How to Remove a Node From a Linked List in Java
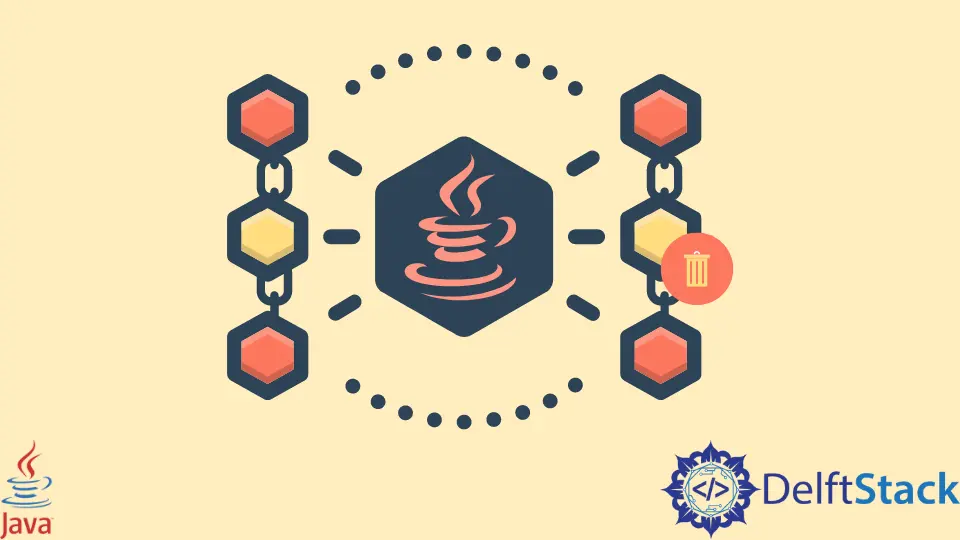
This tutorial demonstrates how to remove a node from a linked list in Java.
Remove a Node From a Linked List in Java
The linked list is a type of data structure from the util
package of Java which implements the Linked List data structure. The linked list is considered a linear data structure wherein each element is a separate object with an address and data part, and no element is stored in the contagious location.
Deleting a node from a linked list can be done by following the process where we will delete the first occurrence of the given key.
Here are the steps to delete a node from a linked list:
-
First, we must find a previous node of the given node. This means finding the node before the node is deleted.
-
The next step is to change the text of the previous node.
-
Finally, we need to free the memory for the deletable node.
This is an iterative process where every node is dynamically allocated using the malloc()
method of C, so we need to call the free()
method to free the memory every time.
Let’s try an example to delete a node from the given Linked list in Java:
package delftstack;
public class Example {
class DemoNode {
int NodeData;
DemoNode NextNode;
public DemoNode(int NodeData) {
this.NodeData = NodeData;
this.NextNode = null;
}
}
// head and tail node
public DemoNode HeadNode = null;
public DemoNode TailNode = null;
// to add new nodes
public void Add_Node(int NodeData) {
DemoNode NewNode = new DemoNode(NodeData);
if (HeadNode == null) {
HeadNode = NewNode;
TailNode = NewNode;
} else {
TailNode.NextNode = NewNode;
TailNode = NewNode;
}
}
// To delete nodes
public void DeleteNode() {
if (HeadNode == null) {
System.out.println("List is empty");
return;
} else {
// Checks if the list contains only one element
if (HeadNode != TailNode) {
DemoNode current = HeadNode;
// Iterate through the list till the second last element
while (current.NextNode != TailNode) {
current = current.NextNode;
}
TailNode = current;
TailNode.NextNode = null;
} else {
HeadNode = TailNode = null;
}
}
}
// To display the node
public void DisplayNode() {
DemoNode CurrentNode = HeadNode;
if (HeadNode == null) {
System.out.println("List is empty");
return;
}
while (CurrentNode != null) {
System.out.print(CurrentNode.NodeData + " ");
CurrentNode = CurrentNode.NextNode;
}
System.out.println();
}
public static void main(String[] args) {
Example Linked_List = new Example();
// Adds nodes to the list
Linked_List.Add_Node(1);
Linked_List.Add_Node(2);
Linked_List.Add_Node(3);
Linked_List.Add_Node(4);
System.out.println("The Original List is: ");
Linked_List.DisplayNode();
while (Linked_List.HeadNode != null) {
Linked_List.DeleteNode();
System.out.println("The list after Deleting a Node: ");
Linked_List.DisplayNode();
}
}
}
The code above will first add the nodes to the linked list and then delete the node from the end of the list. See the output:
The Original List is:
1 2 3 4
The list after Deleting a Node:
1 2 3
The list after Deleting a Node:
1 2
The list after Deleting a Node:
1
The list after Deleting a Node:
List is empty
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook