Doubly Linked List in Java
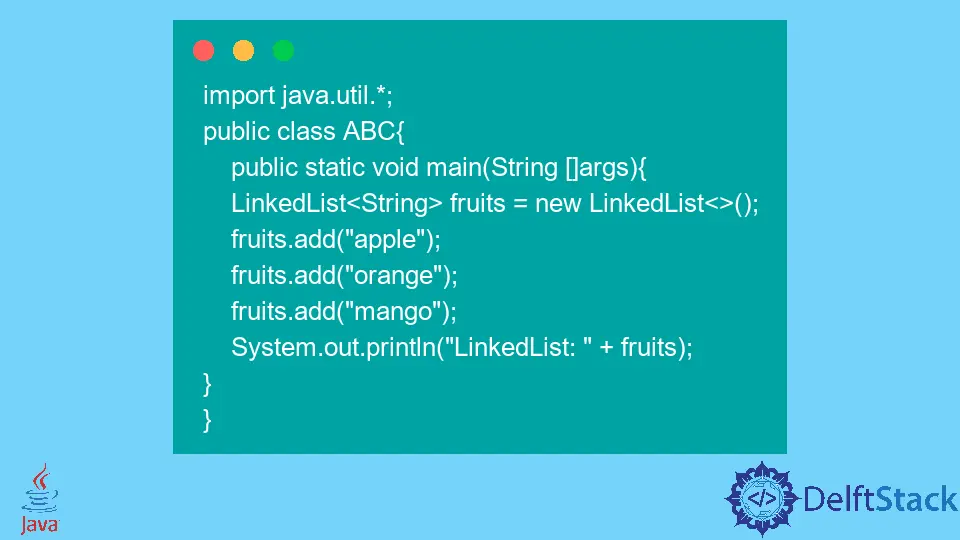
In a Linked List, every element acts as a separate object with a data part and an address part. It does not use a contagious memory location to store data. A Doubly Linked List stores the address for the previous node as well as the next node. Having two address nodes allows a Doubly Linked List to traverse in both directions.
This tutorial will discuss Linked Lists in Java.
In Java, the Linked List class is a part of Java’s Collections framework that provides the functionality of the Linked List data structure, which acts as a Doubly Linked List.
Each element here acts as a node that consists of 3 values, namely Prev
, Next
, and Data
, at a single position. Prev
stores address to the previous element, Next
stores address to next element, and Data
stores the actual data of the node.
For example,
import java.util.*;
public class ABC {
public static void main(String[] args) {
LinkedList<String> fruits = new LinkedList<>();
fruits.add("apple");
fruits.add("orange");
fruits.add("mango");
System.out.println("LinkedList: " + fruits);
}
}
Output:
LinkedList: [apple, orange, mango]
In the above example, we have successfully created a Linked List through Java’s Collections framework.
Here, the first node has data as apple
that also holds null value as its previous and address of orange as its following. Similarly, the second element with data orange
has the address of apple as previous and the address of mango
as next.
Whenever a new element is added, Prev
and Next
addresses are automatically updated internally.
The get()
method is used to access the elements from Linked List, which iterates from beginning to the element. We also have the listIterator()
method to access the components. The next()
and previous()
functions can help in traversing through the list in both directions.
Further, we can use the set()
method to change the Linked List elements. The remove()
function can delete an element.
We use some of these functions in the following example.
import java.util.*;
public class ABC {
public static void main(String[] args) {
LinkedList<String> fruits = new LinkedList<>();
fruits.add("apple");
fruits.add("orange");
fruits.add("mango");
String str = fruits.listIterator(1).previous();
System.out.println("i like " + str);
}
}
Output:
i like apple
In the above example, we accessed the value previous to the element at index 1 using the previous()
function.
We can also create our class with data and pointer variables to simulate a Doubly Linked list in Java.