How to Convert List to Map in Java
- Convert List to Map Using ArrayList and HashMap
-
Convert List to Map Using
Stream
andCollectors
in Java - Convert List to Map With Sort and Collect in Java
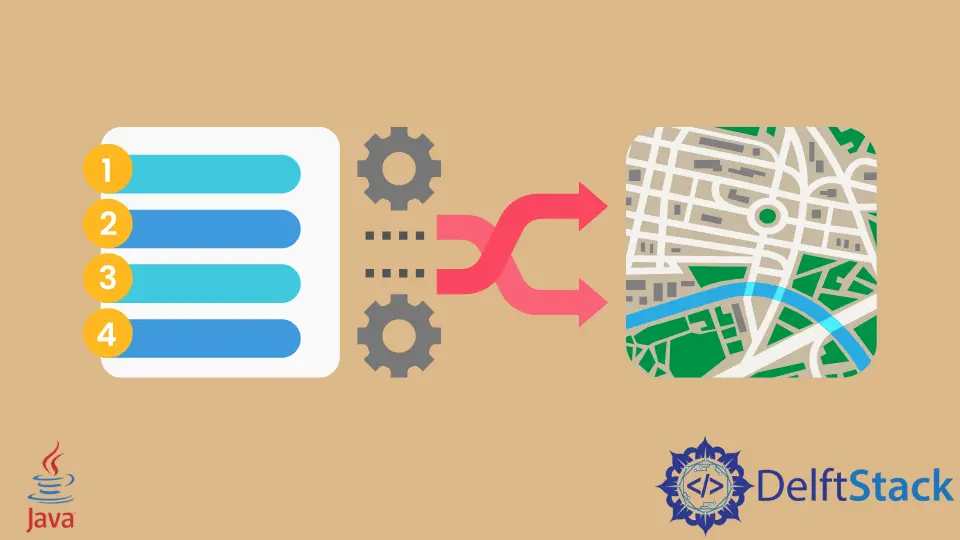
A List
allows maintaining an ordered collection of objects. A Map
object maps keys to values. In Java, there are several methods for converting a List
of objects into a Map
. In this article, we will learn some of them.
Convert List to Map Using ArrayList and HashMap
The List
interface in Java allows ordered collection of objects, and store the duplicate values. It offers index-based methods for updating, deleting, inserting, and searching elements. We can also store null values in the List
. ArrayList
, LinkedList
are some of the classes that implement the List
interface.
The Map
interface in Java maps unique keys to values and cannot contain duplicate keys. It has useful methods to search, update and insert elements based on of that unique key. The HashMap
class implements the Map
interface.
The class Book
has three member variables bookName
, author
, id
, and getter methods for these attributes. We have overridden the toString()
method to format the Book
class data. An object of the Book
class is created. The add()
method of the List
interface inserts objects into the bookList
.
We create a Map
object using the HashMap
class, which holds the key of Integer
type and value as Book
type. We use an enhanced for
loop in which we use the put()
method and add all the Book
data from the bookList
to the map.
The put()
method takes two arguments. We accessed the id
attribute by calling getId()
and using it as a key and storing the book class object b
as the value for that particular key. We can print the bookMap
object as shown in the output.
class Book {
int id;
String bookName;
String author;
public Book(int id, String bookName, String author) {
this.id = id;
this.bookName = bookName;
this.author = author;
}
public int getId() {
return id;
}
public String getAuthor() {
return author;
}
public String getBookName() {
return bookName;
}
@Override
public String toString() {
return "Book{"
+ " bookName='" + bookName + '\'' + ", author='" + author + '\'' + '}';
}
}
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class Test {
public static void main(String[] args) {
List<Book> bookList = new ArrayList<>();
bookList.add(new Book(1, "Barney's Version", "Mordecai Richler"));
bookList.add(new Book(2, "The Unsettlers", "Mark Sundeen"));
bookList.add(new Book(3, "The Debt to Pleasure", "John Lanchester"));
Map<Integer, Book> bookMap = new HashMap();
for (Book b : bookList) {
bookMap.put(b.getId(), b);
}
System.out.println("BookMap " + bookMap.toString());
}
}
Output:
BookMap {1=Book{ bookName="Barney's Version", author='Mordecai Richler'}, 2=Book{ bookName='The Unsettlers', author='Mark Sundeen'}, 3=Book{ bookName='The Debt to Pleasure', author='John Lanchester'}}
Convert List to Map Using Stream
and Collectors
in Java
It is easy to use the lambda function with Stream
and Collectors
in Java 8 to achieve the above task. The stream()
method returns a Stream
of Book
class objects from the bookList
.
To collect these elements, we use the collect()
method of the Stream
class. The elements will be collected in a map, so we used this Collectors.toMap()
. The key is the id
and stores the Book
object as a value. By printing the result1
as output, we can see that we converted bookList
into a Map.
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.stream.Collectors;
public class Test {
public static void main(String[] args) {
List<Book> bookList = new ArrayList<>();
bookList.add(new Book(1, "Barney's Version", "Mordecai Richler"));
bookList.add(new Book(2, "The Unsettlers", "Mark Sundeen"));
bookList.add(new Book(3, "The Debt to Pleasure", "John Lanchester"));
Map<Integer, String> result1 =
bookList.stream().collect(Collectors.toMap(Book::getId, Book::toString));
System.out.println("Result 1 : " + result1);
}
}
Output:
Result 1 : {1=Book{ bookName="Barney's Version", author='Mordecai Richler'}, 2=Book{ bookName='The Unsettlers', author='Mark Sundeen'}, 3=Book{ bookName='The Debt to Pleasure', author='John Lanchester'}}
Convert List to Map With Sort and Collect in Java
We can sort the stream of the Book
class object from bookList
by comparing the id
in reverse order. We first call comparingInt()
where we pass the id
that is int
type then reverse the order calling the reverse()
method.
We can collect the sorted stream on a Map
class object. If there is a duplicate key, which is 2
in our case, then the map will take the new value for the same key
that explains (oldValue, newValue) -> newValue
.
import java.util.*;
import java.util.stream.Collectors;
public class Test {
public static void main(String[] args) {
List<Book> bookList = new ArrayList<>();
bookList.add(new Book(1, "Barney's Version", "Mordecai Richler"));
bookList.add(new Book(2, "The Unsettlers", "Mark Sundeen"));
bookList.add(new Book(2, "The Debt to Pleasure", "John Lanchester"));
Map result1 = bookList.stream()
.sorted(Comparator.comparingInt(Book::getId).reversed())
.collect(Collectors.toMap(Book::getId, Book::toString,
(oldValue, newValue) -> newValue, LinkedHashMap::new));
System.out.println("Result 1 : " + result1);
}
}
Output:
Result 1 : {2=Book{ bookName='The Debt to Pleasure', author='John Lanchester'}, 1=Book{ bookName="Barney's Version", author='Mordecai Richler'}}
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedInRelated Article - Java List
- How to Split a List Into Chunks in Java
- How to Get First Element From List in Java
- How to Find the Index of an Element in a List Using Java
- How to Filter List in Java
- Differences Between List and Arraylist in Java
- List vs. Array in Java