Array of Linked List in Java
- Create an Array of Linked Lists in Java Using a Traditional Array
- Create an Array of Linked Lists in Java Using the Constructor
-
Create an Array of Linked Lists in Java Using the
Object[]
Type Array - Create an Array of Linked Lists in Java Using the Apache Commons Collections Package
- Conclusion
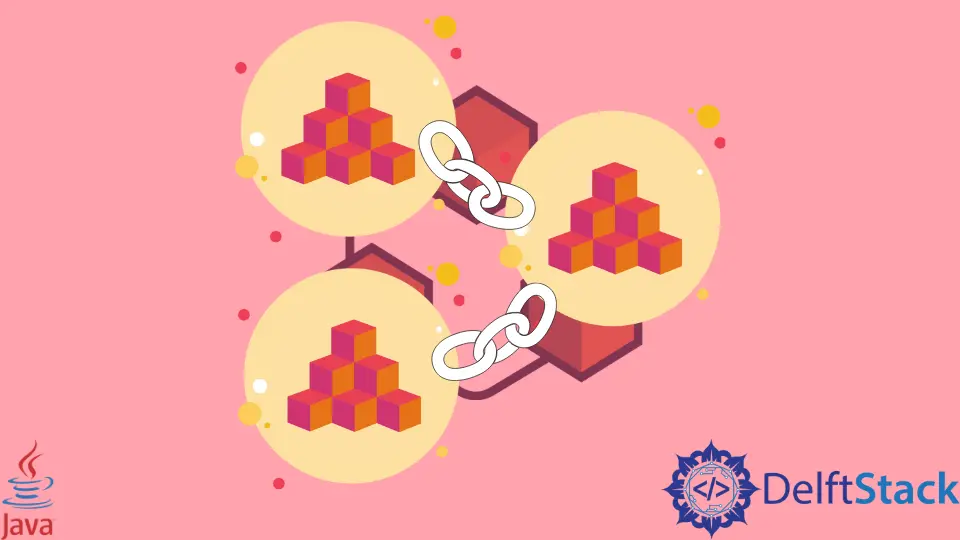
Java, with its robust set of libraries and versatile data structures, offers developers the flexibility to implement solutions tailored to specific needs. One such powerful combination is the creation of an array of linked lists, which provides a dynamic and efficient way to organize and manage data.
In this article, we’ll delve into various methods of creating an array of linked lists in Java.
Create an Array of Linked Lists in Java Using a Traditional Array
An array of linked lists is a useful data structure when you want to organize and manage multiple linked lists efficiently. Each element of the array represents a separate linked list, allowing you to organize data into different groups or buckets.
This can be particularly helpful in scenarios like hash tables, graph representations, or any situation where data needs to be grouped dynamically.
To create an array of linked lists, we’ll use the java.util.LinkedList
class. Each element in the array will be an instance of a linked list, allowing for flexible data organization.
The key steps involve declaring the array, instantiating individual linked lists within a loop, and populating them with elements. See the code below.
import java.util.LinkedList;
public class LinkedListArrayExample {
public static void main(String[] args) {
LinkedList[] linkedListArray = new LinkedList[5];
for (int i = 0; i < linkedListArray.length; i++) {
linkedListArray[i] = new LinkedList();
for (int j = 0; j < i + 1; j++) {
linkedListArray[i].add(j);
}
}
for (LinkedList list : linkedListArray) {
System.out.print(list + " ");
}
}
}
We begin by declaring an array of linked lists named linkedListArray
. Here, we create an array capable of holding five linked lists, as indicated by LinkedList[5]
.
LinkedList[] linkedListArray = new LinkedList[5];
Next, we use a for
loop to iterate through each element in the array. Inside the loop, we instantiate a new linked list for each array element (linkedListArray[i] = new LinkedList();
), effectively creating an array of linked lists.
Additionally, within a nested loop, we populate each linked list with elements. The inner loop (for (int j = 0; j < i + 1; j++)
) adds elements incrementally to each linked list.
This means that the number of elements added corresponds to the index of the linked list in the array plus one. This creates a pattern where the first list has one element, the second has two, and so forth.
Once our array of linked lists is populated, we use another loop to display the content of each linked list in the array (System.out.print(list + " ");
).
Output:
[0] [0, 1] [0, 1, 2] [0, 1, 2, 3] [0, 1, 2, 3, 4]
In this example, the array of linked lists is successfully created and populated, showcasing the dynamic nature of linked lists in Java. Each linked list in the array contains elements based on its position in the array.
Create an Array of Linked Lists in Java Using the Constructor
Let’s explore an alternative method to create an array of linked lists using the constructor. This is a special method that is invoked when an object is instantiated.
Constructors are used to initialize the state of an object. By employing constructors, we can set up our array of linked lists with a specific size and initiate each linked list individually.
The primary steps in this approach include creating instances of LinkedList
using the new
keyword, adding elements to each list, and then adding these lists to an ArrayList
.
Take a look at the code example below:
import java.util.ArrayList;
import java.util.LinkedList;
public class LinkedListConstructorExample {
public static void main(String[] args) {
LinkedList list1 = new LinkedList();
list1.add("Hello");
list1.add("world");
LinkedList list2 = new LinkedList();
list2.add("DelftStack");
list2.add("tutorial");
ArrayList<LinkedList> linkedListArray = new ArrayList<>();
linkedListArray.add(list1);
linkedListArray.add(list2);
linkedListArray.forEach(System.out::println);
System.out.println("Classname: " + linkedListArray.get(0).getClass());
}
}
We start by creating two linked lists, list1
and list2
, using the LinkedList
constructor. Each list is then populated with string elements using the add
method.
In this case, we’ve added value1
and value2
to both lists. You can customize these values or add more linked lists as needed.
Now, we move on to the next step, where we create an ArrayList
named linkedListArray
to hold our linked lists. This provides a flexible container for managing multiple linked lists of the same type.
ArrayList<LinkedList> linkedListArray = new ArrayList<>();
To populate our ArrayList
, we add the previously created linked lists (list1
and list2
) using the add
method. This step can be repeated to include more linked lists in the array.
linkedListArray.add(list1);
linkedListArray.add(list2);
For visualization purposes, we use the forEach
method to iterate through the ArrayList
and print the content of each linked list on separate lines. Additionally, we print the class name of the first linked list in the ArrayList
to highlight the dynamic nature of the data structure.
linkedListArray.forEach(System.out::println);
System.out.println("Classname: " + linkedListArray.get(0).getClass());
Output:
[value1, value2]
[value1, value2]
Classname: class java.util.LinkedList
As you can see, the array of linked lists is successfully created using the constructor approach. The ArrayList
provides a flexible container for managing and iterating through the linked lists.
Create an Array of Linked Lists in Java Using the Object[]
Type Array
In Java, we can also create an array of linked lists using an Object[]
type array to provide a more generalized approach. This allows us to handle different types of linked lists within the same array.
To implement an array of linked lists using an Object[]
type array, we declare the array and instantiate linked lists within it. Since arrays in Java can hold objects of any type, we can mix and match different types of linked lists.
Let’s have an example code:
import java.util.LinkedList;
public class LinkedListObjectArrayExample {
public static void main(String[] args) {
LinkedList<String> list1 = new LinkedList<>();
list1.add("DelftStack");
list1.add("tutorial");
LinkedList<Integer> list2 = new LinkedList<>();
list2.add(1);
list2.add(2);
Object[] objectArray = new Object[2];
objectArray[0] = list1;
objectArray[1] = list2;
LinkedList<String> retrievedList1 = (LinkedList<String>) objectArray[0];
LinkedList<Integer> retrievedList2 = (LinkedList<Integer>) objectArray[1];
System.out.println(retrievedList1);
System.out.println(retrievedList2);
}
}
We start by creating instances of linked lists of different types.
In this example, list1
is of type LinkedList<String>
and list2
is of type LinkedList<Integer>
. You can create more linked lists with various types as needed.
We then declare an array of the Object
type named objectArray
. This array is capable of holding objects of different types, including our linked lists.
Object[] objectArray = new Object[2];
The created linked lists (list1
and list2
) are stored in the Object[]
array at specific indices.
objectArray[0] = list1;
objectArray[1] = list2;
We retrieve the linked lists from the array and explicitly cast them back to their original types. This step is crucial for accessing the methods and properties of the linked lists.
LinkedList<String> retrievedList1 = (LinkedList<String>) objectArray[0];
LinkedList<Integer> retrievedList2 = (LinkedList<Integer>) objectArray[1];
Finally, we print the content of the retrieved linked lists. The output of the program will be:
[DelftStack, tutorial]
[1, 2]
Create an Array of Linked Lists in Java Using the Apache Commons Collections Package
Apache Commons Collections is a library that extends the functionality of Java’s standard collections. Specifically, we will use the CursorableLinkedList
class to enhance our linked lists with cursor functionality, providing flexible navigation through the elements.
Before you start using the Apache Commons Collections, make sure you have it added to your project. You can download the library from the Apache Commons website or include it as a Maven dependency in your project.
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-collections4</artifactId>
<version>4.4</version>
</dependency>
The primary class we’ll utilize is CursorableLinkedList
, which extends AbstractLinkedList
. This class introduces cursor functionality, allowing us to navigate through the linked list efficiently.
Let’s see how we can use the Apache Commons Collections Package to create an array of linked lists.
import org.apache.commons.collections4.list.AbstractLinkedList;
import org.apache.commons.collections4.list.CursorableLinkedList;
public class ArrayOfLinkedListsExample {
public static void main(String[] args) {
int arraySize = 5;
AbstractLinkedList<Integer>[] arrayOfLists = new CursorableLinkedList[arraySize];
for (int i = 0; i < arraySize; i++) {
arrayOfLists[i] = new CursorableLinkedList<>();
}
for (int i = 0; i < arraySize; i++) {
for (int j = 1; j <= 5; j++) {
arrayOfLists[i].add(j + (i * 5));
}
}
for (int i = 0; i < arraySize; i++) {
System.out.println("Linked List " + (i + 1) + ": " + arrayOfLists[i]);
}
}
}
Here, we begin by setting the size of the array with int arraySize = 5;
. This variable determines the number of linked lists we intend to create.
Next, we declare an array named arrayOfLists
to store instances of AbstractLinkedList<Integer>
. However, note that we instantiate it with CursorableLinkedList
, a concrete implementation of AbstractLinkedList
.
AbstractLinkedList<Integer>[] arrayOfLists = new CursorableLinkedList[arraySize];
This provides additional functionality, such as cursor navigation enhancing the linked lists within the array.
Moving on to the initialization phase, we use a loop to create new instances of CursorableLinkedList
and assign them to the respective positions in the array.
for (int i = 0; i < arraySize; i++) {
arrayOfLists[i] = new CursorableLinkedList<>();
}
Now, with the array of linked lists set up, we populate each linked list with elements. Nested loops are used for this task, with the inner loop adding elements based on a specific pattern.
for (int i = 0; i < arraySize; i++) {
for (int j = 1; j <= 5; j++) {
arrayOfLists[i].add(j + (i * 5));
}
}
The values are calculated using j + (i * 5)
, creating an ascending sequence that starts from different points for each linked list.
Another loop iterates through each linked list, and the System.out.println
statement displays the elements, along with a label indicating the linked list number.
Output:
Linked List 1: [1, 2, 3, 4, 5]
Linked List 2: [6, 7, 8, 9, 10]
Linked List 3: [11, 12, 13, 14, 15]
Linked List 4: [16, 17, 18, 19, 20]
Linked List 5: [21, 22, 23, 24, 25]
Conclusion
Creating an array of linked lists in Java offers a powerful solution for scenarios requiring dynamic and efficient data organization. Each method discussed in this article has its strengths, and the choice depends on the specific requirements of your application.
Whether you opt for a traditional array, constructor-based approach, Object[]
type array, or leverage the Apache Commons Collections Package, understanding the principles of linked lists and arrays is essential. Mastering the creation of an array of linked lists equips you with a valuable tool for designing robust and flexible data structures in Java.
Rashmi is a professional Software Developer with hands on over varied tech stack. She has been working on Java, Springboot, Microservices, Typescript, MySQL, Graphql and more. She loves to spread knowledge via her writings. She is keen taking up new things and adopt in her career.
LinkedInRelated Article - Java Linked List
- How to Remove a Node From a Linked List in Java
- How to Print Linked List in Java
- How to Sort Linked List in Java
- Doubly Linked List in Java