Java 中的映射过滤
Haider Ali
2023年10月12日
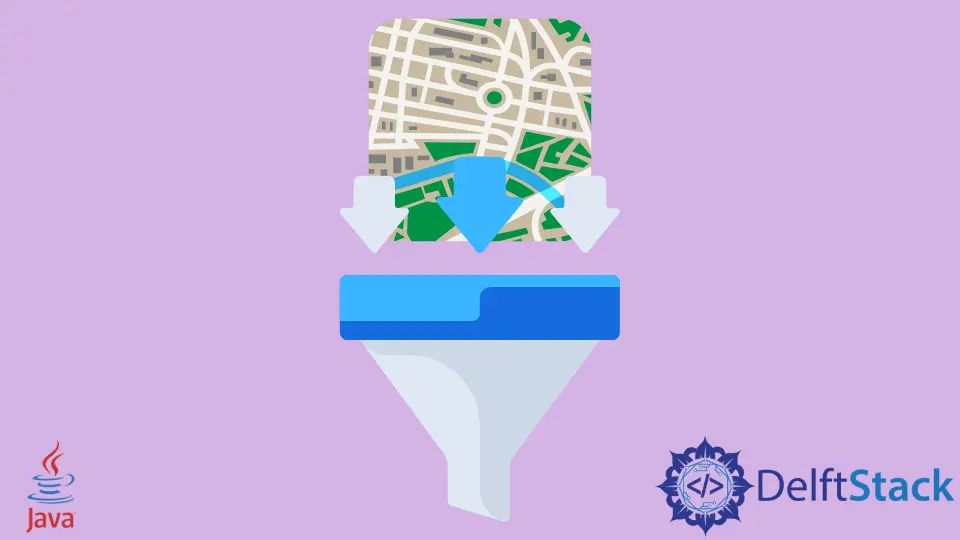
我们将学习如何在 Java 编程语言中过滤映射值。有两种方法可以完成此任务。让我们来看看它们。
Java 中的映射过滤
我们可以用来过滤 map 的两种方法是 entrySet()
和 getKey()
。在第一个方法 entrySet()
中,我们使用值过滤 map。
我们在第二种方法 getKey()
中使用完整的键值对。这些方法有些复杂,涉及多次转换。
entrySet()
entryset()
方法返回一个值。我们可以插入一个值并检查该值是否存在于 Map 中。看下面的代码。
import java.util.HashMap;
import java.util.Map;
import java.util.stream.Collectors;
public class Main {
public static void main(String[] args) {
String result = "";
Map<Integer, String> Country = new HashMap<>();
Country.put(1, "Canada"); // Inserting Value
Country.put(2, "UnitedStates"); // Inserting Value
Country.put(3, "UnitedKingdom"); // Inserting Value
Country.put(4, "Argentina"); // Inserting Value
// Map -> Stream -> Filter -> String //Filter Using Map Value
result = Country.entrySet()
.stream()
.filter(map -> "Argentina".equals(map.getValue()))
.map(map -> map.getValue())
.collect(Collectors.joining());
System.out.println("Filtered Value Is ::" + result);
}
}
输出:
Filtered Value Is ::Argentina
过滤值的代码行很长。如上所述,我们将值从 Map
转换为 Stream
。然后,我们过滤该流并将过滤后的值存储在一个字符串中 - stream
方法将 set 转换为流。filter
方法将从 map 中过滤值。
getKey()
getKey()
方法返回完整的键值对。我们不是匹配一个值,而是使用它的键取出值。完整的过滤键值对存储在另一个映射中,然后打印。看下面的代码。
import java.util.HashMap;
import java.util.Map;
import java.util.stream.Collectors;
public class Main {
public static void main(String[] args) {
String result = "";
Map<Integer, String> Country = new HashMap<>();
Country.put(1, "Canada"); // Inseting Value
Country.put(2, "UnitedStates"); // Inserting Value
Country.put(3, "UnitedKingdom"); // Inserting Value
Country.put(4, "Argentina"); // Inserting Value
// Filter Using Map Value
Map<Integer, String> pair =
Country.entrySet()
.stream()
.filter(map -> map.getKey().intValue() == 1)
.collect(Collectors.toMap(map -> map.getKey(), map -> map.getValue()));
System.out.println("Pair Is : " + pair);
}
}
输出:
Pair Is : {1=Canada}
要具体了解这些概念,你应该访问以下链接。
作者: Haider Ali
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn