在 Java 中将 Stream 元素转换为映射
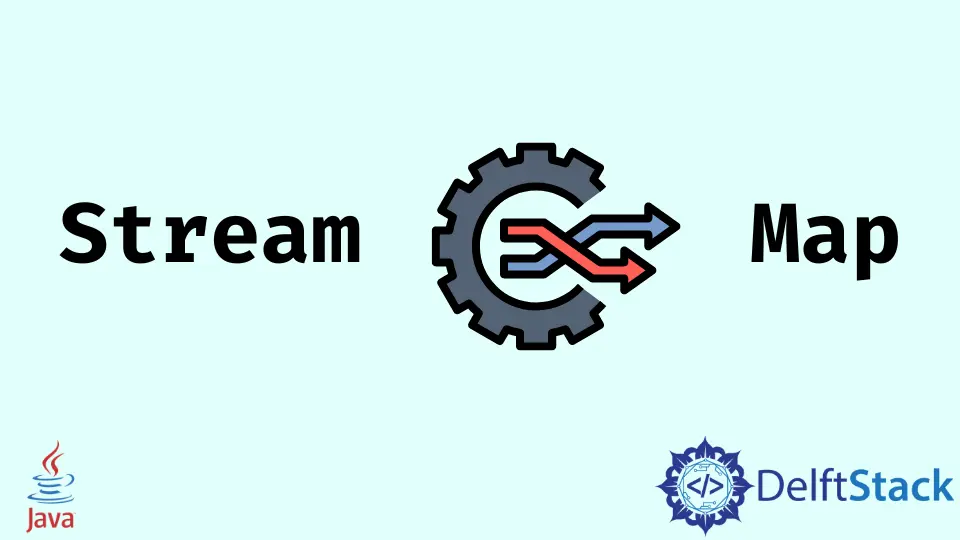
我们将讨论 Java Streams
的实际用途以及如何将流元素转换为映射元素。
要理解本文,你必须对 Java 8 有基本的了解,尤其是 lambda 表达式和 Stream
API。但是,如果你是新手,请不要担心;我们将解释一切。
Java 中要映射的字符串流
你一定想知道字符串本质上是一个元素列表,而不是键或值的列表,不是吗?要将字符串流转换为映射,你必须了解以下方法的基础知识。
Collectors.toMap()
- 它执行不同的功能归约操作,例如将元素收集到集合中。toMap
- 它返回一个Collector
,它将元素收集到一个带有键和值的Map
中。
Map<String, String> GMS = stream.collect(Collectors.toMap(k -> k[0], k -> k[1]));
知道了?我们将指定适当的映射函数来定义如何从流元素中检索键和值。
Example1.java
的实现:
package delftstackStreamToMapJava;
import java.util.Map;
import java.util.stream.Collectors;
import java.util.stream.Stream;
public class Example1 {
// Method to get stream of `String[]`
private static Stream<String[]> MapStringsStream() {
return Stream.of(new String[][] {{"Sky", "Earth"}, {"Fire", "Water"}, {"White", "Black"},
{"Ocean", "Deep"}, {"Life", "Death"}, {"Love", "Fear"}});
}
// Program to convert the stream to a map in Java 8 and above
public static void main(String[] args) {
// get a stream of `String[]`
Stream<String[]> stream = MapStringsStream();
// construct a new map from the stream
Map<String, String> GMS = stream.collect(Collectors.toMap(k -> k[0], k -> k[1]));
System.out.println(GMS);
}
}
输出:
{Sky=Earth, White=Black, Love=Fear, Fire=Water, Life=Death, Ocean=Deep}
从 Java 中的 Stream 对象创建 Map
该程序是使用 Collectors.toMap()
方法的另一个简单演示。
此外,它还使用[引用函数/lambda 表达式]。因此,我们建议你检查几分钟。
另外,如果你以前没有使用过 [Function.identity()
],请阅读它。
- 查看我们的三个流:
``java
List<Double> L1 = Arrays.asList(1.1,2.3)。
List<String> L2 = Arrays.asList("A", "B", "C");
List<Integer> L3 = Arrays.asList(10,20,30);
```
我们将三个数组列表定义为具有不同数据类型的流。
-
为我们的主要映射方法添加更多内容:
2.1 从Stream
对象生成Map
需要一个键和值映射器。
2.2 在这种情况下,Stream
是我们的数组列表,然后将其传递给Collectors
进行归约,同时借助.toMap
映射到键中。Map<Double, Object> printL1 = L1.stream() .collect(Collectors.toMap(Function.identity(), String::valueOf, (k1, k2) -> k1)); System.out.println("Stream of Double to Map: " + printL1);
Example2.java
的实现:
package delftstackStreamToMapJava;
import java.util.Arrays;
import java.util.List;
import java.util.Map;
import java.util.function.Function;
import java.util.stream.Collectors;
<!--undrads4-->
public class Example2 {
public static void main(String[] args) {
// Stream of Integers
List<Double> L1 = Arrays.asList(1.1, 2.3);
List<String> L2 = Arrays.asList("A", "B", "C");
List<Integer> L3 = Arrays.asList(10, 20, 30);
Map<Double, Object> printL1 =
L1.stream().collect(Collectors.toMap(Function.identity(), String::valueOf, (k1, k2) -> k1));
System.out.println("Stream of Double to Map: " + printL1);
Map<String, Object> printL2 =
L2.stream().collect(Collectors.toMap(Function.identity(), String::valueOf, (k1, k2) -> k1));
System.out.println("Stream of String to Map: " + printL2);
Map<Integer, Object> printL3 =
L3.stream().collect(Collectors.toMap(Function.identity(), String::valueOf, (k1, k2) -> k1));
System.out.println("Stream of Integers to Map: " + printL3);
}
}
输出:
Stream of Double to Map: {2.3=2.3, 1.1=1.1}
Stream of String to Map: {A=A, B=B, C=C}
Stream of Integers to Map: {20=20, 10=10, 30=30}
在 Java 中将 Stream 转换为 Map 时确定值的长度
以下代码块将字符串转换为 Map
,其中键表示字符串值,值表示每个单词的长度。这是我们的最后一个示例,尽管这属于映射基本示例的基本流的类别。
解释:
Collectors.toMap
收集器接受两个 lambda 函数作为参数。
stream-> stream
- 确定Stream
值并将其作为映射的键返回。stream-> stream.length
- 找到当前流值,找到它的长度并将其返回给给定键的映射。
Example3.java
的实现:
package delftstackStreamToMapJava;
<!--undrads5-->
import java.util.Arrays;
import java.util.Map;
import java.util.stream.Collectors;
public class Example3 {
public static Map<String, Integer> toMap(String str) {
Map<String, Integer> elementL =
Arrays.stream(str.split("/"))
.collect(Collectors.toMap(stream -> stream, stream -> stream.length()));
return elementL;
}
public static void main(String[] args) {
String stream = "We/Will/Convert/Stream/Elements/To/Map";
System.out.println(toMap(stream));
}
}
输出:
{Convert=7, Stream=6, Will=4, To=2, Elements=8, Map=3, We=2}
在 Java 中为唯一的产品键将流转换为映射
我们将转换整数流 pro_id
和字符串的 productname
。不过,不要混淆!
这是流集合映射值的又一个实现。
它是顺序的,这里的键是唯一的 pro_id
。整个程序除概念外与示例二相同。
这是使用流映射更现实的情况。它还将使你了解可以构建一个从数组对象中提取唯一值和键的自定义程序有多少种方法。
Products.java
的实现:
package delftstackStreamToMapJava;
<!--undrads6-->
import java.util.Arrays;
import java.util.List;
import java.util.Map;
import java.util.function.Function;
import java.util.stream.Collectors;
public class Products {
private Integer pro_id;
private String productname;
public Products(Integer pro_id, String productname) {
this.pro_id = pro_id;
this.productname = productname;
}
public Integer extractpro_id() {
return pro_id;
}
public String getproductname() {
return productname;
}
public String toString() {
return "[productid = " + this.extractpro_id() + ", productname = " + this.getproductname()
+ "]";
}
<!--undrads7-->
public static void main(String args[]) {
List<Products> listofproducts = Arrays.asList(new Products(1, "Lamda 2.0 "),
new Products(2, "Gerrio 3A ultra"), new Products(3, "Maxia Pro"),
new Products(4, "Lemna A32"), new Products(5, "Xoxo Pro"));
Map<Integer, Products> map = listofproducts.stream().collect(
Collectors.toMap(Products::extractpro_id, Function.identity()));
map.forEach((key, value) -> { System.out.println(value); });
}
}
输出:
[productid = 1, productname = Lamda 2.0 ]
[productid = 2, productname = Gerrio 3A ultra]
[productid = 3, productname = Maxia Pro]
[productid = 4, productname = Lemna A32]
[productid = 5, productname = Xoxo Pro]
IllegalArgumentException
。Sarwan Soomro is a freelance software engineer and an expert technical writer who loves writing and coding. He has 5 years of web development and 3 years of professional writing experience, and an MSs in computer science. In addition, he has numerous professional qualifications in the cloud, database, desktop, and online technologies. And has developed multi-technology programming guides for beginners and published many tech articles.
LinkedIn