C# で文字列を日時に変換
-
Convert.ToDateTime()
を使用してstring
をDateTime
に変換する C# プログラム -
DateTime.Parse()
を使用してstring
をDateTime
に変換する C# プログラム -
DateTime.ParseExact()
を使用してstring
をDateTime
に変換する C# プログラム - まとめ
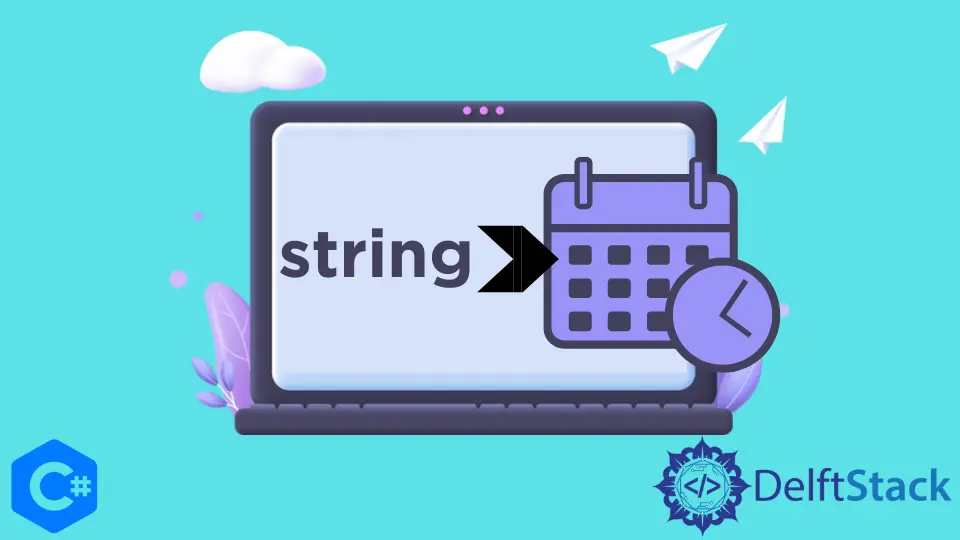
ほとんどの場合、文字列の形式で日付を取得し、日、月、年を個別に使用します。心配する必要はありません。C# では、文字列を DateTime
オブジェクトに変換するために、DateTime
という名前の事前定義されたクラスを使用します。
C# で文字列を DateTime
に変換する方法はいくつかありますが、ここでは、実行例を使用して 3つのメソッドのみを詳しく説明します。これらのメソッドは、Convert.ToDateTime()
、DateTime.Parse()
、および DateTime.ParseExact()
です。
Convert.ToDateTime()
を使用して string
を DateTime
に変換する C# プログラム
Convert.ToDateTime()
の正しい構文は次のとおりです
Convert.ToDateTime(dateTobeConverted);
Convert.ToDateTime(dateTobeConverted, cultureInfo);
ここで、カルチャ情報(CultureInfo
)を提供しない場合、コンパイラーはデフォルトで日付文字列を月/日/年として表示します。ことに注意してください。CultureInfo
は、System.Globalization
名前空間の C# クラスで、特定の文化に関する情報。
using System;
public class Conversion {
public static void Main() {
string CurrentDate = "06/04/2020";
// Use of Convert.ToDateTime()
DateTime DateObject = Convert.ToDateTime(CurrentDate);
Console.WriteLine("The Date is: " + DateObject.Day + " " + DateObject.Month + " " +
DateObject.Year);
}
}
出力:
The Date is: 4 6 2020
次に、CultureInfo
オブジェクトをパラメーターとして渡して実装します。
using System;
using System.Globalization;
public class Conversion {
public static void Main() {
string CurrentDate = "06/04/2020";
// Creating new CultureInfo Object
// You can use different cultures like French, Spanish etc.
CultureInfo Culture = new CultureInfo("en-US");
// Use of Convert.ToDateTime()
DateTime DateObject = Convert.ToDateTime(CurrentDate, Culture);
Console.WriteLine("The Date is: " + DateObject.Day + " " + DateObject.Month + " " +
DateObject.Year);
}
}
出力:
The Date is: 4 6 2020
CultureInfo
を nl-NL
に変更すると、月と日が入れ替わります。
using System;
using System.Globalization;
public class Conversion {
public static void Main() {
string CurrentDate = "06/04/2020";
CultureInfo Culture = new CultureInfo("nl-nl");
DateTime DateObject = Convert.ToDateTime(CurrentDate, Culture);
Console.WriteLine("The Date is: " + DateObject.Day + " " + DateObject.Month + " " +
DateObject.Year);
}
}
出力:
The Date is: 6 4 2020
DateTime.Parse()
を使用して string
を DateTime
に変換する C# プログラム
DateTime.Parse()
の構文は、
DateTime.Parse(dateTobeConverted);
DateTime.Parse(dateTobeConverted, cultureInfo);
DateTime.Parse()
メソッドの場合も同じです。引数としてカルチャ情報を提供していない場合、デフォルトではシステムは月/日/年として表示します。
変換される文字列の値が null
の場合、ArgumentNullException
が返されます。これは、try-catch
ブロックを使用して処理する必要があります。
using System;
public class Conversion {
public static void Main() {
string CurrentDate = "06/04/2020";
// Use of DateTime.Parse()
DateTime DateObject = DateTime.Parse(CurrentDate);
Console.WriteLine("The Date is: " + DateObject.Day + " " + DateObject.Month + " " +
DateObject.Year);
}
}
出力:
The Date is: 4 6 2020
DateTime.ParseExact()
を使用して string
を DateTime
に変換する C# プログラム
DateTime.ParseExact()
の構文は、
DateTime.ParseExact(dateTobeConverted, dateFormat, cultureInfo);
DateTime.ParseExact()
は文字列を DateTime
に変換する最良の方法です。このメソッドでは、日付の形式を引数として渡します。これにより、ユーザーが変換を正確に実行することが容易になります。
ここでは、文化情報の代わりに null をパラメーターとして渡しました。これは、まったく新しいトピックであり、それを理解するには時間がかかるためです。
using System;
public class Conversion {
public static void Main() {
string CurrentDate = "06/04/2020";
// Use of DateTime.ParseExact()
// culture information is null here
DateTime DateObject = DateTime.ParseExact(CurrentDate, "dd/MM/yyyy", null);
Console.WriteLine("The Date is: " + DateObject.Day + " " + DateObject.Month + " " +
DateObject.Year);
}
}
出力:
The Date is: 6 4 2020
まとめ
C# で文字列を DateTime
に変換する方法はたくさんあります。それらのいくつかについて説明しました。文字列を DateTime
に変換する最良の方法は DateTime.ParseExact()
です。
関連記事 - Csharp String
- C# 文字列を列挙型に変換
- C# 整数を文字列に変換
- C# の switch 文で文字列を使用する
- C# で文字列をブール値に変換する方法
- C# で文字列をバイト配列に変換する方法
- C# で文字列をフロートに変換する方法