How to Print Linked List in Java
- Directly Print a Linked List in Java
-
Print a Linked List in Java Using the
toString()
Method - Print a Linked List in Java Using a Loop
- Print a Linked List in Java Using a Recursive Approach
- Print a Linked List Using Java Streams
-
Print a Linked List in Java Using
StringBuilder
- Print a Linked List in Java Using an Iterator
- Conclusion
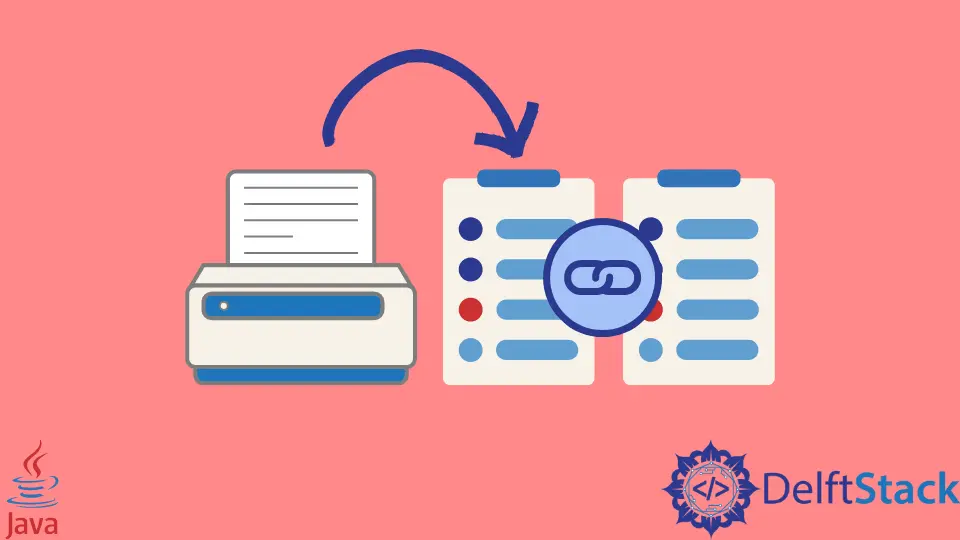
A linked list is a fundamental data structure that organizes elements, known as nodes, sequentially. Printing the contents of a linked list is a common operation, and Java provides various methods to achieve this.
In this article, we’ll explore different approaches to printing a linked list in Java, covering both basic and advanced techniques.
Directly Print a Linked List in Java
Printing a linked list involves traversing through its nodes and displaying the stored data. The LinkedList
class provides a straightforward way to create and manage linked lists.
In the code example below, we’ll create a simple linked list of strings, add some elements to it, and then print the entire list using the System.out.println()
statement.
import java.util.*;
public class PrintLinkedListBasics {
public static void main(String[] args) {
LinkedList<String> fruits = new LinkedList<>();
fruits.add("apple");
fruits.add("orange");
fruits.add("mango");
System.out.println(fruits);
}
}
In this example, we start by importing the java.util
package, which includes the LinkedList
class. We then declare a class named PrintLinkedListBasics
with the main
method as the entry point.
Inside the main
method:
- We create a new
LinkedList
namedfruits
to store strings. - Using the
add
method, we insert three elements (apple
,orange
, andmango
) into the linked list. - Finally, we print the entire linked list using
System.out.println(fruits)
.
This code demonstrates the simplicity of printing a linked list in Java, where the System.out.println()
method automatically calls the toString()
method of the LinkedList
class, displaying the elements in the desired format.
After executing the provided code, the output will be:
[apple, orange, mango]
In this output, the linked list is printed as a string, enclosed within square brackets, and with elements separated by commas. This format is the default representation provided by the LinkedList
class’s toString()
method.
Print a Linked List in Java Using the toString()
Method
In Java, printing a linked list using the toString()
method provides a more controlled and customizable representation of the linked list as a string. This method allows us to define how the linked list should appear when converted to a string.
Let’s explore this approach by enhancing our previous example.
import java.util.*;
public class PrintLinkedListToString {
public static void main(String[] args) {
LinkedList<String> fruits = new LinkedList<>();
fruits.add("apple");
fruits.add("orange");
fruits.add("mango");
System.out.println(fruits.toString());
}
}
In this example, we’ve omitted the direct use of System.out.println(fruits)
, and instead, we rely on the toString()
method to represent the linked list as a string. The toString()
method is implicitly called when the linked list is used within the System.out.println()
statement.
In this code snippet, we follow a similar process as before, creating a linked list named fruits
and adding three string elements to it. The crucial difference lies in the way we print the linked list. Instead of relying on the default toString()
implementation of the LinkedList
class, we explicitly call toString()
in the System.out.println()
statement.
This approach provides greater flexibility because, if needed, we can override the toString()
method in a custom class to control the formatting of the linked list’s string representation. The output of the code will remain the same as in the previous example, but this time, it explicitly emphasizes the use of the toString()
method for string representation.
After executing the provided code, the output remains:
[apple, orange, mango]
The linked list is still printed as a string, enclosed within square brackets, and with elements separated by commas. However, the explicit use of the toString()
method allows for a more intentional and customizable string representation, especially when dealing with user-defined classes or complex data structures.
Print a Linked List in Java Using a Loop
To print a linked list in Java using a loop, we iterate through the nodes of the list, accessing and printing the data of each node. This method provides a manual way of traversing the linked list, offering control over the printing process.
The code example below demonstrates how to achieve this:
import java.util.*;
public class PrintLinkedListWithLoop {
public static void main(String[] args) {
LinkedList<String> fruits = new LinkedList<>();
fruits.add("apple");
fruits.add("orange");
fruits.add("mango");
for (String fruit : fruits) {
System.out.print(fruit + " ");
}
}
}
In this example, we create a linked list of strings named fruits
and add three elements to it. Instead of relying on the built-in methods, we manually traverse the linked list using a for
loop. For each iteration, the current element is stored in the variable fruit
, and then it prints the element followed by a space.
This approach provides explicit control over the traversal process, allowing for additional logic or formatting if needed. It’s particularly useful when custom behaviors are required during the printing process.
After executing the provided code, the output will be:
apple orange mango
The linked list is printed with elements separated by spaces, demonstrating the manual traversal and printing of the linked list using a loop in Java.
Print a Linked List in Java Using a Recursive Approach
Printing a linked list in Java using a recursive approach involves defining a method that prints each node and calls itself for the next node in the list. This method uses the concept of recursion, where a function calls itself until a specific condition is met.
The code example below demonstrates how to print a linked list recursively:
import java.util.*;
public class PrintLinkedListRecursively {
public static void main(String[] args) {
LinkedList<String> fruits = new LinkedList<>();
fruits.add("apple");
fruits.add("orange");
fruits.add("mango");
printLinkedListRecursive(fruits.getFirstNode());
}
static void printLinkedListRecursive(Node current) {
if (current == null) {
return;
}
System.out.print(current.data + " ");
printLinkedListRecursive(current.next);
}
static class Node {
String data;
Node next;
Node(String data) {
this.data = data;
this.next = null;
}
}
static class LinkedList<T> {
Node first;
void add(T data) {
Node newNode = new Node(data.toString());
if (first == null) {
first = newNode;
} else {
Node temp = first;
while (temp.next != null) {
temp = temp.next;
}
temp.next = newNode;
}
}
Node getFirstNode() {
return first;
}
}
}
In this example, the printLinkedListRecursive
method is a recursive function responsible for printing the elements of the linked list. It takes a Node
parameter named current
, representing the current node in the recursion.
The base case checks if the current node is null
, and if so, the method returns, terminating the recursion. Otherwise, it prints the data of the current node, followed by a space, and makes a recursive call to printLinkedListRecursive
with the next node in the linked list (current.next
).
The Node
class defines the structure of a node in the linked list. Each node has a String
data field representing the element and a Node
field named next
pointing to the next node in the list. The class includes a constructor to initialize a node with the given data.
Additionally, an inner LinkedList
class is introduced. It manages the linked list by maintaining a Node
field named first
, representing the head of the list. The add
method appends a new node with the specified data to the end of the linked list.
If the list is empty, the new node becomes the head. The getFirstNode
method returns the head node of the linked list.
After executing the provided code, the output will be:
apple orange mango
The linked list is printed with elements separated by spaces, showcasing the recursive approach to printing a linked list in Java.
Print a Linked List Using Java Streams
Printing a linked list in Java using Java Streams offers a concise and expressive way to iterate through the list and print its elements. Java Streams were introduced in Java 8 to facilitate functional-style programming, and they provide a declarative syntax for processing sequences of elements.
In this example, we’ll leverage Java Streams to print the linked list:
import java.util.*;
public class PrintLinkedListWithStreams {
public static void main(String[] args) {
LinkedList<String> fruits = new LinkedList<>();
fruits.add("apple");
fruits.add("orange");
fruits.add("mango");
fruits.stream().forEach(e -> System.out.print(e + " "));
}
}
In this example, we create a linked list of strings named fruits
and add three elements to it. The stream()
method is applied to the linked list, followed by the forEach
terminal operation, which prints each element.
The main
method initializes the linked list and adds elements. The key part is the usage of Java Streams to process the linked list.
The stream()
method converts the linked list into a stream of elements, and the forEach
method iterates over each element, applying the provided action, which is printing the element followed by a space. This concise syntax eliminates the need for explicit loops or recursion.
Java Streams provide a more functional and expressive way to handle collections, and they can be particularly useful when combined with lambda expressions for succinct operations.
After executing the provided code, the output will be:
apple orange mango
The linked list is printed with elements separated by spaces, showcasing the use of Java Streams for a streamlined approach to printing a linked list in Java.
Print a Linked List in Java Using StringBuilder
Printing a linked list in Java using a StringBuilder
for concatenation is a memory-efficient approach, especially when dealing with a large number of elements. StringBuilder
allows for efficient string manipulation without the overhead of creating multiple string objects.
In the example below, we’ll demonstrate how to use StringBuilder
to concatenate the elements of a linked list and print the result:
import java.util.*;
public class PrintLinkedListWithStringBuilder {
public static void main(String[] args) {
LinkedList<String> fruits = new LinkedList<>();
fruits.add("apple");
fruits.add("orange");
fruits.add("mango");
StringBuilder result = new StringBuilder();
for (String fruit : fruits) {
result.append(fruit).append(" ");
}
System.out.print(result.toString());
}
}
In this example, we create a linked list of strings named fruits
and add three elements to it. A StringBuilder
named result
is used to concatenate the elements of the linked list, and the final result is printed.
The main
method starts by initializing the linked list and adding elements. The key component here is the use of a StringBuilder
named result
.
We iterate through the elements of the linked list using an enhanced for
loop, and for each element, we append it to the StringBuilder
along with a space. This process efficiently builds a string representation of the linked list.
Using StringBuilder
for concatenation is more efficient than directly concatenating strings, especially when dealing with a large number of elements. It reduces the overhead of creating multiple string objects and results in better performance.
After executing the provided code, the output will be:
apple orange mango
The linked list is printed with elements separated by spaces, showcasing the use of StringBuilder
for efficient string concatenation when printing a linked list in Java.
Print a Linked List in Java Using an Iterator
Printing a linked list in Java using an iterator provides a flexible and efficient way to traverse and access elements. The Iterator
interface allows us to iterate through a collection, such as a linked list, without exposing its underlying structure.
Here, we’ll demonstrate how to use an iterator to print the elements of a linked list:
import java.util.*;
public class PrintLinkedListWithIterator {
public static void main(String[] args) {
LinkedList<String> fruits = new LinkedList<>();
fruits.add("apple");
fruits.add("orange");
fruits.add("mango");
Iterator<String> iterator = fruits.iterator();
while (iterator.hasNext()) {
System.out.print(iterator.next() + " ");
}
}
}
In this example, an iterator is used to traverse the linked list fruits
, and the hasNext()
and next()
methods are employed to access and print each element.
The main
method initializes the linked list and adds elements. The crucial part of this example is the use of the Iterator
interface.
We obtain an iterator for the linked list using the iterator()
method, and then, in a while
loop, we check if there are more elements (hasNext()
) and print each element (next()
).
Using an iterator abstracts the underlying details of the linked list’s structure, providing a clean and iterable interface for accessing elements. It is particularly useful when a more controlled traversal is needed.
After executing the provided code, the output will be:
apple orange mango
The linked list is printed with elements separated by spaces, showcasing the use of an iterator for efficient traversal and printing of a linked list in Java.
Conclusion
Printing a linked list in Java offers various methods, each with its advantages and use cases. The choice depends on specific requirements, preferences, and the level of control needed during the printing process.
Whether opting for a simple System.out.println()
or a more advanced recursive or stream-based approach, Java provides a versatile set of tools for efficiently working with linked lists.