How to Convert String to Datetime in C#
-
C# Program to Convert
string
IntoDateTime
UsingConvert.ToDateTime()
-
C# Program to Convert
string
IntoDateTime
UsingDateTime.Parse()
-
C# Program to Convert
string
Into anDateTime
UsingDateTime.ParseExact()
- Conclusion
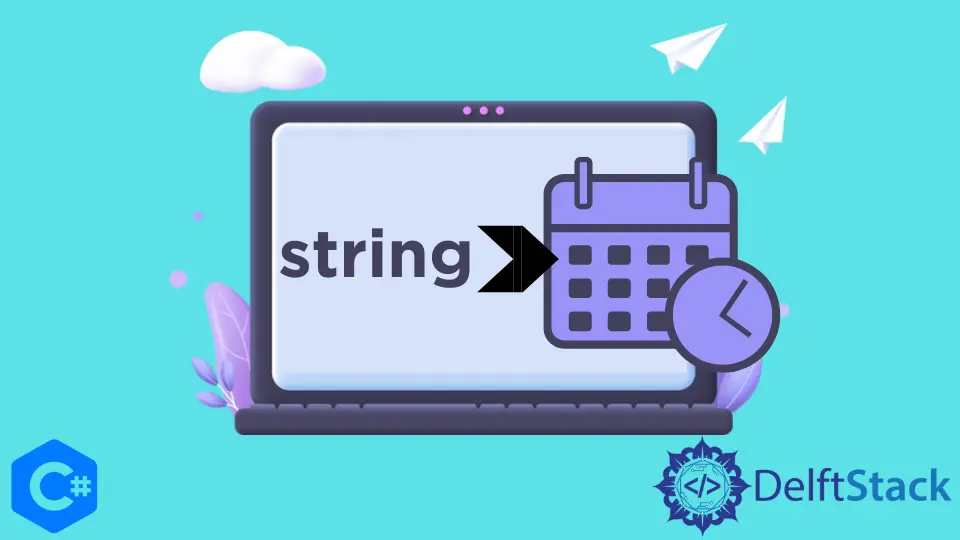
In most cases, we get a date in the form of a string and we want to use day, month and year individually. Not to worry, in C#, in order to convert a string to a DateTime
object, we use a pre-defined class named DateTime
.
There are several methods to convert string into DateTime
in C#, but here, we’ll only elaborate three methods with running examples. These methods are Convert.ToDateTime()
, DateTime.Parse()
and DateTime.ParseExact()
.
C# Program to Convert string
Into DateTime
Using Convert.ToDateTime()
The correct syntax of Convert.ToDateTime()
is
Convert.ToDateTime(dateTobeConverted);
Convert.ToDateTime(dateTobeConverted, cultureInfo);
It should be noted here that if you are not providing culture information then, by default, the compiler will see our date string as month/day/year
. CultureInfo
is a C# class in the System.Globalization
namespace that gives information about a specific culture.
using System;
public class Conversion {
public static void Main() {
string CurrentDate = "06/04/2020";
// Use of Convert.ToDateTime()
DateTime DateObject = Convert.ToDateTime(CurrentDate);
Console.WriteLine("The Date is: " + DateObject.Day + " " + DateObject.Month + " " +
DateObject.Year);
}
}
Output:
The Date is: 4 6 2020
Now we will implement it by passing the CultureInfo
object as a parameter.
using System;
using System.Globalization;
public class Conversion {
public static void Main() {
string CurrentDate = "06/04/2020";
// Creating new CultureInfo Object
// You can use different cultures like French, Spanish etc.
CultureInfo Culture = new CultureInfo("en-US");
// Use of Convert.ToDateTime()
DateTime DateObject = Convert.ToDateTime(CurrentDate, Culture);
Console.WriteLine("The Date is: " + DateObject.Day + " " + DateObject.Month + " " +
DateObject.Year);
}
}
Output:
The Date is: 4 6 2020
If we change the CultureInfo
to nl-NL
, the month and the day will be swapped.
using System;
using System.Globalization;
public class Conversion {
public static void Main() {
string CurrentDate = "06/04/2020";
CultureInfo Culture = new CultureInfo("nl-nl");
DateTime DateObject = Convert.ToDateTime(CurrentDate, Culture);
Console.WriteLine("The Date is: " + DateObject.Day + " " + DateObject.Month + " " +
DateObject.Year);
}
}
Output:
The Date is: 6 4 2020
C# Program to Convert string
Into DateTime
Using DateTime.Parse()
The syntax of DateTime.Parse()
is,
DateTime.Parse(dateTobeConverted);
DateTime.Parse(dateTobeConverted, cultureInfo);
The same is the case with DateTime.Parse()
method, if we are not providing culture information as an argument, then by default our system will see it as month/day/year
.
If the value of the string to be converted is null
, then it will return ArgumentNullException
which should be handled using try-catch
block.
using System;
public class Conversion {
public static void Main() {
string CurrentDate = "06/04/2020";
// Use of DateTime.Parse()
DateTime DateObject = DateTime.Parse(CurrentDate);
Console.WriteLine("The Date is: " + DateObject.Day + " " + DateObject.Month + " " +
DateObject.Year);
}
}
Output:
The Date is: 4 6 2020
C# Program to Convert string
Into an DateTime
Using DateTime.ParseExact()
The syntax of DateTime.ParseExact()
is,
DateTime.ParseExact(dateTobeConverted, dateFormat, cultureInfo);
DateTime.ParseExact()
is the best method to convert a string to DateTime
. In this method, we pass the format of the date as an argument. This makes it easy for the user to exactly perform the conversion.
Here we have passed null as a parameter in place of culture info because its a whole new topic and it takes time to understand that.
using System;
public class Conversion {
public static void Main() {
string CurrentDate = "06/04/2020";
// Use of DateTime.ParseExact()
// culture information is null here
DateTime DateObject = DateTime.ParseExact(CurrentDate, "dd/MM/yyyy", null);
Console.WriteLine("The Date is: " + DateObject.Day + " " + DateObject.Month + " " +
DateObject.Year);
}
}
Output:
The Date is: 6 4 2020
Conclusion
There are many methods to convert a string to DateTime
in C#. We have discussed a few of them. The best method to convert a string to DateTime
is DateTime.ParseExact()
.
Related Article - Csharp String
- How to Convert String to Enum in C#
- How to Convert Int to String in C#
- How to Use Strings in Switch Statement in C#
- How to Convert a String to Boolean in C#
- How to Convert a String to Float in C#
- How to Convert a String to a Byte Array in C#