C# での Datetime のフォーマット
Fil Zjazel Romaeus Villegas
2024年2月16日
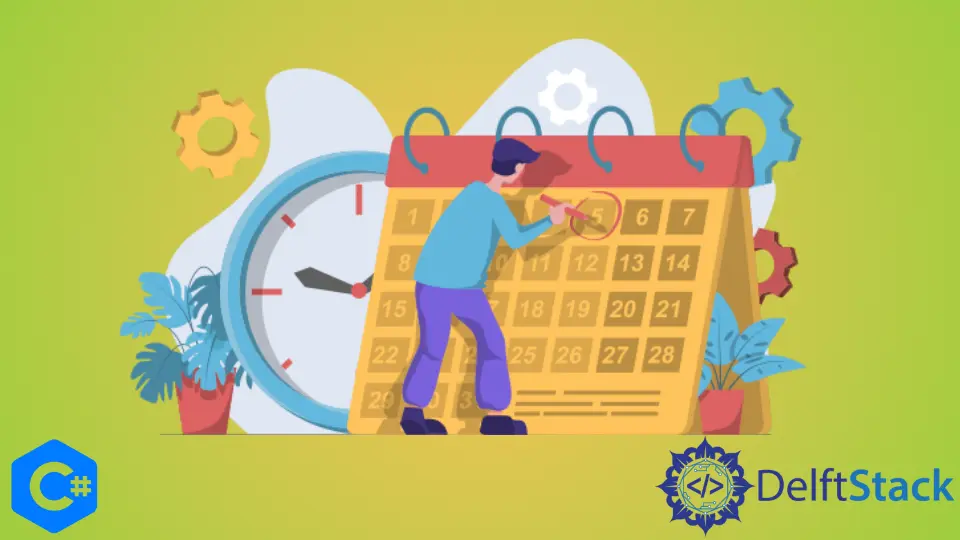
このチュートリアルでは、ToString()
または String.Format
関数を使用して、DateTime
変数からフォーマットされた文字列を作成する方法を示します。
日付形式指定子は、DateTime
変数内のさまざまなコンポーネントの文字列表現です。これらを ToString()
関数と String.Format
関数の両方で使用して、独自のカスタム DateTime
形式を指定できます。
一般的に使用される日付形式の指定子を次に示します。
サンプル日付:08/09/2021 3:25:10 PM
カスタム日時形式指定子:
フォーマット指定子 | 出力 | 日時パート |
---|---|---|
d |
8 |
日 |
dd |
08 |
日 |
M |
9 |
月 |
MM |
09 |
月 |
MMM |
Sep |
月 |
MMMM |
September |
月 |
yy |
21 |
年 |
yyyy |
2021 |
年 |
hh |
03 |
時間 |
HH |
15 |
時間 |
mm |
25 |
分 |
ss |
10 |
セカンド |
tt |
PM |
ティム |
標準の日時形式指定子:
指定子 | DateTimeFormatInfo プロパティ | パターン値 |
---|---|---|
t |
ShortTimePattern |
h:mm tt |
d |
ShortDatePattern |
M/d/yyyy |
F |
FullDateTimePattern |
dddd, MMMM dd, yyyy h:mm:ss tt |
M |
MonthDayPattern |
MMMM dd |
DateTime
フォーマット指定子の詳細なリストと例については、以下の Microsoft の公式ドキュメントを確認してください。
ToString()
を使用した C# での DateTime のフォーマット
DateTime
を文字列にフォーマットするのは、ToString()
を DateTime
に適用し、使用したいフォーマットを指定するのと同じくらい簡単です。
DateTime.Now.ToString("ddMMyyyy") DateTime.Now.ToString("d")
例:
using System;
namespace ConsoleApp1 {
class Program {
static void Main(string[] args) {
// Initialize the datetime value
DateTime date = new DateTime(2021, 12, 18, 16, 5, 7, 123);
// Format the datetime value to a standard format (ShortDatePattern)
string stanadard_fmt_date = date.ToString("d");
// Format the datetime value to a custom format
string custom_fmt_date = date.ToString("ddMMyyyy");
// Print the datetime formatted in the different ways
Console.WriteLine("Plain DateTime: " + date.ToString());
Console.WriteLine("Standard DateTime Format: " + stanadard_fmt_date);
Console.WriteLine("Custom DateTime Format: " + custom_fmt_date);
}
}
}
出力:
Plain DateTime: 18/12/2021 4:05:07 pm
Standard DateTime Format: 18/12/2021
Custom DateTime Format: 18122021
String.Format()
を使用した C# での DateTime のフォーマット
String.Format
メソッドを使用して DateTime
を文字列にフォーマットするには、パラメータにフォーマットと DateTime
値の両方を指定します。フォーマットを出力するときは、フォーマットを角かっこで囲み、接頭辞"0:"
を付ける必要があります。
String.Format("{0:ddMMyyyy}", DateTime.Now) String.Format("{0:d}", DateTime.Now)
例:
using System;
namespace ConsoleApp1 {
class Program {
static void Main(string[] args) {
// Initialize the datetime value
DateTime date = new DateTime(2021, 12, 18, 16, 5, 7, 123);
// Format the datetime value to a standard format (ShortDatePattern)
string stanadard_fmt_date = String.Format("{0:d}", date);
// Format the datetime value to a custom format
string custom_fmt_date = String.Format("{0:ddMMyyyy}", date);
// Print the datetime formatted in the different ways
Console.WriteLine("Plain DateTime: " + date.ToString());
Console.WriteLine("Standard DateTime Format: " + stanadard_fmt_date);
Console.WriteLine("Custom DateTime Format: " + custom_fmt_date);
}
}
}
出力:
Plain DateTime: 18/12/2021 4:05:07 pm
Standard DateTime Format: 18/12/2021
Custom DateTime Format: 18122021