println(str);
}
}
Output:
[Apple, Orange, Mango]
Apple,Orange,Mango
Convert ArrayList
to String
Using StringUtils
Class in Java
If you are working with the Apache commons lang
library, then you can use the join()
method of StringUtils
class to get string object from the ArrayList
. Here, we use the join()
method that takes two arguments: ArrayList
and the separator symbol. See the example below.
import java.util.ArrayList;
import java.util.List;
import org.apache.commons.lang3.StringUtils;
public class SimpleTesting {
public static void main(String[] args) {
// ArrayList
List<String> fruits = new ArrayList<>();
fruits.add("Apple");
fruits.add("Orange");
fruits.add("Mango");
System.out.println(fruits);
String str = StringUtils.join(fruits, ", ");
System.out.println(str);
}
}
Output:
[Apple, Orange, Mango]
Apple, Orange, Mango
Convert ArrayList
to String
Using the replaceAll()
Method in Java
This is a little tricky approach to get the string from ArrayList
. In this way, we use the replaceAll()
method to replace square brackets of the List and then convert all elements into String
using the toString()
method. See the example below.
import java.util.ArrayList;
import java.util.List;
public class SimpleTesting {
public static void main(String[] args) {
// ArrayList
List<String> fruits = new ArrayList<>();
fruits.add("Apple");
fruits.add("Orange");
fruits.add("Mango");
System.out.println(fruits);
String str = fruits.toString().replaceAll("\\[|\\]", "").replaceAll(", ", ", ");
System.out.println(str);
}
}
Output:
[Apple, Orange, Mango]
Apple, Orange, Mango
Convert ArrayList
to String
Using the joining()
Method in Java
If you are using Java 8 or higher version, you can use the joining()
method of Collectors
class to collect all the elements into a single String
object. Here, we use the functional style of Java programming added into Java 8 version.
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
public class SimpleTesting {
public static void main(String[] args) {
// ArrayList
List<String> fruits = new ArrayList<>();
fruits.add("Apple");
fruits.add("Orange");
fruits.add("Mango");
System.out.println(fruits);
String str =
Arrays.asList(fruits).stream().map(Object::toString).collect(Collectors.joining(", "));
System.out.println(str);
}
}
Output:
[Apple, Orange, Mango]
[Apple, Orange, Mango]
Convert ArrayList
to String
Using the deepToString()
Method in Java
We can use the deepToString()
method of Arrays
class that converts all the elements of ArrayList
into a String
object. See the example below.
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
public class SimpleTesting {
public static void main(String[] args) {
// ArrayList
List<String> fruits = new ArrayList<>();
fruits.add("Apple");
fruits.add("Orange");
fruits.add("Mango");
System.out.println(fruits);
String str = Arrays.deepToString(fruits.toArray());
System.out.println(str);
}
}
Output:
[Apple, Orange, Mango]
[Apple, Orange, Mango]
Related Article - Java String
- How to Perform String to String Array Conversion in Java
- How to Remove Substring From String in Java
- How to Convert Byte Array in Hex String in Java
- How to Convert Java String Into Byte
- How to Generate Random String in Java
- The Swap Method in Java