Datetime formatieren in C#
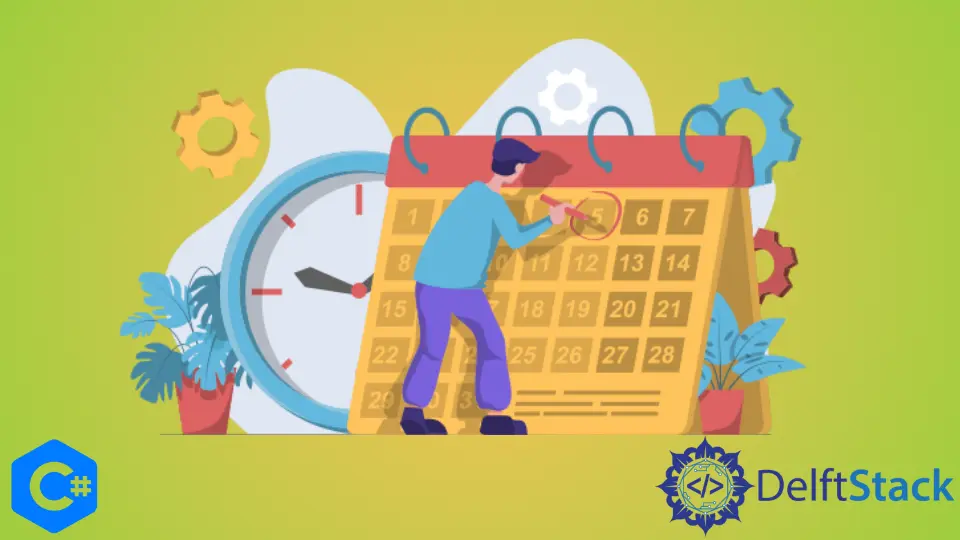
Dieses Tutorial zeigt, wie man einen formatierten String aus einer DateTime
-Variablen mit der ToString()
- oder String.Format
-Funktion erstellt.
Datumsformatspezifizierer sind die Zeichenkettendarstellung der verschiedenen Komponenten in einer DateTime
-Variablen. Sie können sie sowohl in den Funktionen ToString()
als auch String.Format
verwenden, um Ihr eigenes benutzerdefiniertes DateTime
-Format anzugeben.
Hier sind einige häufig verwendete Datumsformatbezeichner.
Beispieldatum: 08/09/2021 3:25:10 PM
Benutzerdefinierte DateTime-Formatspezifizierer:
Formatbezeichner | Ausgabe | DateTime-Teil |
---|---|---|
d |
8 |
Tag |
dd |
08 |
Tag |
M |
9 |
Monat |
MM |
09 |
Monat |
MMM |
Sep |
Monat |
MMMM |
September |
Monat |
yy |
21 |
Jahr |
yyyy |
2021 |
Jahr |
hh |
03 |
Stunde |
HH |
15 |
Stunde |
mm |
25 |
Minute |
ss |
10 |
Sekunde |
tt |
PM |
Uhrzeit |
Standard-DateTime-Formatbezeichner:
Spezifizierer | DateTimeFormatInfo-Eigenschaft | Musterwert |
---|---|---|
t |
ShortTimePattern |
h:mm tt |
d |
ShortDatePattern |
M/d/yyyy |
F |
FullDateTimePattern |
dddd, MMMM dd, yyyy h:mm:ss tt |
M |
MonthDayPattern |
MMMM dd |
Eine detailliertere Liste und weitere Beispiele für DateTime
-Formatbezeichner finden Sie in der offiziellen Dokumentation von Microsoft unten:
Formatieren von DateTime in C# mit ToString()
Das Formatieren einer DateTime
als Zeichenfolge ist so einfach wie das Anwenden von ToString()
auf die DateTime
und das Bereitstellen des Formats, das Sie verwenden möchten.
DateTime.Now.ToString("ddMMyyyy") DateTime.Now.ToString("d")
Beispiel:
using System;
namespace ConsoleApp1 {
class Program {
static void Main(string[] args) {
// Initialize the datetime value
DateTime date = new DateTime(2021, 12, 18, 16, 5, 7, 123);
// Format the datetime value to a standard format (ShortDatePattern)
string stanadard_fmt_date = date.ToString("d");
// Format the datetime value to a custom format
string custom_fmt_date = date.ToString("ddMMyyyy");
// Print the datetime formatted in the different ways
Console.WriteLine("Plain DateTime: " + date.ToString());
Console.WriteLine("Standard DateTime Format: " + stanadard_fmt_date);
Console.WriteLine("Custom DateTime Format: " + custom_fmt_date);
}
}
}
Ausgabe:
Plain DateTime: 18/12/2021 4:05:07 pm
Standard DateTime Format: 18/12/2021
Custom DateTime Format: 18122021
Formatieren von DateTime in C# mit String.Format()
Die Formatierung einer DateTime
in eine Zeichenfolge mit der String.Format
-Methode erfolgt durch die Bereitstellung sowohl des Formats als auch des DateTime
-Werts in den Parametern. Das Format muss beim Drucken des Formats in Klammern eingeschlossen und mit "0:"
vorangestellt werden.
String.Format("{0:ddMMyyyy}", DateTime.Now) String.Format("{0:d}", DateTime.Now)
Beispiel:
using System;
namespace ConsoleApp1 {
class Program {
static void Main(string[] args) {
// Initialize the datetime value
DateTime date = new DateTime(2021, 12, 18, 16, 5, 7, 123);
// Format the datetime value to a standard format (ShortDatePattern)
string stanadard_fmt_date = String.Format("{0:d}", date);
// Format the datetime value to a custom format
string custom_fmt_date = String.Format("{0:ddMMyyyy}", date);
// Print the datetime formatted in the different ways
Console.WriteLine("Plain DateTime: " + date.ToString());
Console.WriteLine("Standard DateTime Format: " + stanadard_fmt_date);
Console.WriteLine("Custom DateTime Format: " + custom_fmt_date);
}
}
}
Ausgabe:
Plain DateTime: 18/12/2021 4:05:07 pm
Standard DateTime Format: 18/12/2021
Custom DateTime Format: 18122021