How to Filter A Value From Map in Java
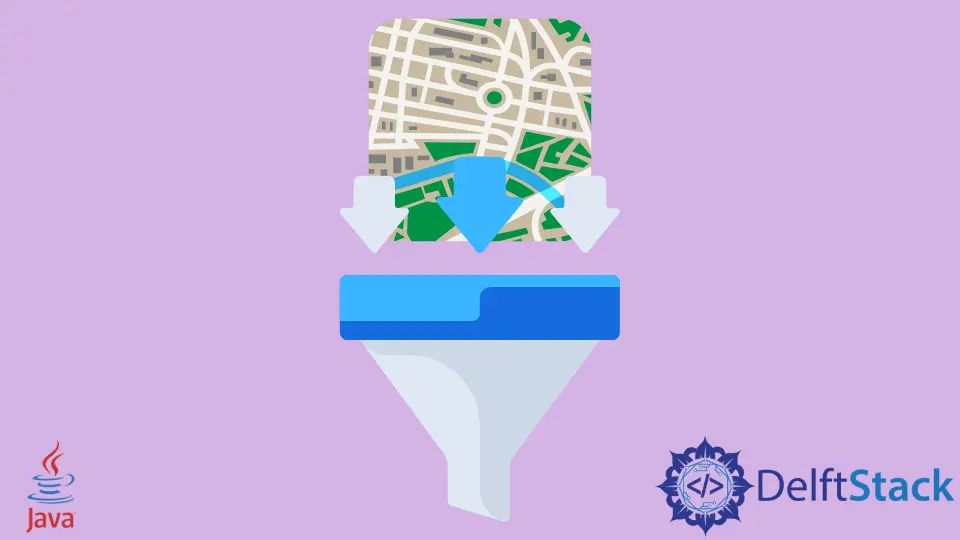
We will learn how to filter map values in the Java programming language. There are two methods through which you can do this task. Let’s take a look at them.
Map Filtering in Java
The two methods we can use to filter a map are entrySet()
and getKey()
. In the first method, entrySet()
, we filter the map using values.
We use the complete key-value pair in the second method, getKey()
. The methods are somewhat complex and involve multiple conversions.
entrySet()
The entryset()
method returns a value. We can insert a value and check whether that value is present in a Map or not. Look at the following code.
import java.util.HashMap;
import java.util.Map;
import java.util.stream.Collectors;
public class Main {
public static void main(String[] args) {
String result = "";
Map<Integer, String> Country = new HashMap<>();
Country.put(1, "Canada"); // Inserting Value
Country.put(2, "UnitedStates"); // Inserting Value
Country.put(3, "UnitedKingdom"); // Inserting Value
Country.put(4, "Argentina"); // Inserting Value
// Map -> Stream -> Filter -> String //Filter Using Map Value
result = Country.entrySet()
.stream()
.filter(map -> "Argentina".equals(map.getValue()))
.map(map -> map.getValue())
.collect(Collectors.joining());
System.out.println("Filtered Value Is ::" + result);
}
}
Output:
Filtered Value Is ::Argentina
The code line that filters a value is pretty long. As mentioned above, we convert values from a Map
into a Stream
. Then, we filter that stream and store the filtered value inside a string - the stream
method converts set to stream. The filter
method will filter the value from the map.
getKey()
The getKey()
method returns the complete key-value pair. Instead of matching a value, we take out the value using its key. The complete filtered key-value pair is stored inside another map then later on printed. Look at the following code.
import java.util.HashMap;
import java.util.Map;
import java.util.stream.Collectors;
public class Main {
public static void main(String[] args) {
String result = "";
Map<Integer, String> Country = new HashMap<>();
Country.put(1, "Canada"); // Inseting Value
Country.put(2, "UnitedStates"); // Inserting Value
Country.put(3, "UnitedKingdom"); // Inserting Value
Country.put(4, "Argentina"); // Inserting Value
// Filter Using Map Value
Map<Integer, String> pair =
Country.entrySet()
.stream()
.filter(map -> map.getKey().intValue() == 1)
.collect(Collectors.toMap(map -> map.getKey(), map -> map.getValue()));
System.out.println("Pair Is : " + pair);
}
}
Output:
Pair Is : {1=Canada}
To have a concrete understanding of the concepts, you should visit the following links.
Learn more about Streams here and learn more about Maps here.
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn