在 Java 中将 map 值转换为列表
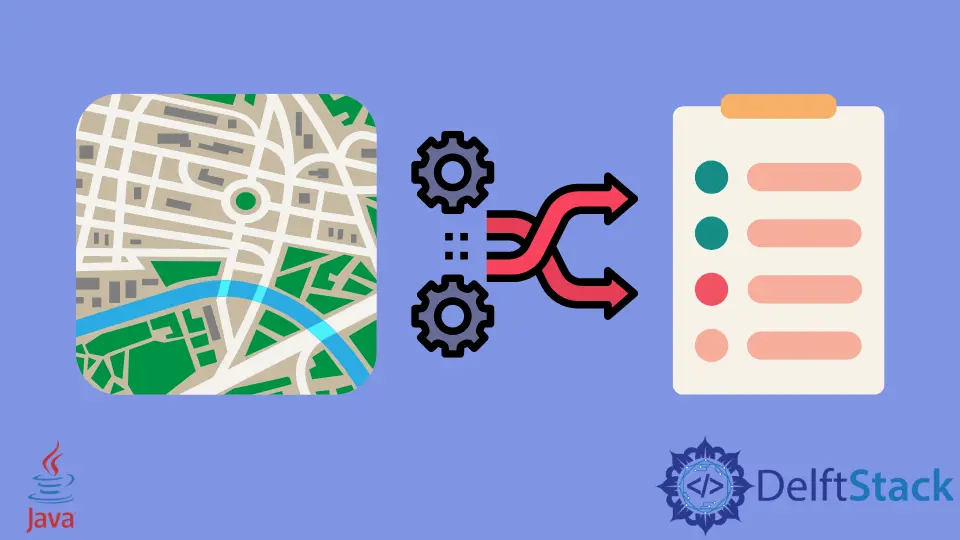
本教程将执行三个程序来展示如何将 Hashmap 值转换为 Java 中的列表。
在 Java 中定义要转换为列表的 map
在将 map 值转换为列表之前,我们必须先拥有一个 map 对象,然后为其分配两种数据类型:整数(键)和字符串(值),然后再将其分组为列表。
该程序中的键是汽车的 RPM 值,而字符串是它们的颜色。
语法:
Map<Integer, String> M2L = new HashMap<>();
M2L.put(5000, "Toyata Black");
M2L.put(6000, "Audi White");
M2L.put(8000, "BMW Red");
M2L.put(12000, "Buggati Silver");
在 Java 中使用 Collectors
流将 map 转换为列表
Collectors
是在 Java 中扩展对象的公共类。它们还帮助收集元素并根据用户预定义的各种基准来总结特征。
在这里,我们使用 keySet()
方法通过从 map 返回的集合创建数组列表来获取键。
查看以下示例,该示例将 map 转换为列表。
示例 1:
package maptolist;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.stream.Collectors;
public class MapToList {
public static void main(String[] args) {
Map<Integer, String> M2L = new HashMap<>();
M2L.put(1, "New York");
M2L.put(2, "Toronto");
M2L.put(3, "Berlin");
List<Integer> ID = M2L.keySet().stream().collect(Collectors.toList());
ID.forEach(System.out::println);
List<String> NAME = M2L.values().stream().collect(Collectors.toList());
NAME.forEach(System.out::println);
}
}
输出:
1
2
3
New York
Toronto
Berlin
在我们的第二个示例中考虑以下代码。
语法:
List<Integer> nums = maptolist.keySet().stream().collect(Collectors.toList());
示例 2:
package maptolist;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.stream.Collectors;
public class MaptoListExample2 {
public static void main(String[] args) {
Map<Integer, String> maptolist = new HashMap<>();
maptolist.put(1, "This");
maptolist.put(2, "Is");
maptolist.put(3, "A");
maptolist.put(4, "Demo");
maptolist.put(5, "Example");
List<Integer> nums = maptolist.keySet().stream().collect(Collectors.toList());
System.out.println("Keys:");
nums.forEach(System.out::println);
// Conversion Strings
List<String> chars = maptolist.values().stream().collect(Collectors.toList());
System.out.println("Values:");
chars.forEach(System.out::println);
}
}
输出:
Keys:
1
2
3
4
5
Values:
This
Is
A
Demo
Example
现在我们已经了解了 map 和列表背后的底层逻辑并使用了对象 Collectors
的组合,下面是一个可靠的 map 到列表程序,它可以在任何情况下工作。
keySet()
函数返回 map 中键的集合视图,以便 map 修改也适用于该集合。在这里,我们在一个 wrap 中形成一对来获得一个键列表,如下所示。
语法:
List<String> setkey = new ArrayList<String>(MAP2LIST.keySet());
示例 3:
package maptolist;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class MapToListExample3 {
public static void main(String[] args) {
// Define Map
Map<String, String> MAP2LIST = new HashMap<String, String>();
// Values (key as values)
MAP2LIST.put("1 + 1", "TWO");
MAP2LIST.put("10 - 5", "FIVE");
MAP2LIST.put("2.4 + 3.6", "SIX");
// Define list
List<String> setkey = new ArrayList<String>(MAP2LIST.keySet());
// foreach function extends setkey and prints the output
setkey.forEach(System.out::println);
List<String> setvalue = new ArrayList<String>(MAP2LIST.values());
setvalue.forEach(System.out::println);
}
}
输出:
10 - 5
1 + 1
2.4 + 3.6
FIVE
TWO
SIX
Sarwan Soomro is a freelance software engineer and an expert technical writer who loves writing and coding. He has 5 years of web development and 3 years of professional writing experience, and an MSs in computer science. In addition, he has numerous professional qualifications in the cloud, database, desktop, and online technologies. And has developed multi-technology programming guides for beginners and published many tech articles.
LinkedIn相关文章 - Java List
- Java 中的整数列表
- Java 中的列表与数组
- Java 中的数组列表
- 在 Java 中按字母顺序对列表进行排序
- 在 Java 中将列表转换为 map
- 在 Java 中的冒泡排序算法对手动链表进行排序