How to Create Map in Java
-
Creating
Map
UsingHashMap
in Java -
Creating
Map
UsingMap.ofEntries
in Java -
Creating
Map
Along With Initialization in Java -
Creating
Map
Using theMap.of()
Method
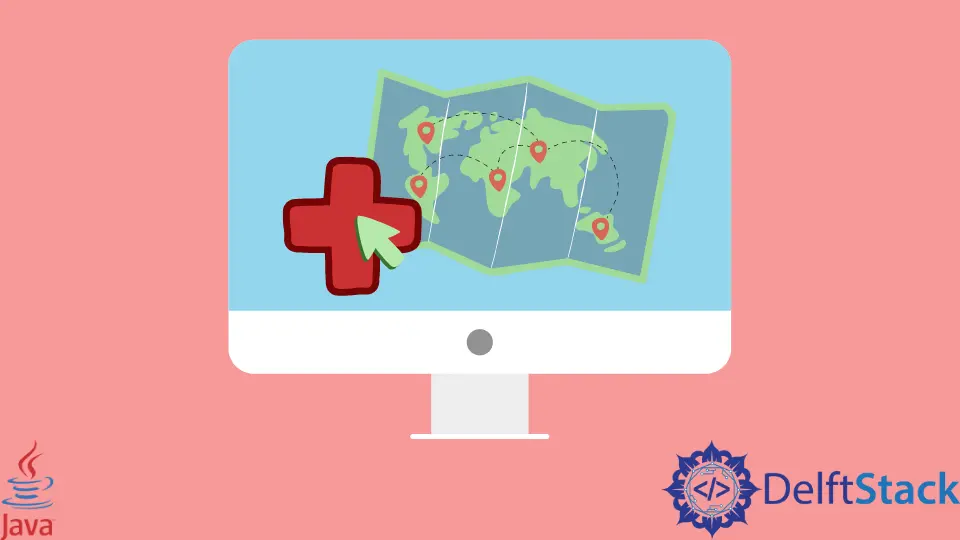
This tutorial introduces how to create Map in Java and lists some example codes to understand the topic.
Map
is an interface in Java and belongs to java.util
package. It is used to store data in key-value pairs. It provides several implementation classes such as HashMap
, LinkedHashMap
, TreeMap
, etc.
We can create Map by using these classes and then hold a reference to Map. Let’s understand by some examples.
Creating Map
Using HashMap
in Java
Let’s create a Map
that holds the integer key and String
values. See, we used the HashMap class and inserted elements by using the put()
method. See the example below.
package javaexample;
import java.util.HashMap;
import java.util.Map;
public class SimpleTesting {
public static void main(String[] args) {
Map<Integer, String> hm = new HashMap<Integer, String>();
hm.put(1, "Red");
hm.put(2, "Green");
hm.put(3, "Blue");
hm.put(4, "White");
System.out.println(hm);
}
}
Output:
{1=Red, 2=Green, 3=Blue, 4=White}
Creating Map
Using Map.ofEntries
in Java
It is a static method of Map interface and was added into Java 9. We can use it to create an immutable map containing keys and values extracted from the given entries. See the example below.
import java.util.Map;
public class SimpleTesting {
public static void main(String[] args) {
Map<Integer, String> map =
Map.ofEntries(Map.entry(1, "Red"), Map.entry(2, "Green"), Map.entry(3, "Blue"));
System.out.println(map);
}
}
Output:
{2=Green, 3=Blue, 1=Red}
Creating Map
Along With Initialization in Java
Java allows initializing a Map at the time of creating and declaration. It is a concise way to create Map in Java. This is another approach we can use to create Map in Java. See the example below.
import java.util.HashMap;
import java.util.Map;
public class SimpleTesting {
public static void main(String[] args) {
Map<Integer, String> map = new HashMap<Integer, String>() {
{
put(1, "Red");
put(2, "Green");
put(3, "Blue");
put(4, "White");
}
};
System.out.println(map);
}
}
Output:
{1=Red, 2=Green, 3=Blue, 4=White}
Creating Map
Using the Map.of()
Method
The Map.of()
method was added to the Map
interface in Java 9. We can use it to create Map in Java. It returns an immutable map containing zero mappings. See the example below.
import java.util.Map;
public class SimpleTesting {
public static void main(String[] args) {
Map map = Map.of(1, "Red", 2, "Green", 3, "Three", 4, "White");
System.out.println(map);
}
}
Output:
{1=Red, 2=Green, 3=Blue, 4=White}