How to Convert Int Array to Arraylist in Java
-
Convert an
int
Array to ArrayList Using Java 8 Stream -
Convert an
int
Array to an ArrayList Using an Enhancedfor
Loop in Java -
Convert an
int
Array to a List of Integer Objects UsingGuava
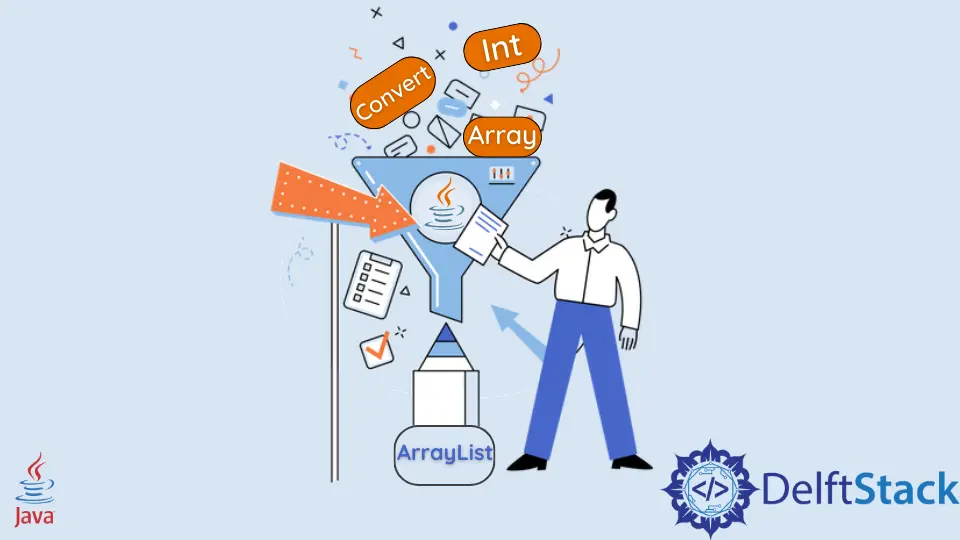
This tutorial introduces how we can convert an array of primitive int
to an ArrayList
in Java. We cannot create an ArrayList
of primitive data types so that we will use the Integer
object.
Convert an int
Array to ArrayList Using Java 8 Stream
This example uses the Stream
API of the Arrays
class that provides several methods to manipulate an array. For this to work, we first create an array of int
elements and use the Arrays
class to call the stream()
method. But as the items of intArray
are of primitive types, we have to use the boxed()
to box each primitive to an Integer
object.
The collect()
method collects the items and Collectors.toList()
converts them into a list. We can cast the returned list to ArrayList<Integer>
.
import java.util.ArrayList;
import java.util.Arrays;
import java.util.stream.Collectors;
public class IntToInteger {
public static void main(String[] args) {
int[] intArray = {10, 20, 30, 40};
ArrayList<Integer> integerArray =
(ArrayList<Integer>) Arrays.stream(intArray).boxed().collect(Collectors.toList());
System.out.println(integerArray);
}
}
Output:
[10, 20, 30, 40]
Convert an int
Array to an ArrayList Using an Enhanced for
Loop in Java
We can use the manual method to add every item of the array to the ArrayList. This method does not use any function, and instead, an enhanced for
loop is enough for this to work. We create an array intArray
with a few int
type elements and an empty ArrayList with the initial size equal to the size of intArray
.
Now, as the ArrayList has precisely the capacity to hold the elements of intArray
, we can use the enhanced for
loop and call the add()
method that adds an item into the ArrayList. The for
loop will iterate through the array, and every item will be added to the ArrayList.
We can see in the output that we get the same elements as intArray
.
import java.util.ArrayList;
public class IntToInteger {
public static void main(String[] args) {
int[] intArray = {13, 17, 21, 23};
ArrayList<Integer> integerArray = new ArrayList<>(intArray.length);
for (int i : intArray) {
integerArray.add(i);
}
System.out.println(integerArray);
}
}
Output:
[13, 17, 21, 23]
Convert an int
Array to a List of Integer Objects Using Guava
In this example, we use the Ints
class included in the Guava
library. To use the library functions, we include its maven dependency to the project.
<dependency>
<groupId>com.google.guava</groupId>
<artifactId>guava</artifactId>
<version>23.5-jre</version>
</dependency>
intArray
is an array with elements of the int
type. We use the Ints
class to call asList()
and pass the array as its argument. Ints.asList(intArray)
returns a list of Integer
objects.
import com.google.common.primitives.Ints;
import java.util.List;
public class IntToInteger {
public static void main(String[] args) {
int[] intArray = {13, 17, 21, 23};
List<Integer> integerArray = Ints.asList(intArray);
System.out.println(integerArray);
}
}
Output:
[13, 17, 21, 23]
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedInRelated Article - Java Array
- How to Concatenate Two Arrays in Java
- How to Convert Byte Array in Hex String in Java
- How to Remove Duplicates From Array in Java
- How to Count Repeated Elements in an Array in Java
- Natural Ordering in Java
- How to Slice an Array in Java