How to Convert Map Values Into a List in Java
- Define a Map to Be Converted Into a List in Java
-
Use
Collector
Streams to Convert a Map Into a List in Java
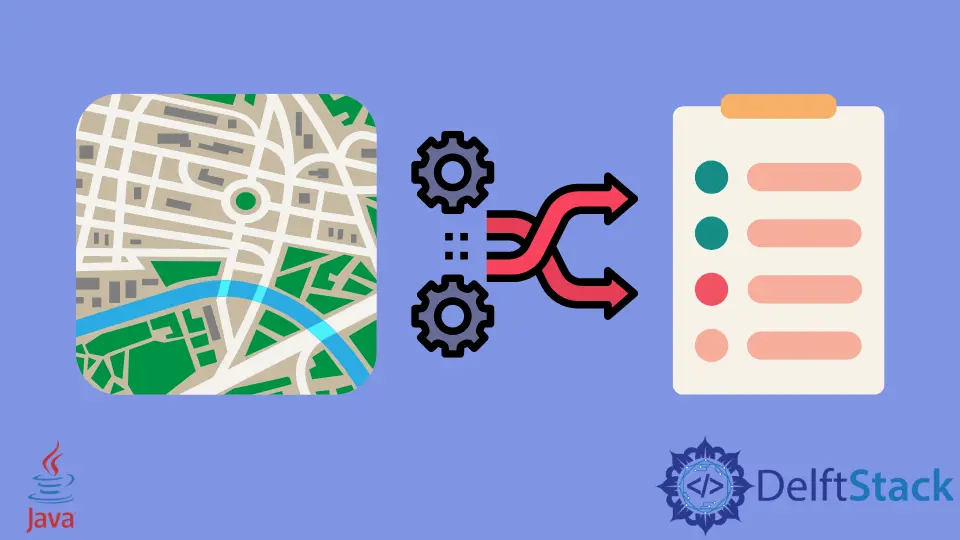
This tutorial will execute three programs to show how to convert hash map values into a list in Java.
Define a Map to Be Converted Into a List in Java
Before converting map values into a list, we must first have a map object and then assign it with two data types: an integer (keys) and string (values) before grouping it into a list.
The keys in this program are the cars’ RPM values, whereas the strings are their colors.
Syntax:
Map<Integer, String> M2L = new HashMap<>();
M2L.put(5000, "Toyata Black");
M2L.put(6000, "Audi White");
M2L.put(8000, "BMW Red");
M2L.put(12000, "Buggati Silver");
Use Collector
Streams to Convert a Map Into a List in Java
Collectors
are public classes that extend objects in Java. They also help collect elements and summarise features based on various benchmarks that users predefine.
Here, we use the keySet()
method to get keys by creating an array list from a set returned by a map.
Check out the following example, which converts a map into a list.
Example 1:
package maptolist;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.stream.Collectors;
public class MapToList {
public static void main(String[] args) {
Map<Integer, String> M2L = new HashMap<>();
M2L.put(1, "New York");
M2L.put(2, "Toronto");
M2L.put(3, "Berlin");
List<Integer> ID = M2L.keySet().stream().collect(Collectors.toList());
ID.forEach(System.out::println);
List<String> NAME = M2L.values().stream().collect(Collectors.toList());
NAME.forEach(System.out::println);
}
}
Output:
1
2
3
New York
Toronto
Berlin
Consider the following code in our second example.
Syntax:
List<Integer> nums = maptolist.keySet().stream().collect(Collectors.toList());
Example 2:
package maptolist;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.stream.Collectors;
public class MaptoListExample2 {
public static void main(String[] args) {
Map<Integer, String> maptolist = new HashMap<>();
maptolist.put(1, "This");
maptolist.put(2, "Is");
maptolist.put(3, "A");
maptolist.put(4, "Demo");
maptolist.put(5, "Example");
List<Integer> nums = maptolist.keySet().stream().collect(Collectors.toList());
System.out.println("Keys:");
nums.forEach(System.out::println);
// Conversion Strings
List<String> chars = maptolist.values().stream().collect(Collectors.toList());
System.out.println("Values:");
chars.forEach(System.out::println);
}
}
Output:
Keys:
1
2
3
4
5
Values:
This
Is
A
Demo
Example
Now that we’ve seen the underlying logic behind maps and lists and used a combination of object collectors, below is a solid map-into-list program that will work in every situation.
The keySet()
function returns a set view of keys in the map so that map modifications also apply to the set. Here, we form a pair within a wrap to obtain a list of keys, as shown below.
Syntax:
List<String> setkey = new ArrayList<String>(MAP2LIST.keySet());
Example 3:
package maptolist;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class MapToListExample3 {
public static void main(String[] args) {
// Define Map
Map<String, String> MAP2LIST = new HashMap<String, String>();
// Values (key as values)
MAP2LIST.put("1 + 1", "TWO");
MAP2LIST.put("10 - 5", "FIVE");
MAP2LIST.put("2.4 + 3.6", "SIX");
// Define list
List<String> setkey = new ArrayList<String>(MAP2LIST.keySet());
// foreach function extends setkey and prints the output
setkey.forEach(System.out::println);
List<String> setvalue = new ArrayList<String>(MAP2LIST.values());
setvalue.forEach(System.out::println);
}
}
Output:
10 - 5
1 + 1
2.4 + 3.6
FIVE
TWO
SIX
Sarwan Soomro is a freelance software engineer and an expert technical writer who loves writing and coding. He has 5 years of web development and 3 years of professional writing experience, and an MSs in computer science. In addition, he has numerous professional qualifications in the cloud, database, desktop, and online technologies. And has developed multi-technology programming guides for beginners and published many tech articles.
LinkedInRelated Article - Java List
- How to Split a List Into Chunks in Java
- How to Get First Element From List in Java
- How to Find the Index of an Element in a List Using Java
- How to Filter List in Java
- Differences Between List and Arraylist in Java
- List vs. Array in Java