How to Compare Arrays in Java
-
Use the
==
Operator to Compare Arrays in Java -
Use the
Arrays.equals()
Method to Compare Arrays in Java -
Use the
Arrays.deepEquals()
Method to Compare Arrays in Java -
Use the
for
Loop to Compare Arrays in Java
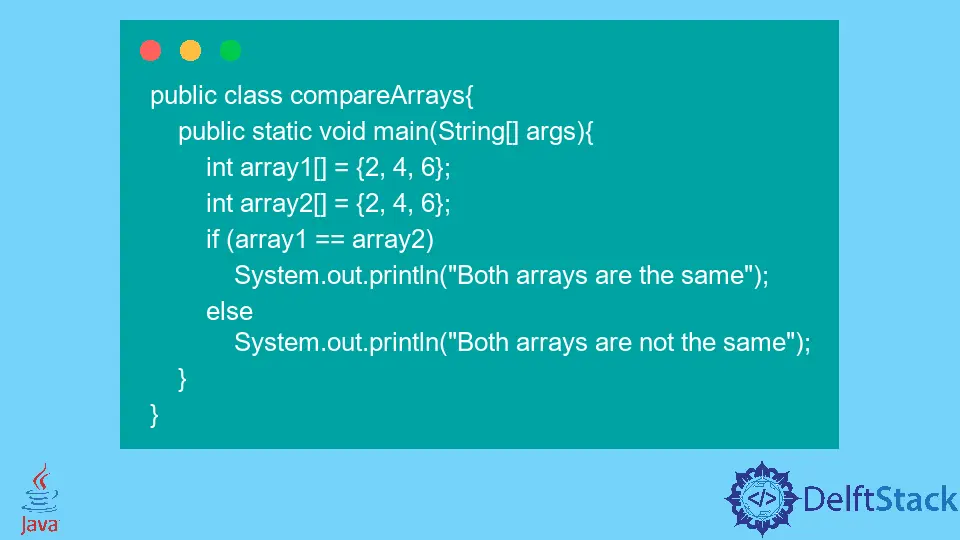
Today, we will write different code snippets to compare arrays in Java. We will see how we can use the ==
operator, Arrays.equals()
, Arrays.deepEquals()
, and a custom function that contains a for
loop to compare arrays in Java.
Use the ==
Operator to Compare Arrays in Java
Example Code:
public class compareArrays {
public static void main(String[] args) {
int array1[] = {2, 4, 6};
int array2[] = {2, 4, 6};
if (array1 == array2)
System.out.println("Both arrays are the same");
else
System.out.println("Both arrays are not the same");
}
}
Output:
Both arrays are not the same
In the main
function, we have two arrays, array1
and array2
, referring to two various objects. So, the two different reference variables are compared, resulting in Both arrays are not the same
.
Use the Arrays.equals()
Method to Compare Arrays in Java
Example code:
import java.util.Arrays;
public class compareArrays {
public static void main(String[] args) {
int array1[] = {2, 4, 6};
int array2[] = {2, 4, 6};
if (Arrays.equals(array1, array2))
System.out.println("Both arrays are the same");
else
System.out.println("Both arrays are not the same");
}
}
Output:
Both arrays are the same
This main
method also contains two arrays named array1
and array2
. Here, we use the Arrays.equals()
method that resides in Arrays Class and takes two arrays and compares their content.
Suppose we have two 2D arrays that we need to compare. Can we get the advantage of the same approach as given above for deep comparison? No.
In the Java Arrays class, we have various equals()
methods for primitive types, such as int, char, etc. And one equals()
method for the Object
class, but we can’t use it to make the deep comparison for two-dimensional arrays.
Example code:
import java.util.Arrays;
public class compareArrays {
public static void main(String[] args) {
int innerArray1[] = {2, 4, 6};
int innerArray2[] = {2, 4, 6};
Object outerArray1[] = {innerArray1};
Object outerArray2[] = {innerArray2};
if (Arrays.equals(outerArray1, outerArray2))
System.out.println("Both arrays are the same");
else
System.out.println("Both arrays are not the same");
}
}
Output:
Both arrays are not the same
We can use the deepEquals()
method for deep comparison, which is helpful if we have two-dimensional arrays.
Use the Arrays.deepEquals()
Method to Compare Arrays in Java
Example code:
import java.util.Arrays;
public class compareArrays {
public static void main(String[] args) {
int innerArray1[] = {2, 4, 6};
int innerArray2[] = {2, 4, 6};
Object outerArray1[] = {innerArray1};
Object outerArray2[] = {innerArray2};
if (Arrays.deepEquals(outerArray1, outerArray2))
System.out.println("Both arrays are the same");
else
System.out.println("Both arrays are not the same");
}
}
Output:
Both arrays are the same
The deepEquals()
method works fine for deep comparison. The Arrays.deepEquals()
method checks whether two arrays are equal or not.
These two arrays can be one, two, or even multi-dimensional.
How does this method decides whether the provided arrays are equal or not? For that, you must have the following points in mind:
- If the references of both provided arrays are null, the arrays would be deeply equal.
- We can declare two array references deeply equal if they point to the arrays containing the exact elements’ number and the corresponding elements’ pairs.
- Two elements,
element1
andelement2
that are possibly null, would be deeply equal if any one condition from the following list holds:- The
Arrays.deepEquals(element1, element2)
returns true if the type of both arrays is an object reference. - The
Arrays.equals(element1, element2)
returns true if both arrays are of the exact primitive type. element1 == element2
element1.equals(element2)
returns true.
- The
Use the for
Loop to Compare Arrays in Java
Example code:
public class compareArrays {
public static boolean compare(int[] array1, int[] array2) {
boolean flag = true;
if (array1 != null && array2 != null) {
if (array1.length != array2.length)
flag = false;
else
for (int i = 0; i < array2.length; i++) {
if (array2[i] != array1[i]) {
flag = false;
}
}
} else {
flag = false;
}
return flag;
}
public static void main(String[] args) {
int array1[] = {2, 4, 6};
int array2[] = {2, 4, 6};
if (compare(array1, array2))
System.out.println("Both arrays are the same");
else
System.out.println("Both arrays are not the same");
}
}
Output:
Both arrays are the same
Here, we write a compare()
function that takes two arrays to the int type. It compares them on individual element level using the for
loop and returns true if both arrays are the same; otherwise, false.