Java 中的對映過濾
Haider Ali
2023年10月12日
Java
Java Map
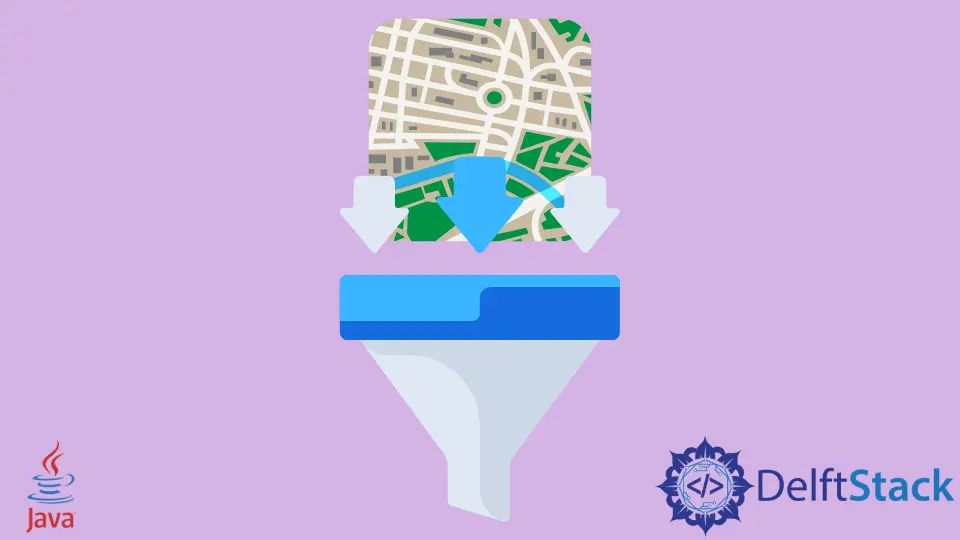
我們將學習如何在 Java 程式語言中過濾對映值。有兩種方法可以完成此任務。讓我們來看看它們。
Java 中的對映過濾
我們可以用來過濾 map 的兩種方法是 entrySet()
和 getKey()
。在第一個方法 entrySet()
中,我們使用值過濾 map。
我們在第二種方法 getKey()
中使用完整的鍵值對。這些方法有些複雜,涉及多次轉換。
entrySet()
entryset()
方法返回一個值。我們可以插入一個值並檢查該值是否存在於 Map 中。看下面的程式碼。
import java.util.HashMap;
import java.util.Map;
import java.util.stream.Collectors;
public class Main {
public static void main(String[] args) {
String result = "";
Map<Integer, String> Country = new HashMap<>();
Country.put(1, "Canada"); // Inserting Value
Country.put(2, "UnitedStates"); // Inserting Value
Country.put(3, "UnitedKingdom"); // Inserting Value
Country.put(4, "Argentina"); // Inserting Value
// Map -> Stream -> Filter -> String //Filter Using Map Value
result = Country.entrySet()
.stream()
.filter(map -> "Argentina".equals(map.getValue()))
.map(map -> map.getValue())
.collect(Collectors.joining());
System.out.println("Filtered Value Is ::" + result);
}
}
輸出:
Filtered Value Is ::Argentina
過濾值的程式碼行很長。如上所述,我們將值從 Map
轉換為 Stream
。然後,我們過濾該流並將過濾後的值儲存在一個字串中 - stream
方法將 set 轉換為流。filter
方法將從 map 中過濾值。
getKey()
getKey()
方法返回完整的鍵值對。我們不是匹配一個值,而是使用它的鍵取出值。完整的過濾鍵值對儲存在另一個對映中,然後列印。看下面的程式碼。
import java.util.HashMap;
import java.util.Map;
import java.util.stream.Collectors;
public class Main {
public static void main(String[] args) {
String result = "";
Map<Integer, String> Country = new HashMap<>();
Country.put(1, "Canada"); // Inseting Value
Country.put(2, "UnitedStates"); // Inserting Value
Country.put(3, "UnitedKingdom"); // Inserting Value
Country.put(4, "Argentina"); // Inserting Value
// Filter Using Map Value
Map<Integer, String> pair =
Country.entrySet()
.stream()
.filter(map -> map.getKey().intValue() == 1)
.collect(Collectors.toMap(map -> map.getKey(), map -> map.getValue()));
System.out.println("Pair Is : " + pair);
}
}
輸出:
Pair Is : {1=Canada}
要具體瞭解這些概念,你應該訪問以下連結。
在此處瞭解有關 Streams 的更多資訊,並在此處瞭解有關 map 的更多資訊。
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: Haider Ali
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn