Java에서 맵 값을 목록으로 변환
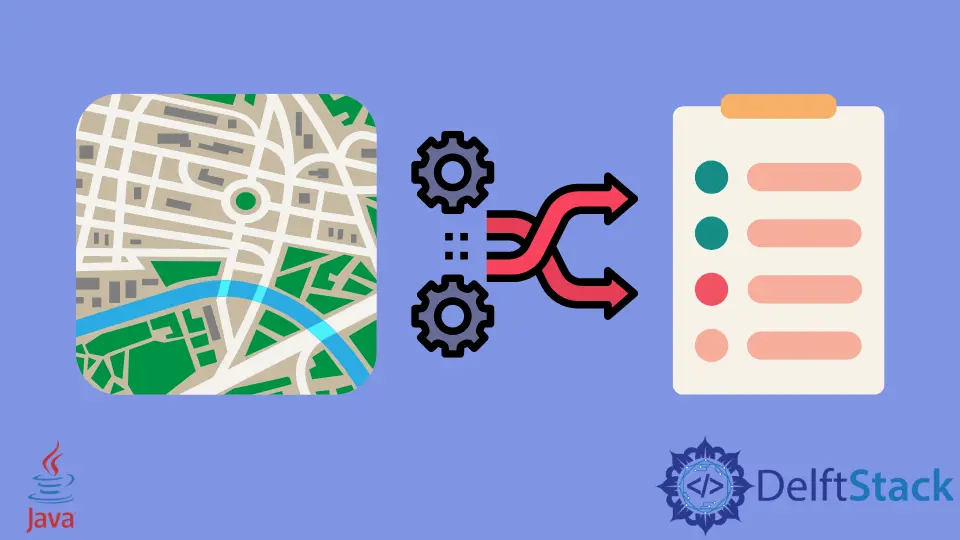
이 자습서에서는 해시 맵 값을 Java에서 목록으로 변환하는 방법을 보여주기 위해 세 가지 프로그램을 실행합니다.
Java에서 목록으로 변환할 맵 정의
지도 값을 목록으로 변환하기 전에 먼저 지도 개체가 있어야 하고 두 가지 데이터 유형, 즉 정수(키)와 문자열(값)을 목록으로 그룹화하기 전에 할당해야 합니다.
이 프로그램의 키는 자동차의 RPM 값이고 문자열은 색상입니다.
통사론:
Map<Integer, String> M2L = new HashMap<>();
M2L.put(5000, "Toyata Black");
M2L.put(6000, "Audi White");
M2L.put(8000, "BMW Red");
M2L.put(12000, "Buggati Silver");
Collector
스트림을 사용하여 Java에서 맵을 목록으로 변환
Collector
는 Java에서 개체를 확장하는 공개 클래스입니다. 또한 사용자가 미리 정의한 다양한 벤치마크를 기반으로 요소를 수집하고 기능을 요약하는 데 도움이 됩니다.
여기에서 keySet()
메서드를 사용하여 맵에서 반환된 집합에서 배열 목록을 만들어 키를 가져옵니다.
지도를 목록으로 변환하는 다음 예를 확인하십시오.
예 1:
package maptolist;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.stream.Collectors;
public class MapToList {
public static void main(String[] args) {
Map<Integer, String> M2L = new HashMap<>();
M2L.put(1, "New York");
M2L.put(2, "Toronto");
M2L.put(3, "Berlin");
List<Integer> ID = M2L.keySet().stream().collect(Collectors.toList());
ID.forEach(System.out::println);
List<String> NAME = M2L.values().stream().collect(Collectors.toList());
NAME.forEach(System.out::println);
}
}
출력:
1
2
3
New York
Toronto
Berlin
두 번째 예에서 다음 코드를 고려하십시오.
통사론:
List<Integer> nums = maptolist.keySet().stream().collect(Collectors.toList());
예 2:
package maptolist;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.stream.Collectors;
public class MaptoListExample2 {
public static void main(String[] args) {
Map<Integer, String> maptolist = new HashMap<>();
maptolist.put(1, "This");
maptolist.put(2, "Is");
maptolist.put(3, "A");
maptolist.put(4, "Demo");
maptolist.put(5, "Example");
List<Integer> nums = maptolist.keySet().stream().collect(Collectors.toList());
System.out.println("Keys:");
nums.forEach(System.out::println);
// Conversion Strings
List<String> chars = maptolist.values().stream().collect(Collectors.toList());
System.out.println("Values:");
chars.forEach(System.out::println);
}
}
출력:
Keys:
1
2
3
4
5
Values:
This
Is
A
Demo
Example
이제 지도와 목록 뒤에 있는 기본 논리를 보고 개체 수집기의 조합을 사용했으므로 아래는 모든 상황에서 작동하는 견고한 목록으로의 지도 프로그램입니다.
keySet()
함수는 지도 수정 사항도 집합에 적용되도록 지도에 있는 키의 집합 보기를 반환합니다. 여기에서 아래와 같이 키 목록을 얻기 위해 랩 내에서 쌍을 형성합니다.
통사론:
List<String> setkey = new ArrayList<String>(MAP2LIST.keySet());
예 3:
package maptolist;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class MapToListExample3 {
public static void main(String[] args) {
// Define Map
Map<String, String> MAP2LIST = new HashMap<String, String>();
// Values (key as values)
MAP2LIST.put("1 + 1", "TWO");
MAP2LIST.put("10 - 5", "FIVE");
MAP2LIST.put("2.4 + 3.6", "SIX");
// Define list
List<String> setkey = new ArrayList<String>(MAP2LIST.keySet());
// foreach function extends setkey and prints the output
setkey.forEach(System.out::println);
List<String> setvalue = new ArrayList<String>(MAP2LIST.values());
setvalue.forEach(System.out::println);
}
}
출력:
10 - 5
1 + 1
2.4 + 3.6
FIVE
TWO
SIX
Sarwan Soomro is a freelance software engineer and an expert technical writer who loves writing and coding. He has 5 years of web development and 3 years of professional writing experience, and an MSs in computer science. In addition, he has numerous professional qualifications in the cloud, database, desktop, and online technologies. And has developed multi-technology programming guides for beginners and published many tech articles.
LinkedIn관련 문장 - Java List
- Java를 사용하여 목록에서 요소의 인덱스 찾기
- Java에서 목록과 배열 목록의 차이점
- Java에서 목록을 청크로 분할
- Java의 목록에서 첫 번째 요소 가져오기
- Java의 필터 목록