Java에서 배열 비교
-
==
연산자를 사용하여 Java에서 배열 비교 -
Arrays.equals()
메서드를 사용하여 Java에서 배열 비교 -
Arrays.deepEquals()
메서드를 사용하여 Java에서 배열 비교 -
for
루프를 사용하여 Java에서 배열 비교
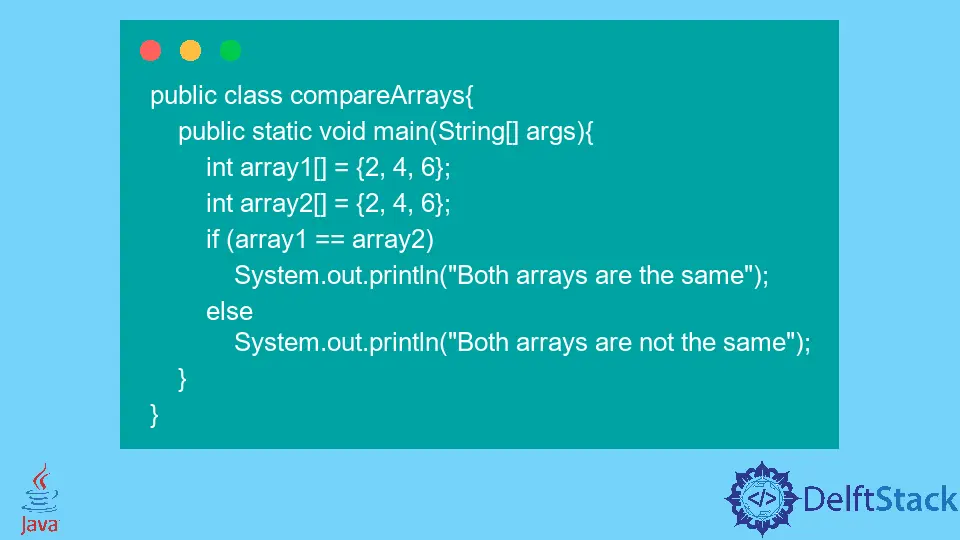
오늘, 우리는 자바에서 배열을 비교하기 위해 다른 코드 조각을 작성할 것입니다. ==
연산자, Arrays.equals()
, Arrays.deepEquals()
및 for
루프가 포함된 사용자 정의 함수를 사용하여 Java에서 배열을 비교하는 방법을 살펴보겠습니다.
==
연산자를 사용하여 Java에서 배열 비교
예제 코드:
public class compareArrays {
public static void main(String[] args) {
int array1[] = {2, 4, 6};
int array2[] = {2, 4, 6};
if (array1 == array2)
System.out.println("Both arrays are the same");
else
System.out.println("Both arrays are not the same");
}
}
출력:
Both arrays are not the same
main
함수에는 두 개의 다양한 객체를 참조하는 array1
과 array2
라는 두 개의 배열이 있습니다. 따라서 서로 다른 두 기준 변수를 비교하여 Both arrays are not the same
이 발생합니다.
Arrays.equals()
메서드를 사용하여 Java에서 배열 비교
예제 코드:
import java.util.Arrays;
public class compareArrays {
public static void main(String[] args) {
int array1[] = {2, 4, 6};
int array2[] = {2, 4, 6};
if (Arrays.equals(array1, array2))
System.out.println("Both arrays are the same");
else
System.out.println("Both arrays are not the same");
}
}
출력:
Both arrays are the same
이 main
메소드에는 array1
및 array2
라는 두 개의 어레이도 포함되어 있습니다. 여기에서는 Arrays Class에 있는 Arrays.equals()
메서드를 사용하고 두 개의 배열을 가져와서 내용을 비교합니다.
비교할 필요가 있는 두 개의 2D 배열이 있다고 가정합니다. 심층 비교를 위해 위에 제공된 것과 동일한 접근 방식의 이점을 얻을 수 있습니까? 아니요.
Java Arrays 클래스에는 int, char 등과 같은 기본 유형에 대한 다양한 equals()
메소드가 있습니다. 그리고 Object
클래스에는 equals()
메소드가 있지만 이를 사용할 수는 없습니다. 2차원 배열에 대한 심층 비교를 수행합니다.
예제 코드:
import java.util.Arrays;
public class compareArrays {
public static void main(String[] args) {
int innerArray1[] = {2, 4, 6};
int innerArray2[] = {2, 4, 6};
Object outerArray1[] = {innerArray1};
Object outerArray2[] = {innerArray2};
if (Arrays.equals(outerArray1, outerArray2))
System.out.println("Both arrays are the same");
else
System.out.println("Both arrays are not the same");
}
}
출력:
Both arrays are not the same
심층 비교를 위해 deepEquals()
메서드를 사용할 수 있습니다. 이는 2차원 배열이 있는 경우에 유용합니다.
Arrays.deepEquals()
메서드를 사용하여 Java에서 배열 비교
예제 코드:
import java.util.Arrays;
public class compareArrays {
public static void main(String[] args) {
int innerArray1[] = {2, 4, 6};
int innerArray2[] = {2, 4, 6};
Object outerArray1[] = {innerArray1};
Object outerArray2[] = {innerArray2};
if (Arrays.deepEquals(outerArray1, outerArray2))
System.out.println("Both arrays are the same");
else
System.out.println("Both arrays are not the same");
}
}
출력:
Both arrays are the same
deepEquals()
메서드는 심층 비교에 적합합니다. Arrays.deepEquals()
메서드는 두 배열이 같은지 여부를 확인합니다.
이 두 배열은 1차원, 2차원 또는 다차원일 수 있습니다.
이 방법은 제공된 배열이 동일한지 여부를 어떻게 결정합니까? 이를 위해서는 다음 사항을 염두에 두어야 합니다.
- 제공된 두 배열의 참조가 모두 null인 경우 배열은 완전히 동일합니다.
- 두 개의 배열 참조가 정확한 요소의 번호와 해당 요소의 쌍을 포함하는 배열을 가리키는 경우 완전히 동일하다고 선언할 수 있습니다.
- null일 가능성이 있는 두 요소
element1
및element2
는 다음 목록의 조건 중 하나가 충족되는 경우 완전히 동일합니다.Arrays.deepEquals(element1, element2)
는 두 어레이의 유형이 객체 참조인 경우 true를 반환합니다.Arrays.equals(element1, element2)
는 두 어레이가 모두 정확한 기본 유형인 경우 true를 반환합니다.element1 == element2
element1.equals(element2)
는 true를 반환합니다.
for
루프를 사용하여 Java에서 배열 비교
예제 코드:
public class compareArrays {
public static boolean compare(int[] array1, int[] array2) {
boolean flag = true;
if (array1 != null && array2 != null) {
if (array1.length != array2.length)
flag = false;
else
for (int i = 0; i < array2.length; i++) {
if (array2[i] != array1[i]) {
flag = false;
}
}
} else {
flag = false;
}
return flag;
}
public static void main(String[] args) {
int array1[] = {2, 4, 6};
int array2[] = {2, 4, 6};
if (compare(array1, array2))
System.out.println("Both arrays are the same");
else
System.out.println("Both arrays are not the same");
}
}
출력:
Both arrays are the same
여기에서 두 개의 배열을 int 유형으로 가져오는 compare()
함수를 작성합니다. for
루프를 사용하여 개별 요소 수준에서 이를 비교하고 두 배열이 동일하면 true를 반환합니다. 그렇지 않으면 거짓.