在 Java 中比較陣列
Mehvish Ashiq
2023年10月12日
Java
Java Array
-
在 Java 中使用
==
運算子比較陣列 -
使用
Arrays.equals()
方法比較 Java 中的陣列 -
使用
Arrays.deepEquals()
方法比較 Java 中的陣列 -
使用
for
迴圈比較 Java 中的陣列
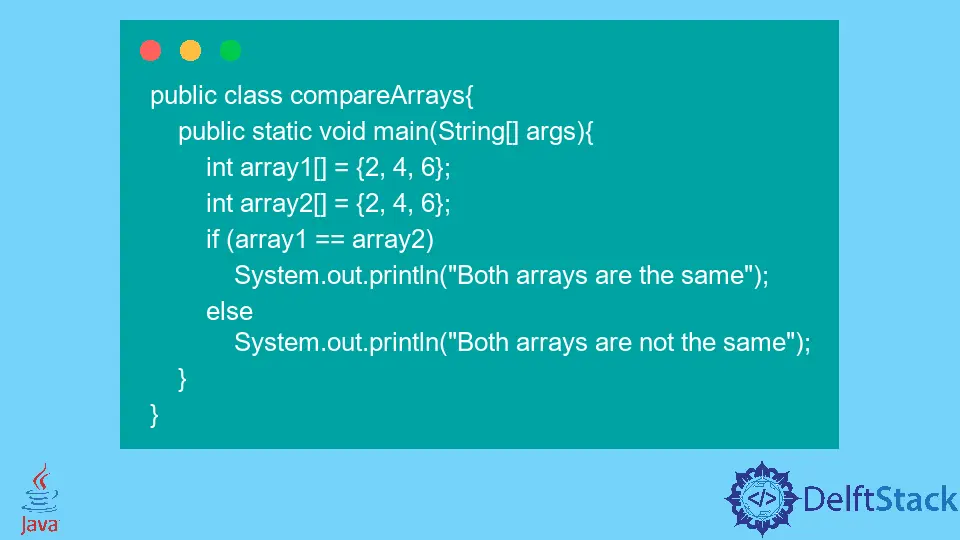
今天,我們將編寫不同的程式碼片段來比較 Java 中的陣列。我們將看到如何使用 ==
運算子、Arrays.equals()
、Arrays.deepEquals()
以及包含 for
迴圈的自定義函式來比較 Java 中的陣列。
在 Java 中使用 ==
運算子比較陣列
示例程式碼:
public class compareArrays {
public static void main(String[] args) {
int array1[] = {2, 4, 6};
int array2[] = {2, 4, 6};
if (array1 == array2)
System.out.println("Both arrays are the same");
else
System.out.println("Both arrays are not the same");
}
}
輸出:
Both arrays are not the same
在 main
函式中,我們有兩個陣列 array1
和 array2
,指的是兩個不同的物件。因此,比較兩個不同的參考變數,導致 Both arrays are not the same
。
使用 Arrays.equals()
方法比較 Java 中的陣列
示例程式碼:
import java.util.Arrays;
public class compareArrays {
public static void main(String[] args) {
int array1[] = {2, 4, 6};
int array2[] = {2, 4, 6};
if (Arrays.equals(array1, array2))
System.out.println("Both arrays are the same");
else
System.out.println("Both arrays are not the same");
}
}
輸出:
Both arrays are the same
這個 main
方法還包含兩個名為 array1
和 array2
的陣列。在這裡,我們使用位於 Arrays Class 中的 Arrays.equals()
方法並獲取兩個陣列並比較它們的內容。
假設我們有兩個需要比較的二維陣列。我們能否利用上面給出的相同方法進行深度比較?不。
在 Java Arrays 類中,我們有各種基本型別的 equals()
方法,例如 int、char 等。Object
類有一個 equals()
方法,但我們不能使用它對二維陣列進行深度比較。
示例程式碼:
import java.util.Arrays;
public class compareArrays {
public static void main(String[] args) {
int innerArray1[] = {2, 4, 6};
int innerArray2[] = {2, 4, 6};
Object outerArray1[] = {innerArray1};
Object outerArray2[] = {innerArray2};
if (Arrays.equals(outerArray1, outerArray2))
System.out.println("Both arrays are the same");
else
System.out.println("Both arrays are not the same");
}
}
輸出:
Both arrays are not the same
我們可以使用 deepEquals()
方法進行深度比較,如果我們有二維陣列,這將很有幫助。
使用 Arrays.deepEquals()
方法比較 Java 中的陣列
示例程式碼:
import java.util.Arrays;
public class compareArrays {
public static void main(String[] args) {
int innerArray1[] = {2, 4, 6};
int innerArray2[] = {2, 4, 6};
Object outerArray1[] = {innerArray1};
Object outerArray2[] = {innerArray2};
if (Arrays.deepEquals(outerArray1, outerArray2))
System.out.println("Both arrays are the same");
else
System.out.println("Both arrays are not the same");
}
}
輸出:
Both arrays are the same
deepEquals()
方法適用於深度比較。Arrays.deepEquals()
方法檢查兩個陣列是否相等。
這兩個陣列可以是一維的、二維的,甚至是多維的。
此方法如何確定提供的陣列是否相等?為此,你必須牢記以下幾點:
- 如果兩個提供的陣列的引用均為空,則陣列將非常相等。
- 如果兩個陣列引用指向包含確切元素數量和對應元素對的陣列,我們可以宣告它們完全相等。
- 如果以下列表中的任何一個條件成立,則可能為 null 的兩個元素
element1
和element2
將完全相等:- 如果兩個陣列的型別都是物件引用,則
Arrays.deepEquals(element1, element2)
返回 true。 - 如果兩個陣列都屬於精確的原始型別,則
Arrays.equals(element1, element2)
返回 true。 element1 == element2
element1.equals(element2)
返回 true。
- 如果兩個陣列的型別都是物件引用,則
使用 for
迴圈比較 Java 中的陣列
示例程式碼:
public class compareArrays {
public static boolean compare(int[] array1, int[] array2) {
boolean flag = true;
if (array1 != null && array2 != null) {
if (array1.length != array2.length)
flag = false;
else
for (int i = 0; i < array2.length; i++) {
if (array2[i] != array1[i]) {
flag = false;
}
}
} else {
flag = false;
}
return flag;
}
public static void main(String[] args) {
int array1[] = {2, 4, 6};
int array2[] = {2, 4, 6};
if (compare(array1, array2))
System.out.println("Both arrays are the same");
else
System.out.println("Both arrays are not the same");
}
}
輸出:
Both arrays are the same
在這裡,我們編寫了一個 compare()
函式,它將兩個陣列轉換為 int 型別。它使用 for
迴圈在單個元素級別上比較它們,如果兩個陣列相同則返回 true;否則為假。
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: Mehvish Ashiq